generated from yediyuz/laravel-package
-
Notifications
You must be signed in to change notification settings - Fork 5
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
2 changed files
with
112 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,3 +1,6 @@ | ||
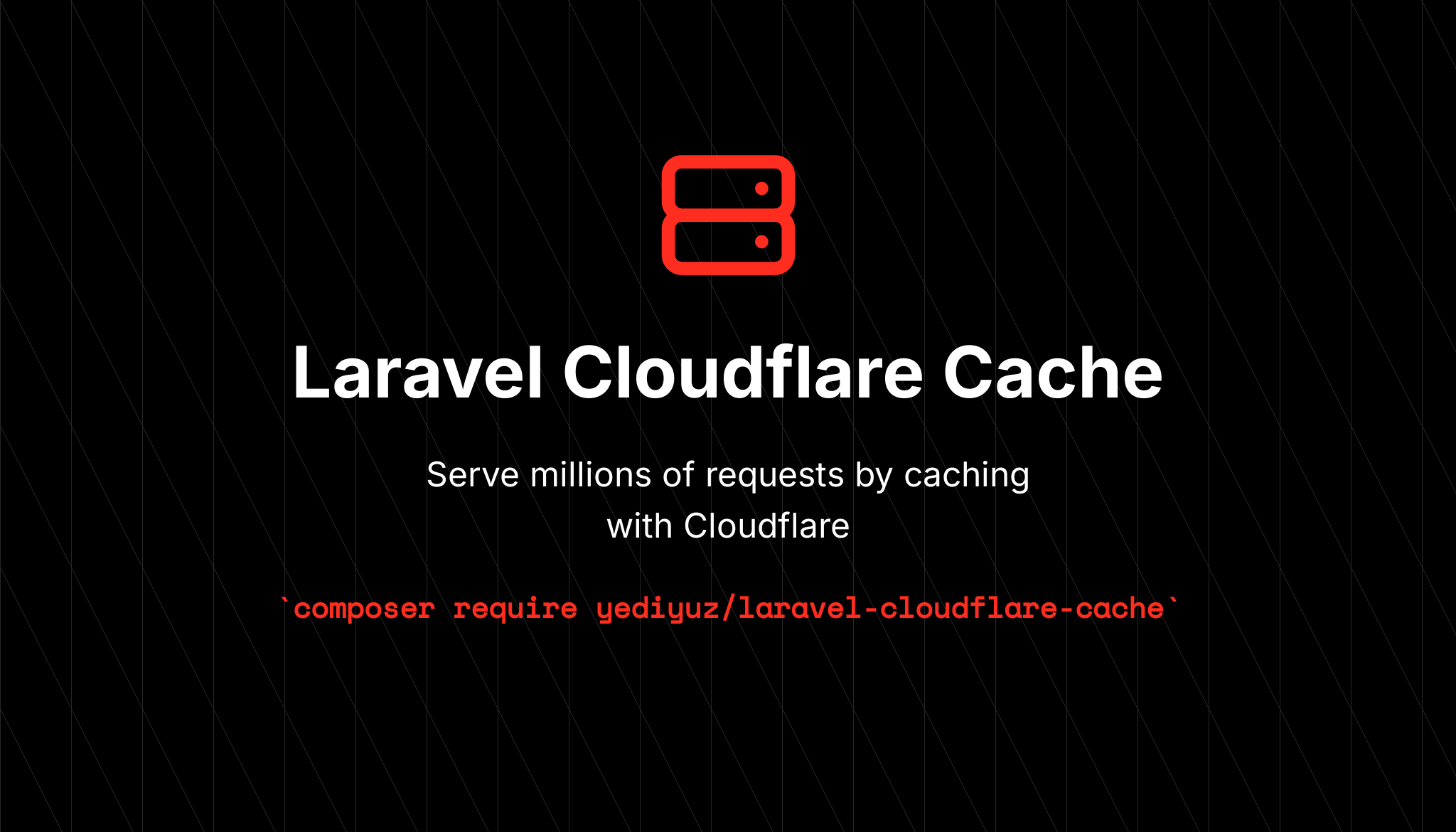 | ||
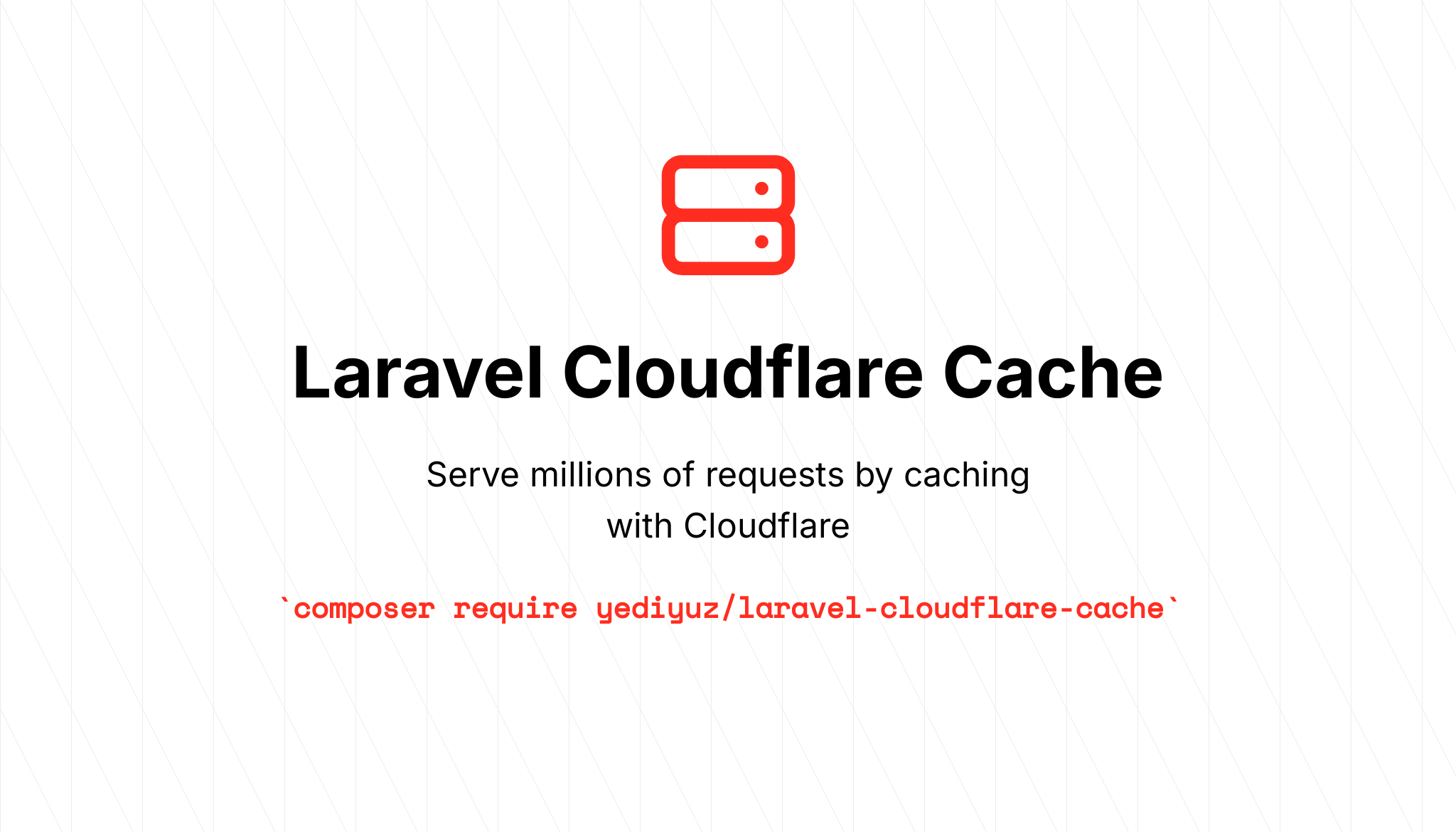 | ||
|
||
# Laravel Cloudflare Cache | ||
|
||
<p> | ||
|
@@ -14,6 +17,112 @@ You can install the package via composer: | |
composer require yediyuz/laravel-cloudflare-cache | ||
``` | ||
|
||
You can publish the config file with: | ||
|
||
```bash | ||
php artisan vendor:publish --tag="cloudflare-cache-config" | ||
``` | ||
|
||
Add environment variables to .env file | ||
```dotenv | ||
[email protected] #Cloudflare account email address | ||
CLOUDFLARE_CACHE_KEY=XXXXXXX #Cloudflare API_KEY | ||
CLOUDFLARE_CACHE_IDENTIFIER=XXXXXXX #ZONE_ID | ||
CLOUDFLARE_DEFAULT_CACHE_TTL=600 #10 minutes | ||
CLOUDFLARE_CACHE_DEBUG=false | ||
``` | ||
|
||
## Usage | ||
|
||
### Define routes to cache | ||
You can use cache groups for your static contents. | ||
```php | ||
Route::cache()->group(function () { | ||
Route::get('/content', function () { | ||
return 'content'; | ||
}); | ||
}); | ||
``` | ||
|
||
You can use cache tags, so you can clear your caches easily. Specify custom ttl for expire time in seconds. When you do not pass ttl, it will use default ttl given in the config. | ||
```php | ||
Route::cache(tags: ['tag1', 'tag2'], ttl: 600)->group(function () { | ||
Route::get('/content_with_tags', function () { | ||
return 'content'; | ||
}); | ||
}); | ||
|
||
Route::cache(tags: ['staticPages'])->group(function () { | ||
// | ||
}); | ||
``` | ||
### Clear Cache | ||
|
||
#### Purges everything | ||
https://developers.cloudflare.com/cache/how-to/purge-cache/purge-everything/ | ||
```php | ||
CloudflareCache::purgeEverything() | ||
``` | ||
|
||
#### Purges by urls | ||
https://developers.cloudflare.com/cache/how-to/purge-cache/purge-by-single-file/ | ||
```php | ||
CloudflareCache::purgeByUrls([ | ||
'https://example.com/hello', | ||
]) | ||
``` | ||
|
||
#### Purges by prefixes (Enterprise only) | ||
https://developers.cloudflare.com/cache/how-to/purge-cache/purge_by_prefix/ | ||
```php | ||
CloudflareCache::purgeByPrefixes([ | ||
'www.example.com/foo', | ||
]) | ||
``` | ||
|
||
#### Purges by tags (Enterprise only) | ||
https://developers.cloudflare.com/cache/how-to/purge-cache/purge-by-tags/ | ||
```php | ||
CloudflareCache::purgeByTags([ | ||
'staticPages', | ||
]) | ||
``` | ||
|
||
#### Purges by hostname (Enterprise only) | ||
https://developers.cloudflare.com/cache/how-to/purge-cache/purge-by-hostname/ | ||
```php | ||
CloudflareCache::purgeByHosts([ | ||
'www.example.com', | ||
'images.example.com', | ||
]) | ||
``` | ||
|
||
Post update example to clear cache | ||
```php | ||
<?php | ||
|
||
namespace App\Http\Controllers; | ||
|
||
use App\Http\Requests\UpdatePostRequest; | ||
use App\Models\Post; | ||
use Yediyuz\CloudflareCache\Facades\CloudflareCache; | ||
|
||
class PostController extends Controller | ||
{ | ||
public function update(Post $post, UpdatePostRequest $request) | ||
{ | ||
$post->update($request->validated()); | ||
|
||
CloudflareCache::purgeByUrls([ | ||
route('post.show', $post->id) | ||
]); | ||
|
||
return back()->with('message', 'Post updated and url cache purged'); | ||
} | ||
} | ||
``` | ||
|
||
|
||
## Testing | ||
|
||
```bash | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters