This repository has been archived by the owner on Aug 14, 2021. It is now read-only.
-
Notifications
You must be signed in to change notification settings - Fork 3
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat: adding documentation for runtime client (CORE-4955) (#24)
* wip: adding back old documentation * docs: removed xavi's integration due to using an outdated runtime client build * docs: updated main README * docs: reviewed up to makeTraceProcessor * docs: correcting docuemntation * docs: added server sample to main README * docs: fixing heading size Co-authored-by: Zhiheng Lu <[email protected]> Co-authored-by: Tyler Han <[email protected]>
- Loading branch information
1 parent
204ac48
commit 8e55a1a
Showing
4 changed files
with
974 additions
and
39 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,71 +1,127 @@ | ||
# @voiceflow/runtime-client-js | ||
# Voiceflow Runtime Client | ||
|
||
> javascript client SDK for running voiceflow projects anywhere | ||
The Voiceflow Runtime Client is an SDK for running Voiceflow apps in JavaScript. | ||
|
||
First, you build a fully-functioning conversational app on [Voiceflow](https://creator.voiceflow.com). Then, you integrate that app into a JavaScript project using the SDK. This allows you to quickly add any kind of voice interface, such as a chatbot, to your project, without the hassle of implementing the conversational flow using code. | ||
|
||
The Runtime Client can be used with jQuery, React, and any other JavaScript library or framework. | ||
|
||
[](https://circleci.com/gh/voiceflow/runtime-client-js/tree/master) | ||
[](https://codecov.io/gh/voiceflow/runtime-client-js) | ||
[](https://sonarcloud.io/dashboard?id=voiceflow_runtime-client-js) | ||
|
||
## Table of Contents | ||
|
||
1. [Demos](#demos) | ||
2. [Samples](#samples) | ||
3. [Install](#install) | ||
4. [Getting Started](#getting-started) | ||
5. [Advanced Usage](#advanced-usage) | ||
6. [Development](#api-reference) | ||
|
||
## Demos | ||
|
||
Web Demo https://voiceflow-burger.webflow.io/ | ||
- Voiceflow Burgers (Web) - [source](https://voiceflow-burger.webflow.io/) | ||
|
||
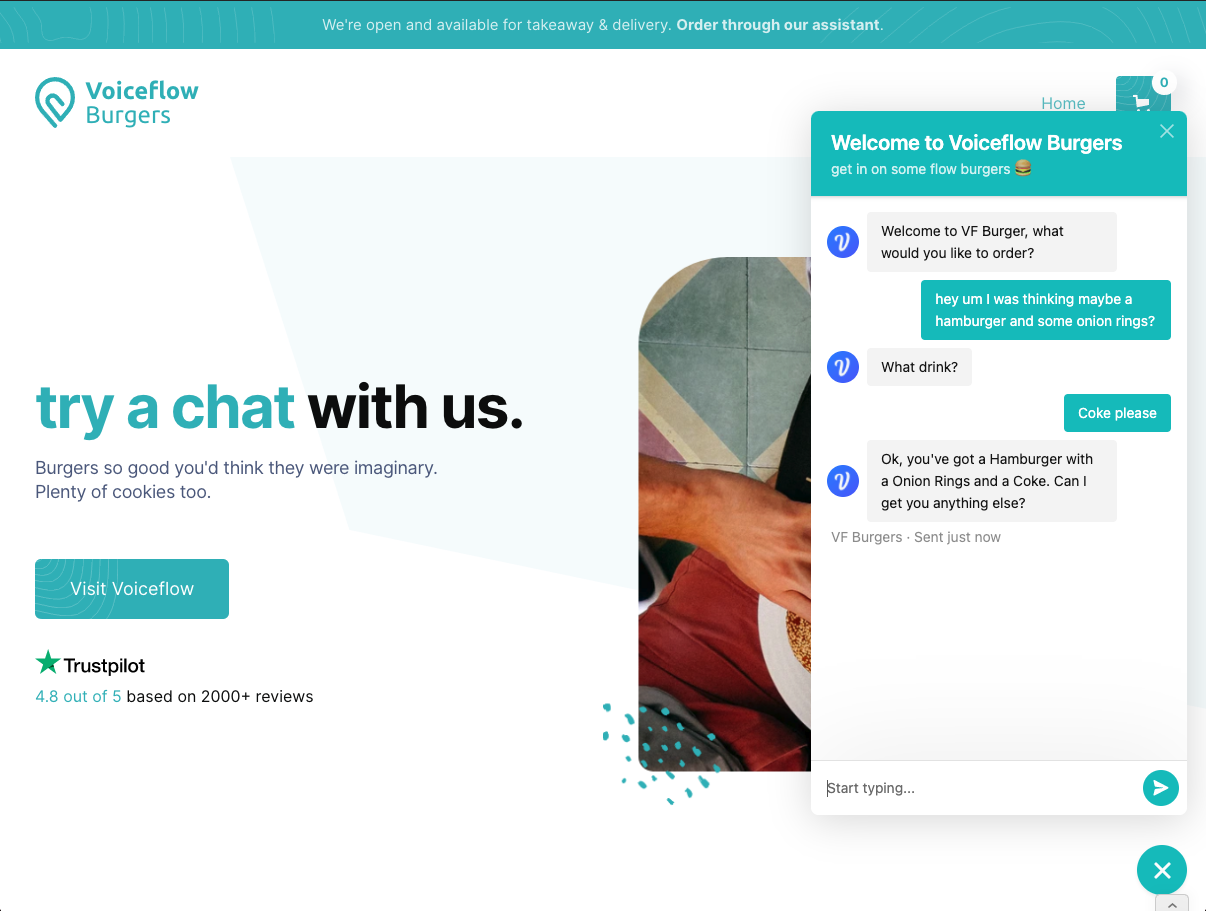 | ||
<img src="https://user-images.githubusercontent.com/5643574/106966841-17b9ee00-6714-11eb-868a-26751b7d560e.png" alt="demo" style="zoom:50%;" /> | ||
|
||
## Samples | ||
|
||
See the parent [rcjs-examples](https://github.com/voiceflow/rcjs-examples) repo for instructions on how to setup each Sample. | ||
|
||
- Hello World (Node.js) - [source](https://github.com/voiceflow/rcjs-examples/tree/master/hello-world) | ||
- Hamburger Order App (jQuery) - [source](https://github.com/voiceflow/rcjs-examples/tree/master/hamburger-order-jQuery) | ||
- Hamburger Order App (React) - [source](https://github.com/voiceflow/rcjs-examples/tree/master/hamburger-order-react) | ||
- Hamburger Order App (Node.js) - [source](https://github.com/voiceflow/rcjs-examples/tree/master/hamburger-order) | ||
- Hamburger Order Server (Express) - [source](https://github.com/voiceflow/rcjs-examples/tree/master/server) | ||
- Using Trace Processor (Node.js) - [source](https://github.com/voiceflow/rcjs-examples/tree/master/trace-processor) | ||
- Using TTS (React) - [source](https://github.com/voiceflow/rcjs-examples/tree/master/text-to-speech) | ||
- Using Suggestion Chips (React) - [source](https://github.com/voiceflow/rcjs-examples/tree/master/suggestion-chips) | ||
|
||
## Install | ||
|
||
``` | ||
```bash | ||
# with NPM | ||
npm install --save @voiceflow/runtime-client-js | ||
|
||
# with yarn | ||
yarn add @voiceflow/runtime-client-js | ||
``` | ||
|
||
## Basic Usage | ||
## Getting Started | ||
|
||
First build a project on [Voiceflow](https://creator.voiceflow.com). Make sure it works by testing it. | ||
### Minimal Working Integration | ||
|
||
Retrieve the `versionID` from the URL: | ||
`https://creator.voiceflow.com/project/{VERSION_ID}/...` and initialize the client | ||
The minimum code to start using the SDK is shown below: | ||
|
||
```javascript | ||
const VF = require('@voiceflow/runtime-client-js'); | ||
```js | ||
const { RuntimeClientFactory } = require('@voiceflow/runtime-client-js'); | ||
// alternatively for ESM/ES6 | ||
// import RuntimeClientFactory from '@voiceflow/runtime-client-js' | ||
|
||
const factory = new VF.RuntimeClientFactory({ | ||
versionID: 'XXXXXXXXXXXXXXXXXXXXXXXX', // voiceflow project versionID | ||
// Construct an object | ||
const factory = new RuntimeClientFactory({ | ||
versionID: 'your-version-id-here', // ADD YOUR VERSION ID HERE | ||
endpoint: 'https://general-runtime.voiceflow.com', | ||
}); | ||
|
||
const client = factory.createClient(); | ||
|
||
// (optional) start the conversation | ||
client.start().then((context) => { | ||
console.log(context.getResponse()); | ||
client.onSpeak((trace) => { | ||
// whenever we come across a speak block, just say the message | ||
console.log(trace); | ||
}); | ||
|
||
// Begin a conversation session | ||
client.start(); | ||
|
||
// call this function from any input source | ||
// e.g. interaction('can I have fries with that'); | ||
async function interaction(input) { | ||
// get a context for every user interaction | ||
const context = await client.sendText(input); | ||
|
||
// print out what the bot says back | ||
console.log(context.getResponse()); | ||
|
||
if (context.isEnding()) { | ||
console.log('conversation is over'); | ||
} | ||
} | ||
const interact = (input) => client.sendText(input); | ||
|
||
// e.g. interact('can I have fries with that'); | ||
``` | ||
|
||
Every interaction with the bot yields a conversation `context`. The `context` is a snapshot of the conversation at the current stage and contains useful information such as the bot's responses and the state of all the variables in the Voiceflow project, and much more! | ||
pass in user input with the `client.sendText(input)` function, and any of your `client.on...` functions will trigger during the response. | ||
|
||
### Setting up a Voiceflow App | ||
|
||
See [here](docs/setting-up-vf-app.md) for instructions on how to quickly setup a Voiceflow app to try out your project. | ||
|
||
### Integration Step-by-Step | ||
|
||
See [here](docs/step-by-step.md) for a step-by-step breakdown of the Minimal Working Integration. Make sure to read "Setting up a Voiceflow App" first. | ||
|
||
## Advanced Usage | ||
|
||
See the documentation [here](docs/advanced-usage.md) for the available advanced features of the SDK. | ||
|
||
## Development | ||
|
||
### Important Scripts | ||
|
||
#### `yarn install` | ||
|
||
Run `yarn install` to install any necessary dependencies to get started with working on the SDK. | ||
|
||
#### `yarn build` | ||
|
||
Use this to build the `runtime-client-js` locally. The build will be stored in the `/build` folder | ||
|
||
#### `yarn lint` | ||
|
||
Use this command to find any issues that fails our linter. It is important to have proper linting, otherwise your PR will not pass our automation. | ||
|
||
Use `yarn lint:fix` to check and automatically fix linting issues where possible. | ||
|
||
#### `yarn test` | ||
|
||
Use this command to run all of the integration and unit tests. Make sure that your PR achieves 100% coverage and tests for potential edge-cases. | ||
|
||
Use `yarn test:single` to execute a single unit or integration test. | ||
|
||
- To begin a conversation "session", the client code should invoke the `.start()` method, which returns a `context`. | ||
- For subsequent requests, the client code should invoke `.sendText()`, or `.sendRequest()` and pass in any appropriate data. Each method also returns a `context`. | ||
- `context.getResponses()` is the main conversation data. It is a list of `Trace` objects that represents the bot's response. | ||
- certain `Trace` types are filtered out by default, such as `block`, `debug`, `flow` traces. To access the entire trace, use `context.getTrace()` | ||
- `context.isEnding()` is a boolean that is true when the Voiceflow project is done. Make sure to check for this during each interaction. | ||
- `context.getChips()` returns an array of suggested responses that the user can say. This is generated based on what is configured in your Voiceflow project. | ||
Use `yarn test:unit` to run all of the unit tests | ||
|
||
## Runtime | ||
Use `yarn test:integration` to run all of the integration tests. | ||
|
||
As the name suggests, `runtime-client-js` interfaces with a Voiceflow "runtime" server. You can check out [https://github.com/voiceflow/general-runtime](https://github.com/voiceflow/general-runtime) and host your own runtime server. Modifying the runtime allows for extensive customization of bot behavior and integrations. | ||
### Submitting a PR | ||
|
||
By default, the client will use the Voiceflow hosted runtime at `https://general-runtime.voiceflow.com` | ||
We're always open to improving our Runtime Client SDK. Consider opening a PR if there is some improvement that you think should be added. Make sure to achieve 100% coverage for unit tests and provide documentation if applicable. |
Oops, something went wrong.