-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
0 parents
commit 6b35e9d
Showing
7 changed files
with
189 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,11 @@ | ||
.vscode | ||
PascalPons | ||
|
||
|
||
*.js | ||
*.html | ||
*.css | ||
|
||
LICENSE.txt | ||
|
||
*MARKS.txt |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,47 @@ | ||
# Introduction to AI Assignments | ||
|
||
Hey there! Welcome to my README for the assignments in the Computer Science 6980 Special Topics in Artificial Intelligence course. Below, I've provided comprehensive descriptions of each assignment along with links to their respective README files for demos. | ||
|
||
Special thanks to Professor Dave Churchill for his outstanding guidance and instruction throughout the course. | ||
|
||
## Assignments | ||
|
||
1. [BFS/DFS/IDDFS Grid-World Path-Finding](#assignment-1-bfsdfsiddfs-grid-world-path-finding) | ||
2. [A* Pathfinding](#assignment-2-a-pathfinding) | ||
|
||
--- | ||
|
||
## Assignment 1: BFS/DFS/IDDFS Grid-World Path-Finding | ||
|
||
### Description: | ||
|
||
For this assignment, I dived into the fundamentals of pathfinding algorithms, specifically Breadth-First Search (BFS), Depth-First Search (DFS), and Iterative Deepening Depth-First Search (IDDFS). It was quite exciting to implement these algorithms in the context of grid-world pathfinding scenarios. My task was to find optimal paths from a start point to a goal point in various grid configurations, and let me tell you, testing the efficiency and effectiveness of each algorithm was quite enlightening! | ||
|
||
### Instructions: | ||
|
||
- Implemented BFS, DFS, and IDDFS algorithms for grid-world pathfinding. | ||
- Tested the algorithms on different grid configurations and analyzed their performance. | ||
|
||
For detailed instructions and setup guidelines, you can check out the [Assignment 1 README](assignment-1/README.md). | ||
|
||
--- | ||
|
||
## Assignment 2: A* Pathfinding | ||
|
||
### Description: | ||
|
||
This assignment delved into the A* pathfinding algorithm, a widely-used method for finding the shortest path between nodes in a graph. I had the opportunity to implement the A* algorithm with support for both unidirectional and bidirectional search modes. Additionally, incorporating multiple heuristic options such as 8-directional Manhattan, 4-directional Manhattan, and 2D Euclidean distance truly enhanced the pathfinding accuracy and efficiency. | ||
|
||
### Instructions: | ||
|
||
- Implemented the A* pathfinding algorithm with unidirectional and bidirectional search modes. | ||
- Integrated various heuristic options to evaluate path costs and guide the search process. | ||
- Conducted thorough testing and performance analysis to assess the algorithm's effectiveness in different scenarios. | ||
|
||
For comprehensive instructions and setup details, refer to the [Assignment 2 README](assignment-2/README.md). | ||
|
||
--- | ||
|
||
These assignments provided me with invaluable insights into essential AI algorithms and techniques, significantly enhancing my proficiency in artificial intelligence concepts and applications. | ||
|
||
GPT used for the creating of this file |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,99 @@ | ||
## 1. Class Structure | ||
|
||
The algorithm is encapsulated within the `Search_Student` class, designed to handle the search process. It contains essential properties and methods for initializing, starting, and iterating through the search. | ||
|
||
## 2. Initialization | ||
|
||
- **Constructor:** | ||
- Initializes the search configuration, grid, start and goal positions, cost, and other necessary variables. | ||
|
||
## 3. Start Search | ||
|
||
- **startSearch(sx, sy, gx, gy):** | ||
- Initiates the search process by setting the start and goal positions. | ||
- Resets open and closed lists and creates the root node representing the start state. | ||
- Marks the search as in progress. | ||
|
||
## 4. Search Iteration | ||
|
||
- **searchIteration():** | ||
- Performs one iteration of the search process. | ||
- Expands nodes based on the selected search strategy (BFS or DFS). | ||
- Checks for the goal state, updates path, and sets the cost if the goal is found. | ||
- Manages open and closed lists to keep track of visited nodes. | ||
|
||
## 5. Node Expansion | ||
|
||
- **expand(node):** | ||
- Expands a given node by generating child nodes based on legal actions. | ||
- Child nodes are added to the open list for future exploration. | ||
|
||
## 6. Iterative Deepening Depth-First Search (IDDFS) | ||
|
||
- **searchIterationIDDFS():** | ||
- Handles search iterations specifically for Iterative Deepening Depth-First Search. | ||
- Increments the search depth with each iteration. | ||
- Expands nodes only if their depth is within the current depth limit. | ||
- Manages cutoff conditions when the depth limit is reached. | ||
|
||
## 7. Legal Actions and State Checking | ||
|
||
- **isLegalAction(x, y, action):** | ||
- Checks if a given action is legal from a specified position on the grid. | ||
- Ensures that the new state is within the grid boundaries and maintains consistency with the grid values. | ||
|
||
## 8. Result and Path Construction | ||
|
||
- **foundGoal(node):** | ||
- Constructs the path when the goal state is found. | ||
- Sets the cost based on the length of the path. | ||
|
||
- **didNotFindGoal():** | ||
- Handles situations where the goal state is not found. | ||
- Marks the search as not in progress, resets variables, and sets the cost to -1. | ||
|
||
## 9. CloseList Optimization | ||
|
||
- **CloseList class:** | ||
- Implements a 2D array for optimizing the tracking of visited points. | ||
- Saves and looks up points efficiently. | ||
- Allows resetting and retrieving all points. | ||
|
||
## 10. Usage and Configuration | ||
|
||
- **Usage section in README:** | ||
- Provides instructions on how to use the algorithm. | ||
- Explains the configuration options, including legal actions, action costs, and search strategy. | ||
|
||
## 11. Media Section | ||
|
||
### Breadth-First Search (BFS) | ||
|
||
#### Image: BFS Visualization | ||
 | ||
|
||
#### Video: BFS Algorithm in Action | ||
[](https://youtu.be/RkOh7328P44) | ||
|
||
|
||
|
||
### Depth-First Search (DFS) | ||
|
||
#### Image: DFS Visualization | ||
 | ||
|
||
#### Video: DFS Algorithm in Action | ||
[](https://youtu.be/a4rH3vMH-LA) | ||
|
||
|
||
### Iterative Deepening Depth-First Search (ID-DFS) | ||
|
||
#### Image: ID-DFS Visualization | ||
 | ||
|
||
#### Video: ID-DFS Algorithm in Action | ||
[](https://youtu.be/GvyiwZuNRco) | ||
|
||
|
||
These main ideas collectively form a basic framework for a grid-based search algorithm that can be applied to various problems. The specific search strategy (BFS, DFS, IDDFS) and the grid-related details are crucial aspects of the algorithm's functionality. | ||
|
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,32 @@ | ||
# COMP6980 - Assignment 2 | ||
|
||
## Overview | ||
This code implements the A* algorithm for pathfinding, with support for both unidirectional and bidirectional search modes. It includes multiple heuristic options such as 8-directional Manhattan, 4-directional Manhattan, and 2D Euclidean distance. | ||
|
||
## Implementation | ||
The implementation consists of a class `Search_Student` which represents the search algorithm. Here's a brief overview of important methods: | ||
|
||
- **canFit(x, y, size)**: Computes whether a given size agent can fit at the specified (x, y) location. | ||
- **computeSectors()**: Computes sectors using 4D BFS algorithm. | ||
- **isConnected(x1, y1, x2, y2, size)**: Determines whether two locations are connected. | ||
- **isLegalAction(x, y, action)**: Computes whether the given action can be performed from the given position. | ||
- **estimateCost(x, y, gx, gy)**: Computes the heuristic function h(n) from start location to goal location. | ||
- **startSearch(sx, sy, gx, gy, size)**: Initiates the search algorithm. | ||
- **searchIteration()**: Performs a single iteration of the search algorithm. | ||
- **getOpen()**: Returns the current open list states. | ||
- **getClosed()**: Returns the current closed list states. | ||
|
||
## Unidirectional vs Bidirectional Search | ||
- **Unidirectional Search**: Searches from the start node towards the goal node. | ||
- **Bidirectional Search**: Searches from both the start and goal nodes simultaneously, meeting at some midpoint. | ||
|
||
## Heuristic Options | ||
- **8-directional Manhattan**: Considers all 8 possible movement directions. | ||
- **4-directional Manhattan**: Considers only the 4 cardinal directions. | ||
- **2D Euclidean Distance**: Computes straight-line distance between two points. | ||
|
||
## Admissibility of Heuristics | ||
- **8-directional Search with 4-directional Heuristic**: Not admissible because it underestimates the true cost, leading to suboptimal paths. | ||
|
||
#### Video: Searches with different options | ||
[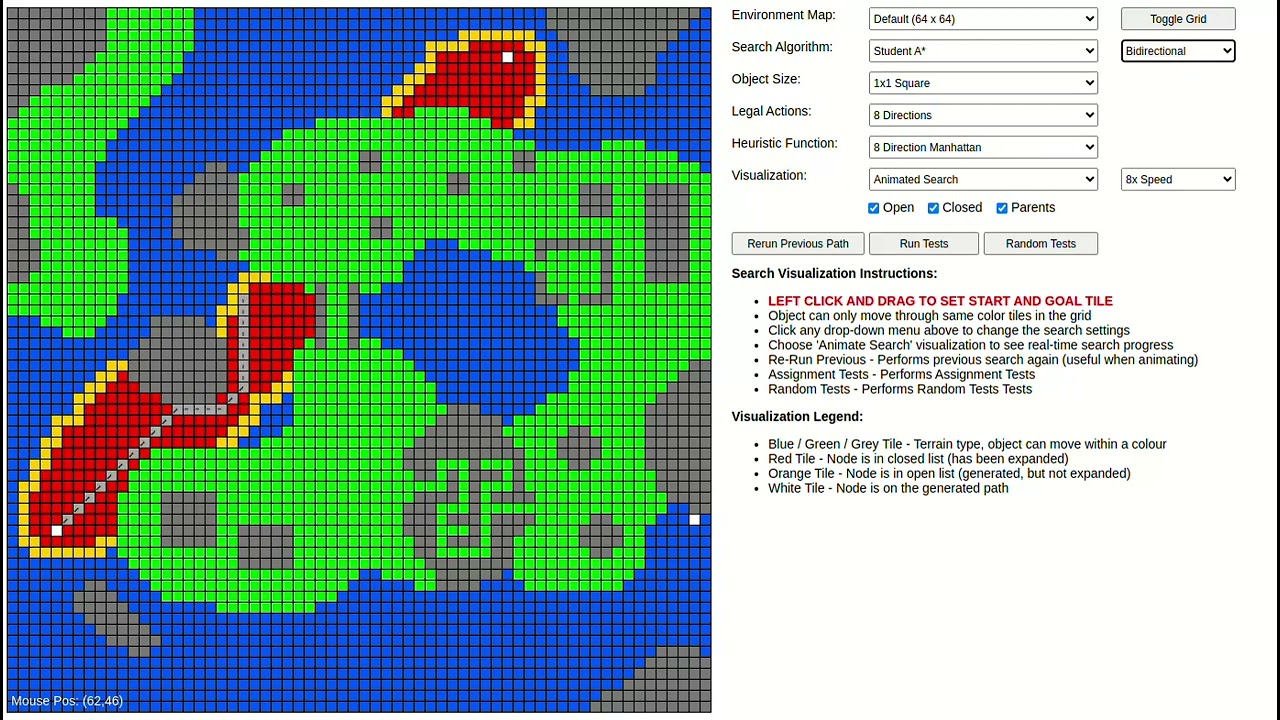](https://youtu.be/DajZy4A2tA8) |