Commit 7e288a4
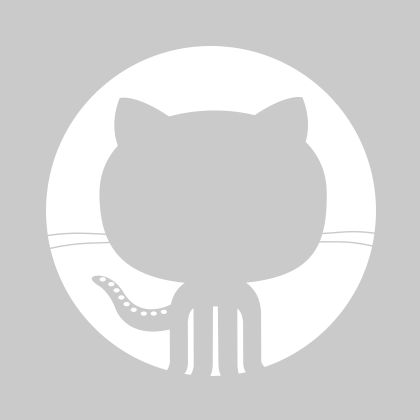
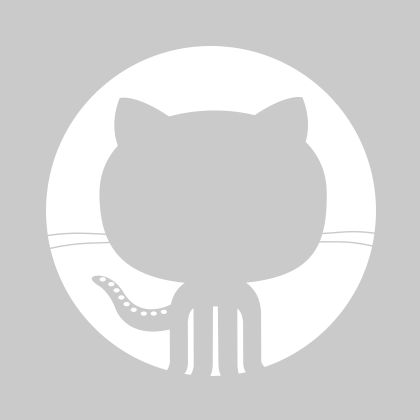
igor.stulikov
igor.stulikov
1 parent a406468 commit 7e288a4
File tree
3 files changed
+200
-14
lines changed- httpcore/backends
- tests
- _async
- _sync
3 files changed
+200
-14
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
| 2 | + | |
2 | 3 |
| |
3 |
| - | |
| 4 | + | |
4 | 5 |
| |
5 |
| - | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
6 | 9 |
| |
| 10 | + | |
7 | 11 |
| |
8 | 12 |
| |
9 | 13 |
| |
| |||
45 | 49 |
| |
46 | 50 |
| |
47 | 51 |
| |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
48 | 60 |
| |
49 |
| - | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
50 | 67 |
| |
51 | 68 |
| |
| 69 | + | |
52 | 70 |
| |
53 | 71 |
| |
54 | 72 |
| |
| |||
57 | 75 |
| |
58 | 76 |
| |
59 | 77 |
| |
60 |
| - | |
| 78 | + | |
61 | 79 |
| |
62 | 80 |
| |
63 | 81 |
| |
64 | 82 |
| |
65 |
| - | |
| 83 | + | |
66 | 84 |
| |
67 | 85 |
| |
68 | 86 |
| |
| |||
99 | 117 |
| |
100 | 118 |
| |
101 | 119 |
| |
| 120 | + | |
| 121 | + | |
| 122 | + | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
| 126 | + | |
| 127 | + | |
102 | 128 |
| |
103 |
| - | |
| 129 | + | |
| 130 | + | |
| 131 | + | |
| 132 | + | |
| 133 | + | |
| 134 | + | |
104 | 135 |
| |
105 | 136 |
| |
| 137 | + | |
106 | 138 |
| |
107 | 139 |
| |
108 | 140 |
| |
| |||
111 | 143 |
| |
112 | 144 |
| |
113 | 145 |
| |
114 |
| - | |
| 146 | + | |
115 | 147 |
| |
116 | 148 |
| |
117 | 149 |
| |
118 | 150 |
| |
119 |
| - | |
| 151 | + | |
120 | 152 |
| |
121 | 153 |
| |
122 | 154 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 |
| - | |
| 1 | + | |
| 2 | + | |
2 | 3 |
| |
3 | 4 |
| |
4 | 5 |
| |
| |||
7 | 8 |
| |
8 | 9 |
| |
9 | 10 |
| |
| 11 | + | |
10 | 12 |
| |
11 | 13 |
| |
12 | 14 |
| |
13 | 15 |
| |
14 |
| - | |
| 16 | + | |
15 | 17 |
| |
16 | 18 |
| |
17 | 19 |
| |
| |||
502 | 504 |
| |
503 | 505 |
| |
504 | 506 |
| |
| 507 | + | |
| 508 | + | |
| 509 | + | |
| 510 | + | |
| 511 | + | |
| 512 | + | |
| 513 | + | |
| 514 | + | |
| 515 | + | |
| 516 | + | |
| 517 | + | |
| 518 | + | |
| 519 | + | |
| 520 | + | |
| 521 | + | |
| 522 | + | |
| 523 | + | |
| 524 | + | |
| 525 | + | |
| 526 | + | |
| 527 | + | |
| 528 | + | |
| 529 | + | |
| 530 | + | |
| 531 | + | |
| 532 | + | |
| 533 | + | |
| 534 | + | |
| 535 | + | |
| 536 | + | |
| 537 | + | |
| 538 | + | |
| 539 | + | |
| 540 | + | |
| 541 | + | |
| 542 | + | |
| 543 | + | |
505 | 544 |
| |
506 | 545 |
| |
507 | 546 |
| |
| |||
534 | 573 |
| |
535 | 574 |
| |
536 | 575 |
| |
| 576 | + | |
| 577 | + | |
| 578 | + | |
| 579 | + | |
| 580 | + | |
| 581 | + | |
| 582 | + | |
| 583 | + | |
| 584 | + | |
| 585 | + | |
| 586 | + | |
| 587 | + | |
| 588 | + | |
| 589 | + | |
| 590 | + | |
| 591 | + | |
| 592 | + | |
| 593 | + | |
| 594 | + | |
| 595 | + | |
| 596 | + | |
| 597 | + | |
| 598 | + | |
| 599 | + | |
| 600 | + | |
| 601 | + | |
| 602 | + | |
| 603 | + | |
| 604 | + | |
| 605 | + | |
| 606 | + | |
| 607 | + | |
| 608 | + | |
| 609 | + | |
| 610 | + | |
| 611 | + | |
| 612 | + | |
| 613 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 |
| - | |
| 1 | + | |
| 2 | + | |
| 3 | + | |
2 | 4 |
| |
3 | 5 |
| |
4 |
| - | |
5 | 6 |
| |
6 | 7 |
| |
7 | 8 |
| |
8 | 9 |
| |
9 | 10 |
| |
| 11 | + | |
10 | 12 |
| |
11 | 13 |
| |
12 | 14 |
| |
13 | 15 |
| |
14 |
| - | |
15 |
| - | |
| 16 | + | |
| 17 | + | |
16 | 18 |
| |
17 | 19 |
| |
18 | 20 |
| |
| |||
503 | 505 |
| |
504 | 506 |
| |
505 | 507 |
| |
| 508 | + | |
| 509 | + | |
| 510 | + | |
| 511 | + | |
| 512 | + | |
| 513 | + | |
| 514 | + | |
| 515 | + | |
| 516 | + | |
| 517 | + | |
| 518 | + | |
| 519 | + | |
| 520 | + | |
| 521 | + | |
| 522 | + | |
| 523 | + | |
| 524 | + | |
| 525 | + | |
| 526 | + | |
| 527 | + | |
| 528 | + | |
| 529 | + | |
| 530 | + | |
| 531 | + | |
| 532 | + | |
| 533 | + | |
| 534 | + | |
| 535 | + | |
| 536 | + | |
| 537 | + | |
| 538 | + | |
| 539 | + | |
| 540 | + | |
| 541 | + | |
| 542 | + | |
| 543 | + | |
| 544 | + | |
| 545 | + | |
| 546 | + | |
| 547 | + | |
| 548 | + | |
| 549 | + | |
| 550 | + | |
| 551 | + | |
| 552 | + | |
| 553 | + | |
| 554 | + | |
| 555 | + | |
| 556 | + | |
| 557 | + | |
| 558 | + | |
| 559 | + | |
| 560 | + | |
| 561 | + | |
| 562 | + | |
| 563 | + | |
| 564 | + | |
| 565 | + | |
| 566 | + | |
| 567 | + | |
| 568 | + | |
| 569 | + | |
| 570 | + | |
| 571 | + | |
| 572 | + | |
| 573 | + | |
| 574 | + | |
| 575 | + | |
| 576 | + | |
| 577 | + | |
| 578 | + | |
| 579 | + | |
| 580 | + | |
| 581 | + | |
| 582 | + | |
506 | 583 |
| |
507 | 584 |
| |
508 | 585 |
| |
|
0 commit comments