Commit a406468
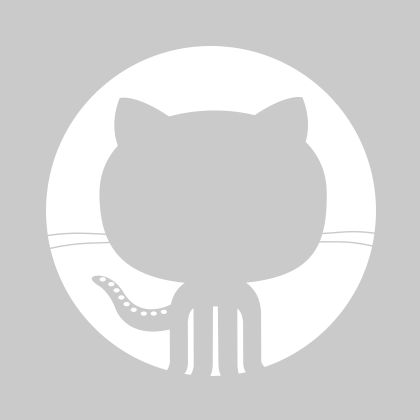
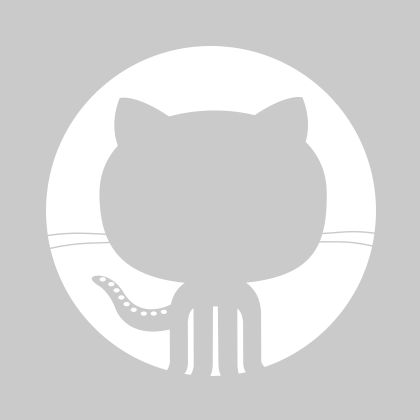
igor.stulikov
igor.stulikov
1 parent 7eb2022 commit a406468
File tree
24 files changed
+112
-0
lines changed- httpcore
- _async
- _sync
- tests
- _async
- _sync
24 files changed
+112
-0
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
185 | 185 |
| |
186 | 186 |
| |
187 | 187 |
| |
| 188 | + | |
| 189 | + | |
| 190 | + | |
188 | 191 |
| |
189 | 192 |
| |
190 | 193 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
140 | 140 |
| |
141 | 141 |
| |
142 | 142 |
| |
| 143 | + | |
| 144 | + | |
| 145 | + | |
| 146 | + | |
143 | 147 |
| |
144 | 148 |
| |
145 | 149 |
| |
| |||
168 | 172 |
| |
169 | 173 |
| |
170 | 174 |
| |
| 175 | + | |
| 176 | + | |
| 177 | + | |
| 178 | + | |
| 179 | + | |
| 180 | + | |
| 181 | + | |
| 182 | + | |
| 183 | + | |
| 184 | + | |
| 185 | + | |
| 186 | + | |
| 187 | + | |
| 188 | + | |
| 189 | + | |
171 | 190 |
| |
172 | 191 |
| |
173 | 192 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
269 | 269 |
| |
270 | 270 |
| |
271 | 271 |
| |
| 272 | + | |
| 273 | + | |
| 274 | + | |
272 | 275 |
| |
273 | 276 |
| |
274 | 277 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
411 | 411 |
| |
412 | 412 |
| |
413 | 413 |
| |
| 414 | + | |
| 415 | + | |
| 416 | + | |
414 | 417 |
| |
415 | 418 |
| |
416 | 419 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
204 | 204 |
| |
205 | 205 |
| |
206 | 206 |
| |
| 207 | + | |
| 208 | + | |
| 209 | + | |
207 | 210 |
| |
208 | 211 |
| |
209 | 212 |
| |
| |||
336 | 339 |
| |
337 | 340 |
| |
338 | 341 |
| |
| 342 | + | |
| 343 | + | |
| 344 | + | |
339 | 345 |
| |
340 | 346 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
133 | 133 |
| |
134 | 134 |
| |
135 | 135 |
| |
| 136 | + | |
| 137 | + | |
| 138 | + | |
| 139 | + | |
| 140 | + | |
| 141 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
329 | 329 |
| |
330 | 330 |
| |
331 | 331 |
| |
| 332 | + | |
| 333 | + | |
| 334 | + | |
332 | 335 |
| |
333 | 336 |
| |
334 | 337 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
185 | 185 |
| |
186 | 186 |
| |
187 | 187 |
| |
| 188 | + | |
| 189 | + | |
| 190 | + | |
188 | 191 |
| |
189 | 192 |
| |
190 | 193 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
140 | 140 |
| |
141 | 141 |
| |
142 | 142 |
| |
| 143 | + | |
| 144 | + | |
| 145 | + | |
| 146 | + | |
143 | 147 |
| |
144 | 148 |
| |
145 | 149 |
| |
| |||
168 | 172 |
| |
169 | 173 |
| |
170 | 174 |
| |
| 175 | + | |
| 176 | + | |
| 177 | + | |
| 178 | + | |
| 179 | + | |
| 180 | + | |
| 181 | + | |
| 182 | + | |
| 183 | + | |
| 184 | + | |
| 185 | + | |
| 186 | + | |
| 187 | + | |
| 188 | + | |
| 189 | + | |
171 | 190 |
| |
172 | 191 |
| |
173 | 192 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
269 | 269 |
| |
270 | 270 |
| |
271 | 271 |
| |
| 272 | + | |
| 273 | + | |
| 274 | + | |
272 | 275 |
| |
273 | 276 |
| |
274 | 277 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
411 | 411 |
| |
412 | 412 |
| |
413 | 413 |
| |
| 414 | + | |
| 415 | + | |
| 416 | + | |
414 | 417 |
| |
415 | 418 |
| |
416 | 419 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
204 | 204 |
| |
205 | 205 |
| |
206 | 206 |
| |
| 207 | + | |
| 208 | + | |
| 209 | + | |
207 | 210 |
| |
208 | 211 |
| |
209 | 212 |
| |
| |||
336 | 339 |
| |
337 | 340 |
| |
338 | 341 |
| |
| 342 | + | |
| 343 | + | |
| 344 | + | |
339 | 345 |
| |
340 | 346 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
133 | 133 |
| |
134 | 134 |
| |
135 | 135 |
| |
| 136 | + | |
| 137 | + | |
| 138 | + | |
| 139 | + | |
| 140 | + | |
| 141 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
329 | 329 |
| |
330 | 330 |
| |
331 | 331 |
| |
| 332 | + | |
| 333 | + | |
| 334 | + | |
332 | 335 |
| |
333 | 336 |
| |
334 | 337 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
29 | 29 |
| |
30 | 30 |
| |
31 | 31 |
| |
| 32 | + | |
32 | 33 |
| |
33 | 34 |
| |
34 | 35 |
| |
| |||
45 | 46 |
| |
46 | 47 |
| |
47 | 48 |
| |
| 49 | + | |
48 | 50 |
| |
49 | 51 |
| |
50 | 52 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
33 | 33 |
| |
34 | 34 |
| |
35 | 35 |
| |
| 36 | + | |
36 | 37 |
| |
37 | 38 |
| |
38 | 39 |
| |
| |||
63 | 64 |
| |
64 | 65 |
| |
65 | 66 |
| |
| 67 | + | |
66 | 68 |
| |
67 | 69 |
| |
68 | 70 |
| |
| |||
85 | 87 |
| |
86 | 88 |
| |
87 | 89 |
| |
| 90 | + | |
88 | 91 |
| |
89 | 92 |
| |
90 | 93 |
| |
| |||
114 | 117 |
| |
115 | 118 |
| |
116 | 119 |
| |
| 120 | + | |
117 | 121 |
| |
118 | 122 |
| |
119 | 123 |
| |
| |||
146 | 150 |
| |
147 | 151 |
| |
148 | 152 |
| |
| 153 | + | |
149 | 154 |
| |
150 | 155 |
| |
151 | 156 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
43 | 43 |
| |
44 | 44 |
| |
45 | 45 |
| |
| 46 | + | |
46 | 47 |
| |
47 | 48 |
| |
48 | 49 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
47 | 47 |
| |
48 | 48 |
| |
49 | 49 |
| |
| 50 | + | |
50 | 51 |
| |
51 | 52 |
| |
52 | 53 |
| |
| |||
102 | 103 |
| |
103 | 104 |
| |
104 | 105 |
| |
| 106 | + | |
105 | 107 |
| |
106 | 108 |
| |
107 | 109 |
| |
| |||
193 | 195 |
| |
194 | 196 |
| |
195 | 197 |
| |
| 198 | + | |
196 | 199 |
| |
197 | 200 |
| |
198 | 201 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
46 | 46 |
| |
47 | 47 |
| |
48 | 48 |
| |
| 49 | + | |
49 | 50 |
| |
50 | 51 |
| |
51 | 52 |
| |
| |||
107 | 108 |
| |
108 | 109 |
| |
109 | 110 |
| |
| 111 | + | |
110 | 112 |
| |
111 | 113 |
| |
112 | 114 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
29 | 29 |
| |
30 | 30 |
| |
31 | 31 |
| |
| 32 | + | |
32 | 33 |
| |
33 | 34 |
| |
34 | 35 |
| |
| |||
45 | 46 |
| |
46 | 47 |
| |
47 | 48 |
| |
| 49 | + | |
48 | 50 |
| |
49 | 51 |
| |
50 | 52 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
33 | 33 |
| |
34 | 34 |
| |
35 | 35 |
| |
| 36 | + | |
36 | 37 |
| |
37 | 38 |
| |
38 | 39 |
| |
| |||
63 | 64 |
| |
64 | 65 |
| |
65 | 66 |
| |
| 67 | + | |
66 | 68 |
| |
67 | 69 |
| |
68 | 70 |
| |
| |||
85 | 87 |
| |
86 | 88 |
| |
87 | 89 |
| |
| 90 | + | |
88 | 91 |
| |
89 | 92 |
| |
90 | 93 |
| |
| |||
114 | 117 |
| |
115 | 118 |
| |
116 | 119 |
| |
| 120 | + | |
117 | 121 |
| |
118 | 122 |
| |
119 | 123 |
| |
| |||
146 | 150 |
| |
147 | 151 |
| |
148 | 152 |
| |
| 153 | + | |
149 | 154 |
| |
150 | 155 |
| |
151 | 156 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
43 | 43 |
| |
44 | 44 |
| |
45 | 45 |
| |
| 46 | + | |
46 | 47 |
| |
47 | 48 |
| |
48 | 49 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
47 | 47 |
| |
48 | 48 |
| |
49 | 49 |
| |
| 50 | + | |
50 | 51 |
| |
51 | 52 |
| |
52 | 53 |
| |
| |||
102 | 103 |
| |
103 | 104 |
| |
104 | 105 |
| |
| 106 | + | |
105 | 107 |
| |
106 | 108 |
| |
107 | 109 |
| |
| |||
193 | 195 |
| |
194 | 196 |
| |
195 | 197 |
| |
| 198 | + | |
196 | 199 |
| |
197 | 200 |
| |
198 | 201 |
| |
|
0 commit comments