diff --git a/README.md b/README.md index 0d54d87..961b1c0 100644 --- a/README.md +++ b/README.md @@ -1,3 +1,6 @@ +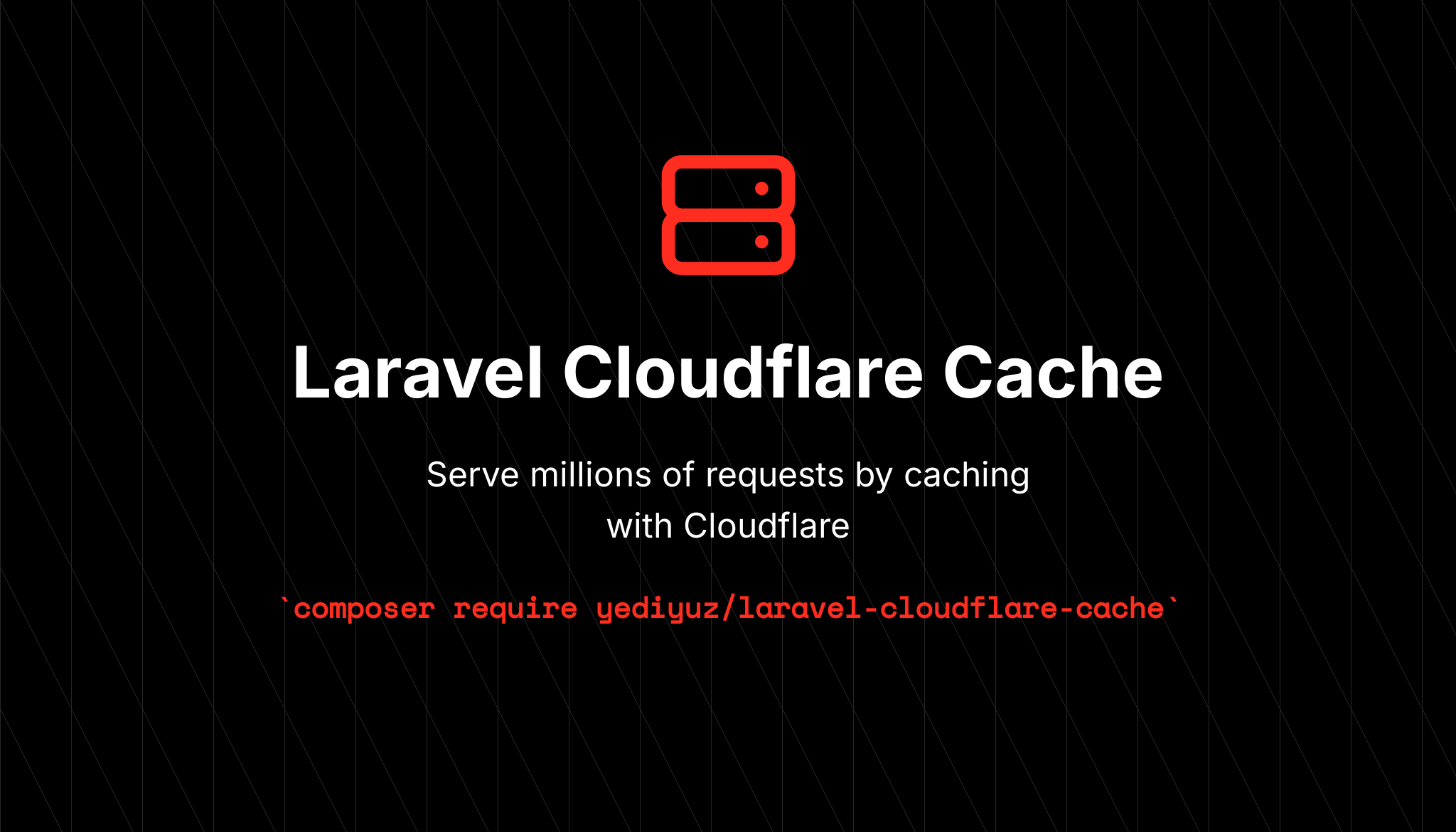 +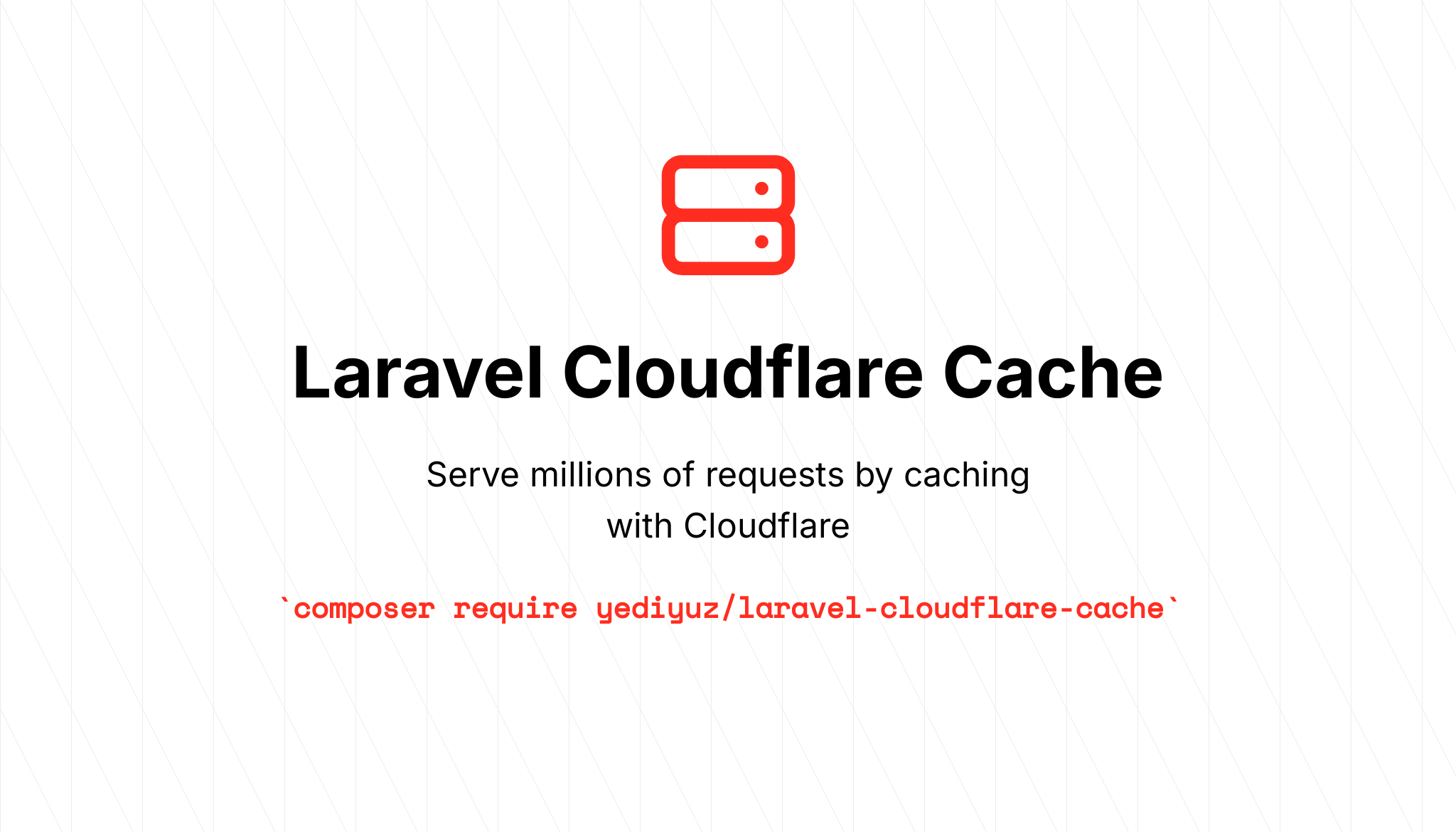 + # Laravel Cloudflare Cache
@@ -14,6 +17,112 @@ You can install the package via composer: composer require yediyuz/laravel-cloudflare-cache ``` +You can publish the config file with: + +```bash +php artisan vendor:publish --tag="cloudflare-cache-config" +``` + +Add environment variables to .env file +```dotenv +CLOUDFLARE_CACHE_EMAIL=info@example.com #Cloudflare account email address +CLOUDFLARE_CACHE_KEY=XXXXXXX #Cloudflare API_KEY +CLOUDFLARE_CACHE_IDENTIFIER=XXXXXXX #ZONE_ID +CLOUDFLARE_DEFAULT_CACHE_TTL=600 #10 minutes +CLOUDFLARE_CACHE_DEBUG=false +``` + +## Usage + +### Define routes to cache +You can use cache groups for your static contents. +```php +Route::cache()->group(function () { + Route::get('/content', function () { + return 'content'; + }); +}); +``` + +You can use cache tags, so you can clear your caches easily. Specify custom ttl for expire time in seconds. When you do not pass ttl, it will use default ttl given in the config. +```php +Route::cache(tags: ['tag1', 'tag2'], ttl: 600)->group(function () { + Route::get('/content_with_tags', function () { + return 'content'; + }); +}); + +Route::cache(tags: ['staticPages'])->group(function () { + // +}); +``` +### Clear Cache + +#### Purges everything +https://developers.cloudflare.com/cache/how-to/purge-cache/purge-everything/ +```php +CloudflareCache::purgeEverything() +``` + +#### Purges by urls +https://developers.cloudflare.com/cache/how-to/purge-cache/purge-by-single-file/ +```php +CloudflareCache::purgeByUrls([ + 'https://example.com/hello', +]) +``` + +#### Purges by prefixes (Enterprise only) +https://developers.cloudflare.com/cache/how-to/purge-cache/purge_by_prefix/ +```php +CloudflareCache::purgeByPrefixes([ + 'www.example.com/foo', +]) +``` + +#### Purges by tags (Enterprise only) +https://developers.cloudflare.com/cache/how-to/purge-cache/purge-by-tags/ +```php +CloudflareCache::purgeByTags([ + 'staticPages', +]) +``` + +#### Purges by hostname (Enterprise only) +https://developers.cloudflare.com/cache/how-to/purge-cache/purge-by-hostname/ +```php +CloudflareCache::purgeByHosts([ + 'www.example.com', + 'images.example.com', +]) +``` + +Post update example to clear cache +```php +update($request->validated()); + + CloudflareCache::purgeByUrls([ + route('post.show', $post->id) + ]); + + return back()->with('message', 'Post updated and url cache purged'); + } +} +``` + + ## Testing ```bash diff --git a/config/cloudflare-cache.php b/config/cloudflare-cache.php index eeeac44..43b92ee 100644 --- a/config/cloudflare-cache.php +++ b/config/cloudflare-cache.php @@ -5,10 +5,13 @@ return [ 'debug' => env('CLOUDFLARE_CACHE_DEBUG', false), + // your cloudflare email address 'api_email' => env('CLOUDFLARE_CACHE_EMAIL'), + // Generate global api key https://dash.cloudflare.com/profile/api-tokens 'api_key' => env('CLOUDFLARE_CACHE_KEY'), + // zone_id of your site on cloudflare dashboard 'identifier' => env('CLOUDFLARE_CACHE_IDENTIFIER'), 'default_cache_ttl' => env('CLOUDFLARE_DEFAULT_CACHE_TTL'),