- {row.map((cell, x) => (
-
{
- const entity = simulator.current?.getEntityAt({
- x,
- y,
- });
- if (
- entity?.getType() === EntityType.Zombie &&
- entity.getHealth() === 1
- ) {
- return 0.5;
- }
- return 1;
- })(),
- }}
- className={`border flex items-center justify-center dark:bg-black bg-slate-50`}
- >
- {cell}
+ <>
+
+
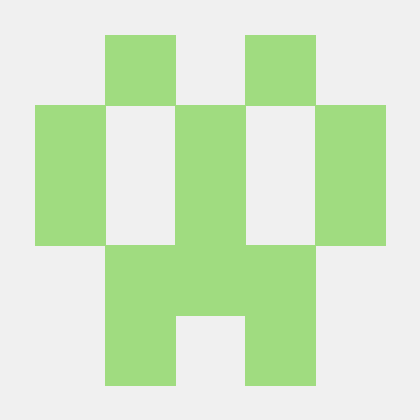
+
+ {mapState.map((row, y) => (
+
+ {row.map((cell, x) => (
+
{
+ const entity = simulator.current?.getEntityAt({
+ x,
+ y,
+ });
+ if (
+ entity?.getType() === EntityType.Zombie &&
+ entity.getHealth() === 1
+ ) {
+ return 0.5;
+ }
+ return 1;
+ })(),
+ }}
+ className={`border flex items-center justify-center`}
+ >
+ {getCellImage(cell)}
+
+ ))}
))}
- ))}
- {controls && (
-
-
-
-
- )}
-
+
+
+ {controls && (
+
+
+
+
+ )}
+
+ >
);
}
diff --git a/convex/maps.ts b/convex/maps.ts
index e404171..b90d37e 100644
--- a/convex/maps.ts
+++ b/convex/maps.ts
@@ -45,15 +45,6 @@ const LEVELS = [
[" ", " ", "Z"],
],
},
- {
- grid: [
- [" ", " ", "R", " ", "Z"],
- [" ", " ", " ", " ", "B"],
- [" ", " ", " ", "R", " "],
- ["Z", " ", " ", " ", " "],
- [" ", " ", "B", " ", " "],
- ],
- },
];
export const addMap = mutation({
diff --git a/public/entities/block.svg b/public/entities/block.svg
new file mode 100644
index 0000000..1fba712
--- /dev/null
+++ b/public/entities/block.svg
@@ -0,0 +1,29 @@
+
diff --git a/public/entities/player_alive_1.svg b/public/entities/player_alive_1.svg
new file mode 100644
index 0000000..4d3cb92
--- /dev/null
+++ b/public/entities/player_alive_1.svg
@@ -0,0 +1,479 @@
+
diff --git a/public/entities/player_alive_2.svg b/public/entities/player_alive_2.svg
new file mode 100644
index 0000000..977d276
--- /dev/null
+++ b/public/entities/player_alive_2.svg
@@ -0,0 +1,479 @@
+
diff --git a/public/entities/rocks.png b/public/entities/rocks.png
new file mode 100644
index 0000000..1f2e53a
Binary files /dev/null and b/public/entities/rocks.png differ
diff --git a/public/entities/zombie_alive_1.svg b/public/entities/zombie_alive_1.svg
new file mode 100644
index 0000000..cfa8061
--- /dev/null
+++ b/public/entities/zombie_alive_1.svg
@@ -0,0 +1,311 @@
+
diff --git a/public/entities/zombie_alive_2.svg b/public/entities/zombie_alive_2.svg
new file mode 100644
index 0000000..0b274f3
--- /dev/null
+++ b/public/entities/zombie_alive_2.svg
@@ -0,0 +1,311 @@
+
diff --git a/public/entities/zombie_dead.svg b/public/entities/zombie_dead.svg
new file mode 100644
index 0000000..e66c93c
--- /dev/null
+++ b/public/entities/zombie_dead.svg
@@ -0,0 +1,227 @@
+
diff --git a/public/map.png b/public/map.png
new file mode 100644
index 0000000..690c534
Binary files /dev/null and b/public/map.png differ