You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
HParams: Update tb_http_client to support the hparams plugin backend take 2 (#6340)
## Motivation for features / changes
As part of the effort to surface hparams data in the time series
dashboard we need to start actually fetching it. The logic existed
internally but for some reason was not previously available in OSS.
I originally attempted this in #6318 but due to incompatibilities with
internal Colab had to revert it in #6336.
The issue was that Colab does not allow `POST` requests and our method
of mapping POST requests to GET requests didn't meet the expectations of
the hparams_plugin backend.
I've improved the tb_http_client in #6337 to now work with the
hparams_plugin backend so this should now be fine
## Technical description of changes
I mostly copied the internal code to the oss implementation and made a
few small adjustments to imports.
The biggest change is that I now utilize the `serializeUnder` parameter
of our custom POST function to ensure the requests meet the expectations
of the hparams backend
## Screenshots of UI changes
### enableHparamsInTimeSeries=false&tensorboardColab=false
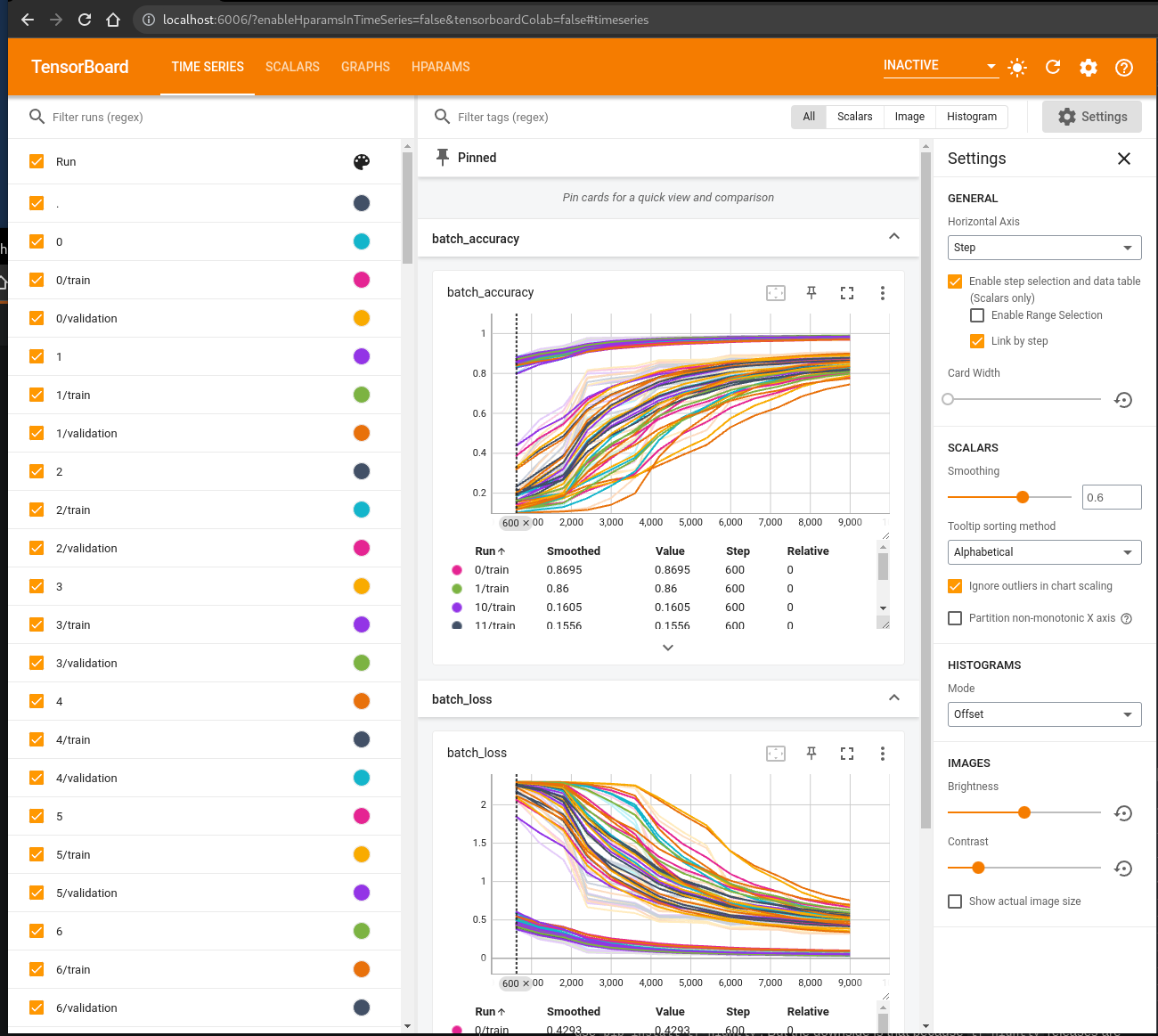
### enableHparamsInTimeSeries=true&tensorboardColab=false
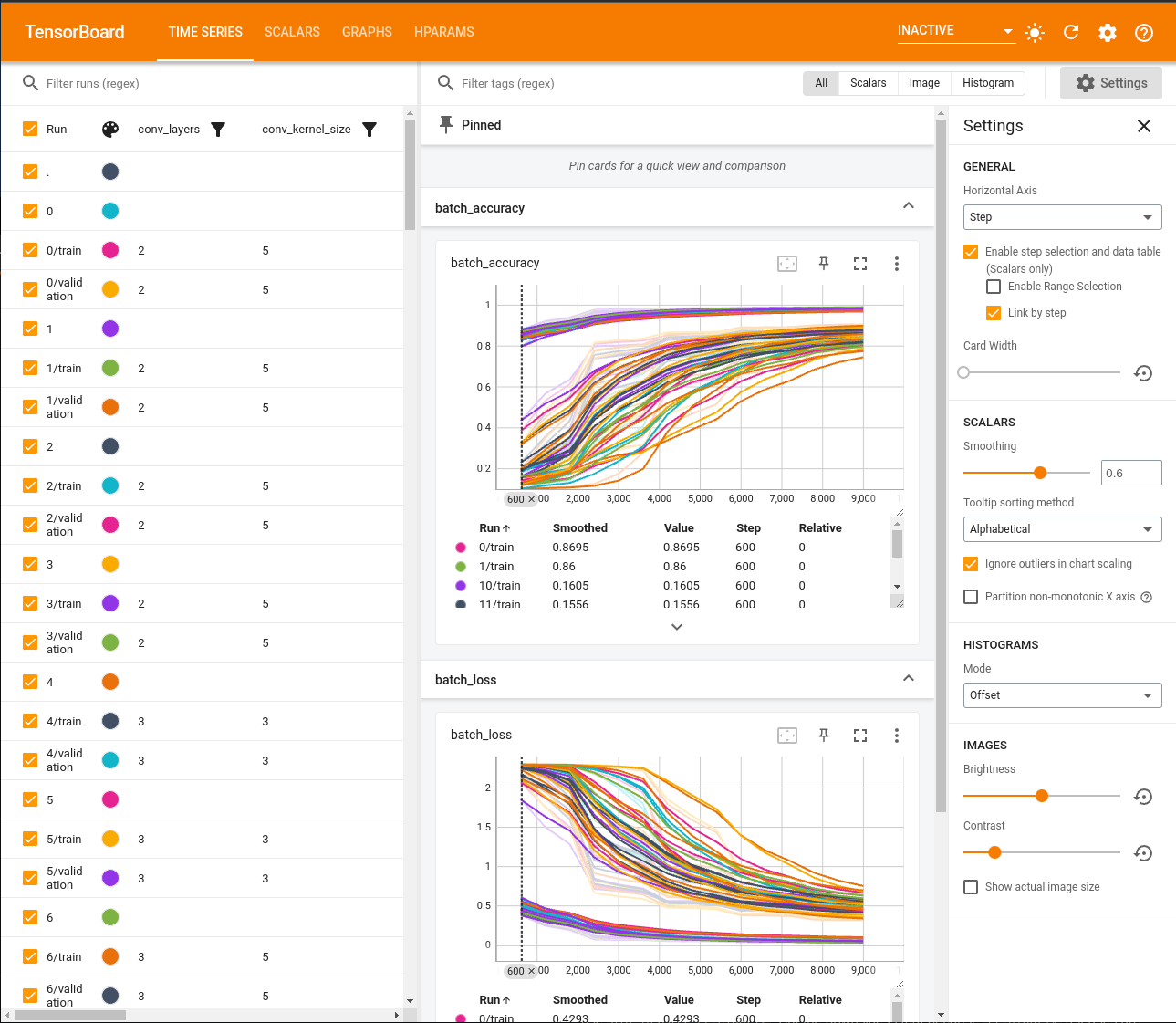
### enableHparamsInTimeSeries=false&tensorboardColab=true
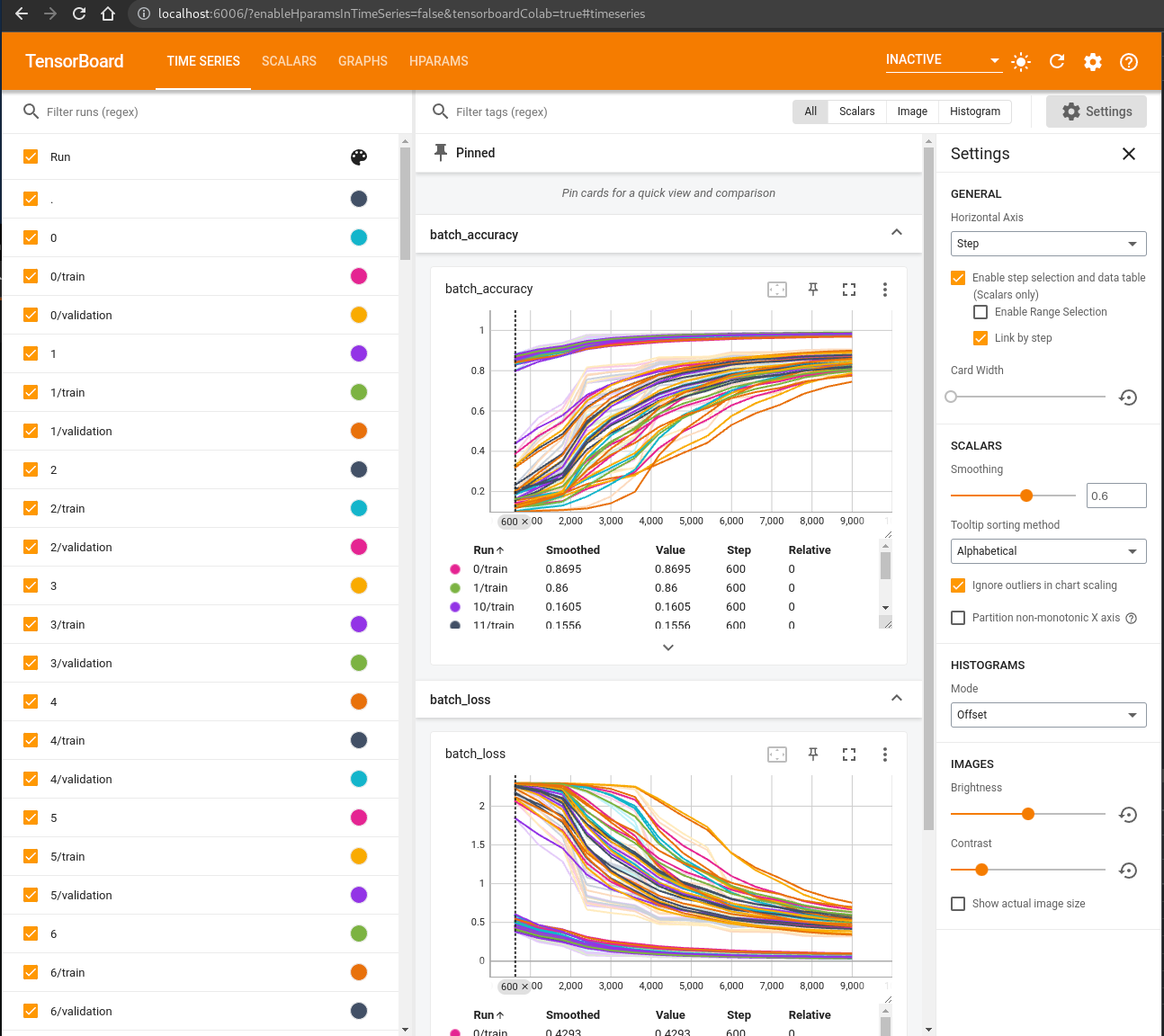
### enableHparamsInTimeSeries=true&tensorboardColab=true
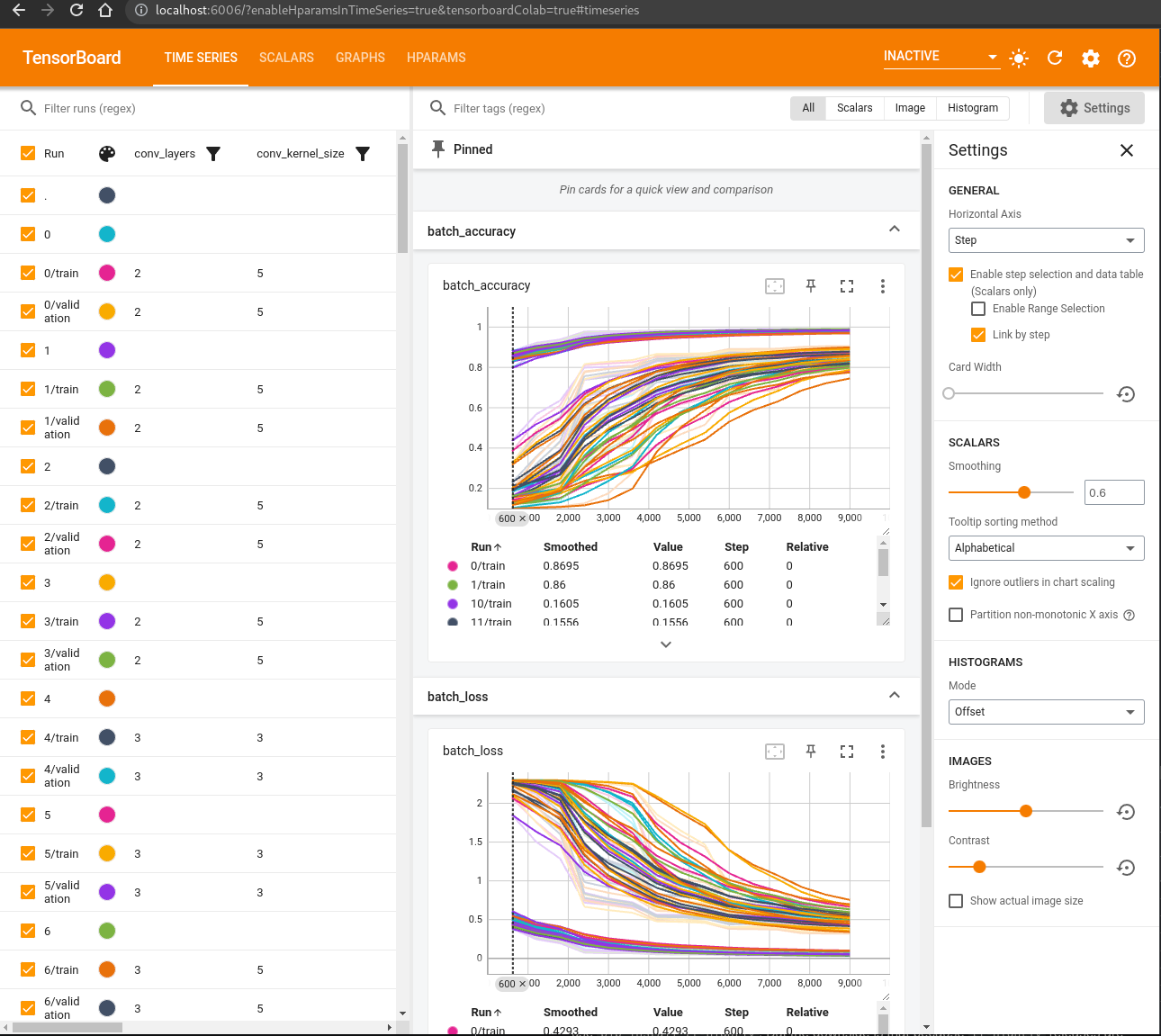
## Detailed steps to verify changes work correctly (as executed by you)
I ensured all of the above states result in the runs table being
displayed properly and that no errors are thrown by the hparams plugin
backend
0 commit comments