datasetId,
* Returns the cardinality of {@code input_dataset}.
*
* @param inputDataset A variant tensor representing the dataset to return cardinality for.
+ * @param options carries optional attribute values
* @return a new instance of DatasetCardinality
*/
- public DatasetCardinality datasetCardinality(Operand extends TType> inputDataset) {
- return DatasetCardinality.create(scope, inputDataset);
+ public DatasetCardinality datasetCardinality(Operand extends TType> inputDataset,
+ DatasetCardinality.Options... options) {
+ return DatasetCardinality.create(scope, inputDataset, options);
}
/**
@@ -1302,27 +1304,29 @@ public PrivateThreadPoolDataset privateThreadPoolDataset(Operand extends TType
/**
* Creates a Dataset that returns pseudorandom numbers.
* Creates a Dataset that returns a stream of uniformly distributed
- * pseudorandom 64-bit signed integers.
+ * pseudorandom 64-bit signed integers. It accepts a boolean attribute that
+ * determines if the random number generators are re-applied at each epoch. The
+ * default value is True which means that the seeds are applied and the same
+ * sequence of random numbers are generated at each epoch. If set to False, the
+ * seeds are not re-applied and a different sequence of random numbers are
+ * generated at each epoch.
* In the TensorFlow Python API, you can instantiate this dataset via the
- * class {@code tf.data.experimental.RandomDataset}.
- *
Instances of this dataset are also created as a result of the
- * {@code hoist_random_uniform} static optimization. Whether this optimization is
- * performed is determined by the {@code experimental_optimization.hoist_random_uniform}
- * option of {@code tf.data.Options}.
+ * class {@code tf.data.experimental.RandomDatasetV2}.
*
* @param seed A scalar seed for the random number generator. If either seed or
* seed2 is set to be non-zero, the random number generator is seeded
* by the given seed. Otherwise, a random seed is used.
* @param seed2 A second scalar seed to avoid seed collision.
+ * @param seedGenerator A resource for the random number seed generator.
* @param outputTypes The value of the outputTypes attribute
* @param outputShapes The value of the outputShapes attribute
* @param options carries optional attribute values
* @return a new instance of RandomDataset
*/
public RandomDataset randomDataset(Operand seed, Operand seed2,
- List> outputTypes, List outputShapes,
- RandomDataset.Options... options) {
- return RandomDataset.create(scope, seed, seed2, outputTypes, outputShapes, options);
+ Operand extends TType> seedGenerator, List> outputTypes,
+ List outputShapes, RandomDataset.Options... options) {
+ return RandomDataset.create(scope, seed, seed2, seedGenerator, outputTypes, outputShapes, options);
}
/**
@@ -1768,13 +1772,15 @@ public TextLineDataset textLineDataset(Operand filenames,
* compression), (ii) "ZLIB", or (iii) "GZIP".
* @param bufferSize A scalar representing the number of bytes to buffer. A value of
* 0 means no buffering will be performed.
+ * @param byteOffsets A scalar or vector containing the number of bytes for each file
+ * that will be skipped prior to reading.
* @param options carries optional attribute values
* @return a new instance of TfRecordDataset
*/
public TfRecordDataset tfRecordDataset(Operand filenames,
- Operand compressionType, Operand bufferSize,
+ Operand compressionType, Operand bufferSize, Operand byteOffsets,
TfRecordDataset.Options... options) {
- return TfRecordDataset.create(scope, filenames, compressionType, bufferSize, options);
+ return TfRecordDataset.create(scope, filenames, compressionType, bufferSize, byteOffsets, options);
}
/**
diff --git a/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/MathOps.java b/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/MathOps.java
index 7b2d4faedfe..ae95a5c9cd6 100644
--- a/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/MathOps.java
+++ b/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/MathOps.java
@@ -253,7 +253,7 @@ public AddN addN(Iterable> inputs) {
* For example:
*
* # tensor 'input' is [-2.25 + 4.75j, 3.25 + 5.75j]
- * tf.angle(input) ==> [2.0132, 1.056]
+ * tf.math.angle(input) ==> [2.0132, 1.056]
*
* {@literal @}compatibility(numpy)
* Equivalent to np.angle.
@@ -277,7 +277,7 @@ public Angle angle(Operand extends TType> input) {
* For example:
*
* # tensor 'input' is [-2.25 + 4.75j, 3.25 + 5.75j]
- * tf.angle(input) ==> [2.0132, 1.056]
+ * tf.math.angle(input) ==> [2.0132, 1.056]
*
* {@literal @}compatibility(numpy)
* Equivalent to np.angle.
@@ -994,9 +994,10 @@ public FloorDiv floorDiv(Operand x, Operand y) {
}
/**
- * Returns element-wise remainder of division. When {@code x < 0} xor {@code y < 0} is
- * true, this follows Python semantics in that the result here is consistent
- * with a flooring divide. E.g. {@code floor(x / y) * y + mod(x, y) = x}.
+ * Returns element-wise remainder of division.
+ * This follows Python semantics in that the
+ * result here is consistent with a flooring divide. E.g.
+ * {@code floor(x / y) * y + floormod(x, y) = x}, regardless of the signs of x and y.
* NOTE: {@code math.FloorMod} supports broadcasting. More about broadcasting
* here
*
@@ -1730,21 +1731,33 @@ public Rsqrt rsqrt(Operand x) {
* Computes a tensor such that
* \(output_i = \max_j(data_j)\) where {@code max} is over {@code j} such
* that {@code segment_ids[j] == i}.
- *
If the max is empty for a given segment ID {@code i}, {@code output[i] = 0}.
+ *
If the maximum is empty for a given segment ID {@code i}, it outputs the smallest
+ * possible value for the specific numeric type,
+ * {@code output[i] = numeric_limits::lowest()}.
+ * Note: That this op is currently only supported with jit_compile=True.
*
Caution: On CPU, values in {@code segment_ids} are always validated to be sorted,
* and an error is thrown for indices that are not increasing. On GPU, this
* does not throw an error for unsorted indices. On GPU, out-of-order indices
* result in safe but unspecified behavior, which may include treating
* out-of-order indices as the same as a smaller following index.
- *
- *
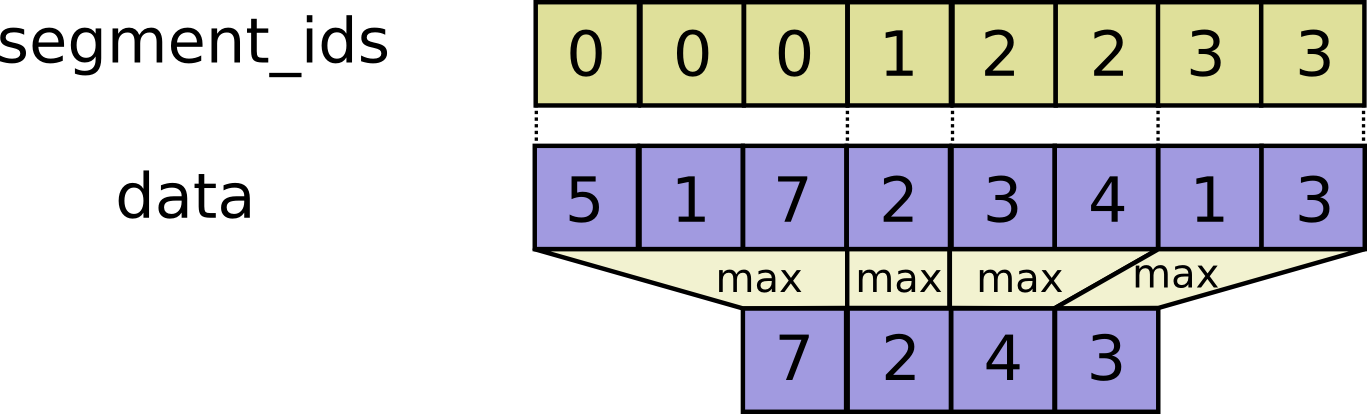
- *
+ * The only difference with SegmentMax is the additional input {@code num_segments}.
+ * This helps in evaluating the output shape in compile time.
+ * {@code num_segments} should be consistent with segment_ids.
+ * e.g. Max(segment_ids) should be equal to {@code num_segments} - 1 for a 1-d segment_ids
+ * With inconsistent num_segments, the op still runs. only difference is,
+ * the output takes the size of num_segments irrespective of size of segment_ids and data.
+ * for num_segments less than expected output size, the last elements are ignored
+ * for num_segments more than the expected output size, last elements are assigned
+ * smallest possible value for the specific numeric type.
*
For example:
*
*
*
- * c = tf.constant([[1,2,3,4], [4, 3, 2, 1], [5,6,7,8]])
- * tf.math.segment_max(c, tf.constant([0, 0, 1])).numpy()
+ *
{@literal @}tf.function(jit_compile=True)
+ * ... def test(c):
+ * ... return tf.raw_ops.SegmentMaxV2(data=c, segment_ids=tf.constant([0, 0, 1]), num_segments=2)
+ * c = tf.constant([[1,2,3,4], [4, 3, 2, 1], [5,6,7,8]])
+ * test(c).numpy()
* array([[4, 3, 3, 4],
* [5, 6, 7, 8]], dtype=int32)
*
@@ -1755,14 +1768,16 @@ public Rsqrt rsqrt(Operand x) {
* @param data The data value
* @param segmentIds A 1-D tensor whose size is equal to the size of {@code data}'s
* first dimension. Values should be sorted and can be repeated.
+ * The values must be less than {@code num_segments}.
* Caution: The values are always validated to be sorted on CPU, never validated
* on GPU.
- * @param data type for {@code SegmentMax} output and operands
+ * @param numSegments The numSegments value
+ * @param data type for {@code SegmentMaxV2} output and operands
* @return a new instance of SegmentMax
*/
public SegmentMax segmentMax(Operand data,
- Operand extends TNumber> segmentIds) {
- return SegmentMax.create(scope, data, segmentIds);
+ Operand extends TNumber> segmentIds, Operand extends TNumber> numSegments) {
+ return SegmentMax.create(scope, data, segmentIds, numSegments);
}
/**
@@ -1818,21 +1833,33 @@ public SegmentMean segmentMean(Operand data,
* Computes a tensor such that
* \(output_i = \min_j(data_j)\) where {@code min} is over {@code j} such
* that {@code segment_ids[j] == i}.
- *
If the min is empty for a given segment ID {@code i}, {@code output[i] = 0}.
+ *
If the minimum is empty for a given segment ID {@code i}, it outputs the largest
+ * possible value for the specific numeric type,
+ * {@code output[i] = numeric_limits::max()}.
+ * Note: That this op is currently only supported with jit_compile=True.
*
Caution: On CPU, values in {@code segment_ids} are always validated to be sorted,
* and an error is thrown for indices that are not increasing. On GPU, this
* does not throw an error for unsorted indices. On GPU, out-of-order indices
* result in safe but unspecified behavior, which may include treating
* out-of-order indices as the same as a smaller following index.
- *
- *
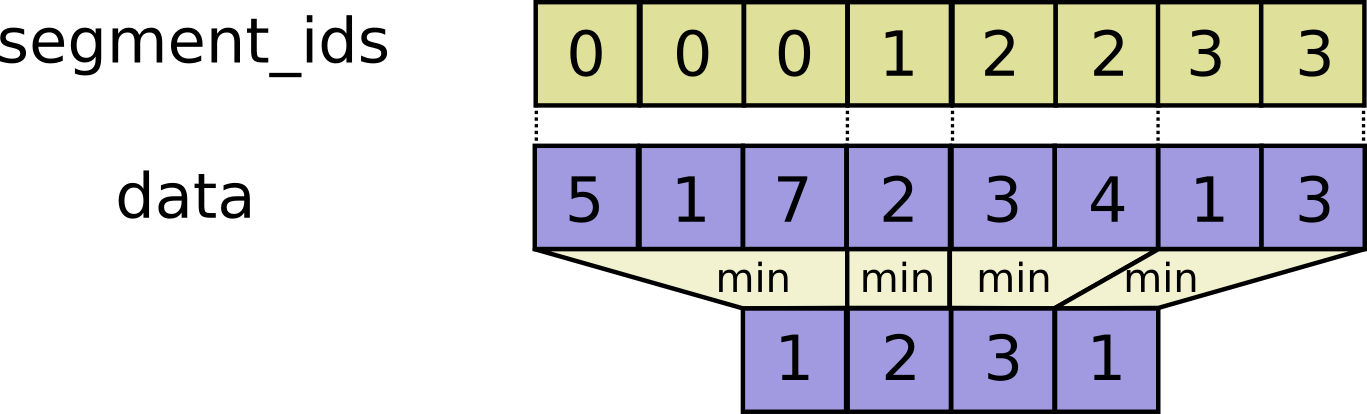
- *
+ * The only difference with SegmentMin is the additional input {@code num_segments}.
+ * This helps in evaluating the output shape in compile time.
+ * {@code num_segments} should be consistent with segment_ids.
+ * e.g. Max(segment_ids) should be equal to {@code num_segments} - 1 for a 1-d segment_ids
+ * With inconsistent num_segments, the op still runs. only difference is,
+ * the output takes the size of num_segments irrespective of size of segment_ids and data.
+ * for num_segments less than expected output size, the last elements are ignored
+ * for num_segments more than the expected output size, last elements are assigned
+ * the largest possible value for the specific numeric type.
*
For example:
*
*
*
- * c = tf.constant([[1,2,3,4], [4, 3, 2, 1], [5,6,7,8]])
- * tf.math.segment_min(c, tf.constant([0, 0, 1])).numpy()
+ *
{@literal @}tf.function(jit_compile=True)
+ * ... def test(c):
+ * ... return tf.raw_ops.SegmentMinV2(data=c, segment_ids=tf.constant([0, 0, 1]), num_segments=2)
+ * c = tf.constant([[1,2,3,4], [4, 3, 2, 1], [5,6,7,8]])
+ * test(c).numpy()
* array([[1, 2, 2, 1],
* [5, 6, 7, 8]], dtype=int32)
*
@@ -1843,14 +1870,16 @@ public SegmentMean segmentMean(Operand data,
* @param data The data value
* @param segmentIds A 1-D tensor whose size is equal to the size of {@code data}'s
* first dimension. Values should be sorted and can be repeated.
+ * The values must be less than {@code num_segments}.
* Caution: The values are always validated to be sorted on CPU, never validated
* on GPU.
- * @param data type for {@code SegmentMin} output and operands
+ * @param numSegments The numSegments value
+ * @param data type for {@code SegmentMinV2} output and operands
* @return a new instance of SegmentMin
*/
public SegmentMin segmentMin(Operand data,
- Operand extends TNumber> segmentIds) {
- return SegmentMin.create(scope, data, segmentIds);
+ Operand extends TNumber> segmentIds, Operand extends TNumber> numSegments) {
+ return SegmentMin.create(scope, data, segmentIds, numSegments);
}
/**
@@ -1862,20 +1891,24 @@ public SegmentMin segmentMin(Operand data,
* \(output_i = \prod_j data_j\) where the product is over {@code j} such
* that {@code segment_ids[j] == i}.
* If the product is empty for a given segment ID {@code i}, {@code output[i] = 1}.
- *
Caution: On CPU, values in {@code segment_ids} are always validated to be sorted,
- * and an error is thrown for indices that are not increasing. On GPU, this
- * does not throw an error for unsorted indices. On GPU, out-of-order indices
- * result in safe but unspecified behavior, which may include treating
- * out-of-order indices as the same as a smaller following index.
- *
- *
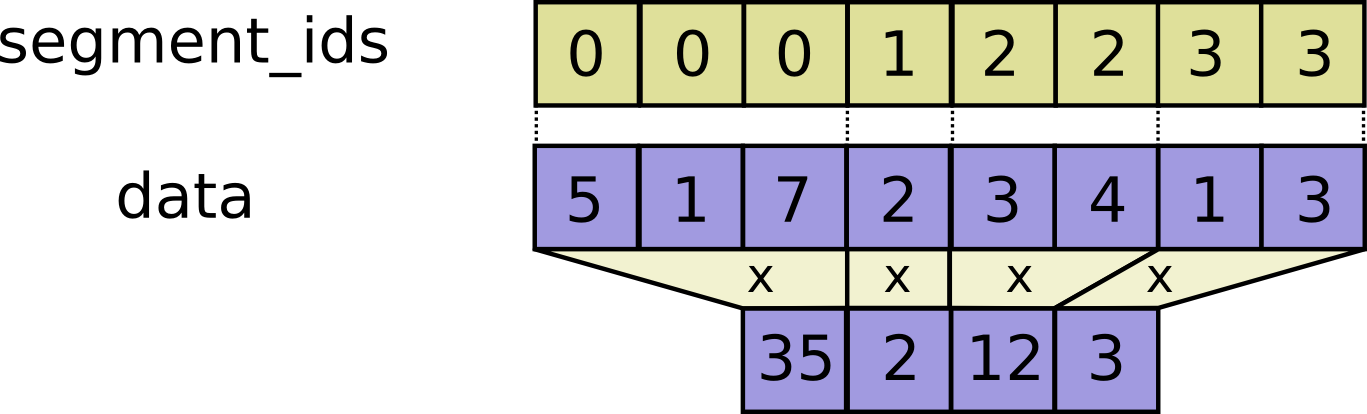
- *
+ * Note: That this op is currently only supported with jit_compile=True.
+ *
The only difference with SegmentProd is the additional input {@code num_segments}.
+ * This helps in evaluating the output shape in compile time.
+ * {@code num_segments} should be consistent with segment_ids.
+ * e.g. Max(segment_ids) - 1 should be equal to {@code num_segments} for a 1-d segment_ids
+ * With inconsistent num_segments, the op still runs. only difference is,
+ * the output takes the size of num_segments irrespective of size of segment_ids and data.
+ * for num_segments less than expected output size, the last elements are ignored
+ * for num_segments more than the expected output size, last elements are assigned 1.
*
For example:
*
*
*
- * c = tf.constant([[1,2,3,4], [4, 3, 2, 1], [5,6,7,8]])
- * tf.math.segment_prod(c, tf.constant([0, 0, 1])).numpy()
+ *
{@literal @}tf.function(jit_compile=True)
+ * ... def test(c):
+ * ... return tf.raw_ops.SegmentProdV2(data=c, segment_ids=tf.constant([0, 0, 1]), num_segments=2)
+ * c = tf.constant([[1,2,3,4], [4, 3, 2, 1], [5,6,7,8]])
+ * test(c).numpy()
* array([[4, 6, 6, 4],
* [5, 6, 7, 8]], dtype=int32)
*
@@ -1886,14 +1919,16 @@ public SegmentMin segmentMin(Operand data,
* @param data The data value
* @param segmentIds A 1-D tensor whose size is equal to the size of {@code data}'s
* first dimension. Values should be sorted and can be repeated.
+ * The values must be less than {@code num_segments}.
* Caution: The values are always validated to be sorted on CPU, never validated
* on GPU.
- * @param data type for {@code SegmentProd} output and operands
+ * @param numSegments The numSegments value
+ * @param data type for {@code SegmentProdV2} output and operands
* @return a new instance of SegmentProd
*/
public SegmentProd segmentProd(Operand data,
- Operand extends TNumber> segmentIds) {
- return SegmentProd.create(scope, data, segmentIds);
+ Operand extends TNumber> segmentIds, Operand extends TNumber> numSegments) {
+ return SegmentProd.create(scope, data, segmentIds, numSegments);
}
/**
@@ -1905,38 +1940,23 @@ public SegmentProd segmentProd(Operand data,
* \(output_i = \sum_j data_j\) where sum is over {@code j} such
* that {@code segment_ids[j] == i}.
* If the sum is empty for a given segment ID {@code i}, {@code output[i] = 0}.
- *
Caution: On CPU, values in {@code segment_ids} are always validated to be sorted,
- * and an error is thrown for indices that are not increasing. On GPU, this
- * does not throw an error for unsorted indices. On GPU, out-of-order indices
- * result in safe but unspecified behavior, which may include treating
- * out-of-order indices as the same as a smaller following index.
- *
- *
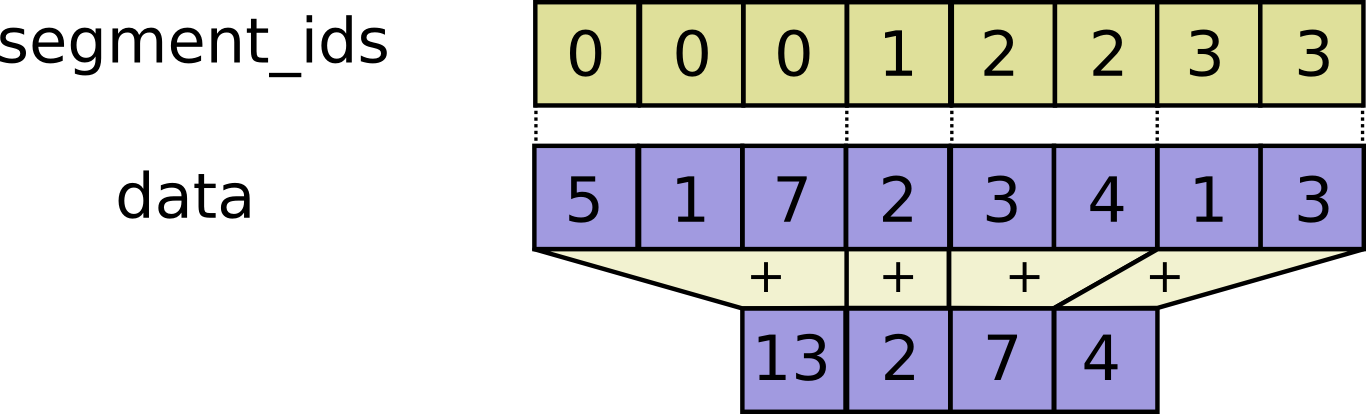
+ *
Note that this op is currently only supported with jit_compile=True.
*
- * For example:
- *
- *
- *
- * c = tf.constant([[1,2,3,4], [4, 3, 2, 1], [5,6,7,8]])
- * tf.math.segment_sum(c, tf.constant([0, 0, 1])).numpy()
- * array([[5, 5, 5, 5],
- * [5, 6, 7, 8]], dtype=int32)
- *
- *
- *
*
* @param data type for {@code output} output
* @param data The data value
* @param segmentIds A 1-D tensor whose size is equal to the size of {@code data}'s
* first dimension. Values should be sorted and can be repeated.
+ * The values must be less than {@code num_segments}.
* Caution: The values are always validated to be sorted on CPU, never validated
* on GPU.
- * @param data type for {@code SegmentSum} output and operands
+ * @param numSegments The numSegments value
+ * @param data type for {@code SegmentSumV2} output and operands
* @return a new instance of SegmentSum
*/
public SegmentSum segmentSum(Operand data,
- Operand extends TNumber> segmentIds) {
- return SegmentSum.create(scope, data, segmentIds);
+ Operand extends TNumber> segmentIds, Operand extends TNumber> numSegments) {
+ return SegmentSum.create(scope, data, segmentIds, numSegments);
}
/**
@@ -2128,7 +2148,7 @@ public Tanh tanh(Operand x) {
}
/**
- * Returns x / y element-wise for integer types.
+ * Returns x / y element-wise, rounded towards zero.
* Truncation designates that negative numbers will round fractional quantities
* toward zero. I.e. -7 / 5 = -1. This matches C semantics but it is different
* than Python semantics. See {@code FloorDiv} for a division function that matches
diff --git a/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/NnOps.java b/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/NnOps.java
index 4b0902ac98f..7ac1a318348 100644
--- a/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/NnOps.java
+++ b/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/NnOps.java
@@ -27,6 +27,7 @@
import org.tensorflow.op.nn.BiasAdd;
import org.tensorflow.op.nn.BiasAddGrad;
import org.tensorflow.op.nn.ComputeAccidentalHits;
+import org.tensorflow.op.nn.Conv;
import org.tensorflow.op.nn.Conv2d;
import org.tensorflow.op.nn.Conv2dBackpropFilter;
import org.tensorflow.op.nn.Conv2dBackpropInput;
@@ -281,6 +282,33 @@ public ComputeAccidentalHits computeAccidentalHits(Operand trueClasses,
return ComputeAccidentalHits.create(scope, trueClasses, sampledCandidates, numTrue, options);
}
+ /**
+ * Computes a N-D convolution given (N+1+batch_dims)-D {@code input} and (N+2)-D {@code filter} tensors.
+ * General function for computing a N-D convolution. It is required that
+ * {@code 1 <= N <= 3}.
+ *
+ * @param data type for {@code output} output
+ * @param input Tensor of type T and shape {@code batch_shape + spatial_shape + [in_channels]} in the
+ * case that {@code channels_last_format = true} or shape
+ * {@code batch_shape + [in_channels] + spatial_shape} if {@code channels_last_format = false}.
+ * spatial_shape is N-dimensional with {@code N=2} or {@code N=3}.
+ * Also note that {@code batch_shape} is dictated by the parameter {@code batch_dims}
+ * and defaults to 1.
+ * @param filter An {@code (N+2)-D} Tensor with the same type as {@code input} and shape
+ * {@code spatial_filter_shape + [in_channels, out_channels]}, where spatial_filter_shape
+ * is N-dimensional with {@code N=2} or {@code N=3}.
+ * @param strides 1-D tensor of length {@code N+2}. The stride of the sliding window for each
+ * dimension of {@code input}. Must have {@code strides[0] = strides[N+1] = 1}.
+ * @param padding The type of padding algorithm to use.
+ * @param options carries optional attribute values
+ * @param data type for {@code Conv} output and operands
+ * @return a new instance of Conv
+ */
+ public Conv conv(Operand input, Operand filter, List strides,
+ String padding, Conv.Options... options) {
+ return Conv.create(scope, input, filter, strides, padding, options);
+ }
+
/**
* Computes a 2-D convolution given 4-D {@code input} and {@code filter} tensors.
* Given an input tensor of shape {@code [batch, in_height, in_width, in_channels]}
@@ -2070,18 +2098,47 @@ public SparseSoftmaxCrossEntropyWithLogits sparseSoftmaxC
* If two elements are equal, the lower-index element appears first.
*
* @param data type for {@code values} output
+ * @param data type for {@code indices} output
* @param input 1-D or higher with last dimension at least {@code k}.
* @param k 0-D. Number of top elements to look for along the last dimension (along each
* row for matrices).
* @param options carries optional attribute values
* @param data type for {@code TopKV2} output and operands
- * @return a new instance of TopK
+ * @return a new instance of TopK, with default output types
*/
- public TopK topK(Operand input, Operand k,
- TopK.Options... options) {
+ public TopK topK(Operand input, Operand extends TNumber> k,
+ TopK.Options[] options) {
return TopK.create(scope, input, k, options);
}
+ /**
+ * Finds values and indices of the {@code k} largest elements for the last dimension.
+ * If the input is a vector (rank-1), finds the {@code k} largest entries in the vector
+ * and outputs their values and indices as vectors. Thus {@code values[j]} is the
+ * {@code j}-th largest entry in {@code input}, and its index is {@code indices[j]}.
+ * For matrices (resp. higher rank input), computes the top {@code k} entries in each
+ * row (resp. vector along the last dimension). Thus,
+ *
+ * values.shape = indices.shape = input.shape[:-1] + [k]
+ *
+ * If two elements are equal, the lower-index element appears first.
+ *
+ * @param data type for {@code values} output
+ * @param data type for {@code indices} output
+ * @param input 1-D or higher with last dimension at least {@code k}.
+ * @param k 0-D. Number of top elements to look for along the last dimension (along each
+ * row for matrices).
+ * @param indexType The value of the indexType attribute
+ * @param options carries optional attribute values
+ * @param data type for {@code TopKV2} output and operands
+ * @param data type for {@code TopKV2} output and operands
+ * @return a new instance of TopK
+ */
+ public TopK topK(Operand input,
+ Operand extends TNumber> k, Class indexType, TopK.Options... options) {
+ return TopK.create(scope, input, k, indexType, options);
+ }
+
/**
* Get the parent {@link Ops} object.
*/
diff --git a/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/Ops.java b/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/Ops.java
index 37f7aa35358..5dd7f842b47 100644
--- a/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/Ops.java
+++ b/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/Ops.java
@@ -77,6 +77,7 @@
import org.tensorflow.op.core.ConsumeMutexLock;
import org.tensorflow.op.core.ControlTrigger;
import org.tensorflow.op.core.CopyToMesh;
+import org.tensorflow.op.core.CopyToMeshGrad;
import org.tensorflow.op.core.CountUpTo;
import org.tensorflow.op.core.DecodeProto;
import org.tensorflow.op.core.DeepCopy;
@@ -176,6 +177,7 @@
import org.tensorflow.op.core.RefSelect;
import org.tensorflow.op.core.RefSwitch;
import org.tensorflow.op.core.Relayout;
+import org.tensorflow.op.core.RelayoutLike;
import org.tensorflow.op.core.RemoteCall;
import org.tensorflow.op.core.Reshape;
import org.tensorflow.op.core.ResourceCountUpTo;
@@ -231,7 +233,6 @@
import org.tensorflow.op.core.StatefulPartitionedCall;
import org.tensorflow.op.core.StatefulWhile;
import org.tensorflow.op.core.StatelessIf;
-import org.tensorflow.op.core.StatelessPartitionedCall;
import org.tensorflow.op.core.StatelessWhile;
import org.tensorflow.op.core.StopGradient;
import org.tensorflow.op.core.StridedSlice;
@@ -381,10 +382,10 @@ public final class Ops {
public final SignalOps signal;
- public final QuantizationOps quantization;
-
public final TrainOps train;
+ public final QuantizationOps quantization;
+
private final Scope scope;
Ops(Scope scope) {
@@ -407,8 +408,8 @@ public final class Ops {
math = new MathOps(this);
audio = new AudioOps(this);
signal = new SignalOps(this);
- quantization = new QuantizationOps(this);
train = new TrainOps(this);
+ quantization = new QuantizationOps(this);
}
/**
@@ -1062,7 +1063,9 @@ public BatchToSpaceNd batchToSpaceNd(Operand input,
*
*
* NOTE: Bitcast is implemented as a low-level cast, so machines with different
- * endian orderings will give different results.
+ * endian orderings will give different results. A copy from input buffer to output
+ * buffer is made on BE machines when types are of different sizes in order to get
+ * the same casting results as on LE machines.
*
* @param data type for {@code output} output
* @param input The input value
@@ -2087,14 +2090,26 @@ public ControlTrigger controlTrigger() {
*
* @param data type for {@code output} output
* @param input The input value
- * @param layout The value of the layout attribute
- * @param options carries optional attribute values
+ * @param mesh The value of the mesh attribute
* @param data type for {@code CopyToMesh} output and operands
* @return a new instance of CopyToMesh
*/
- public CopyToMesh copyToMesh(Operand input, String layout,
- CopyToMesh.Options... options) {
- return CopyToMesh.create(scope, input, layout, options);
+ public CopyToMesh copyToMesh(Operand input, String mesh) {
+ return CopyToMesh.create(scope, input, mesh);
+ }
+
+ /**
+ * The CopyToMeshGrad operation
+ *
+ * @param data type for {@code output} output
+ * @param input The input value
+ * @param forwardInput The forwardInput value
+ * @param data type for {@code CopyToMeshGrad} output and operands
+ * @return a new instance of CopyToMeshGrad
+ */
+ public CopyToMeshGrad copyToMeshGrad(Operand input,
+ Operand forwardInput) {
+ return CopyToMeshGrad.create(scope, input, forwardInput);
}
/**
@@ -2282,6 +2297,15 @@ public DestroyTemporaryVariable destroyTemporaryVariable(Op
*
*
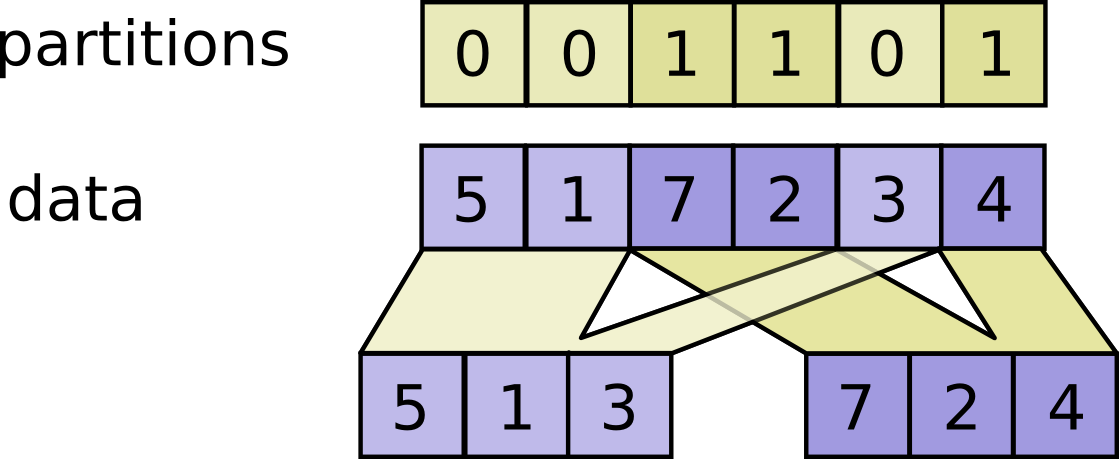
*
+ * Raises:
+ *
+ * - {@code InvalidArgumentError} in following cases:
+ *
+ * - If partitions is not in range {@code [0, num_partiions)}
+ * - If {@code partitions.shape} does not match prefix of {@code data.shape} argument.
+ *
+ *
+ *
*
* @param data type for {@code outputs} output
* @param data The data value
@@ -4009,8 +4033,9 @@ public ParallelDynamicStitch parallelDynamicStitch(
/**
* returns {@code f(inputs)}, where {@code f}'s body is placed and partitioned.
- *
- * Selects between {@link StatefulPartitionedCall} and {@link StatelessPartitionedCall} based on the statefulness of the function arguments.
+ * Asynchronously executes a function, potentially across multiple devices but
+ * within a single process. The kernel places and partitions a given function's
+ * underlying graph, and executes each of the partitioned subgraphs as a function.
*
* @param args A list of input tensors.
* @param Tout A list of output types.
@@ -4018,8 +4043,7 @@ public ParallelDynamicStitch parallelDynamicStitch(
* A function that takes 'args', a list of tensors, and returns 'output',
* another list of tensors. Input and output types are specified by 'Tin'
* and 'Tout'. The function body of f will be placed and partitioned across
- * devices, setting this op apart from the regular Call op. This op is
- * stateful.
+ * devices, setting this op apart from the regular Call op.
*
* @param options carries optional attribute values
* @return a new instance of PartitionedCall
@@ -4119,12 +4143,13 @@ public QuantizedReshape quantizedReshape(Operand tensor,
* @param index A scalar tensor or a vector of dtype {@code dtype}. The index (or indices) to be shuffled. Must be within [0, max_index].
* @param seed A tensor of dtype {@code Tseed} and shape [3] or [n, 3]. The random seed.
* @param maxIndex A scalar tensor or vector of dtype {@code dtype}. The upper bound(s) of the interval (inclusive).
+ * @param options carries optional attribute values
* @param data type for {@code RandomIndexShuffle} output and operands
* @return a new instance of RandomIndexShuffle
*/
public RandomIndexShuffle randomIndexShuffle(Operand index,
- Operand extends TNumber> seed, Operand maxIndex) {
- return RandomIndexShuffle.create(scope, index, seed, maxIndex);
+ Operand extends TNumber> seed, Operand maxIndex, RandomIndexShuffle.Options... options) {
+ return RandomIndexShuffle.create(scope, index, seed, maxIndex, options);
}
/**
@@ -4360,6 +4385,20 @@ public Relayout relayout(Operand input, String layout) {
return Relayout.create(scope, input, layout);
}
+ /**
+ * The RelayoutLike operation
+ *
+ * @param data type for {@code output} output
+ * @param input The input value
+ * @param layoutInput The layoutInput value
+ * @param data type for {@code RelayoutLike} output and operands
+ * @return a new instance of RelayoutLike
+ */
+ public RelayoutLike relayoutLike(Operand input,
+ Operand extends TType> layoutInput) {
+ return RelayoutLike.create(scope, input, layoutInput);
+ }
+
/**
* Runs function {@code f} on a remote device indicated by {@code target}.
*
@@ -5275,7 +5314,7 @@ public ScatterMul scatterMul(Operand ref,
*
* In Python, this scatter operation would look like this:
*
- * indices = tf.constant([[0], [2]])
+ * indices = tf.constant([[1], [3]])
* updates = tf.constant([[[5, 5, 5, 5], [6, 6, 6, 6],
* [7, 7, 7, 7], [8, 8, 8, 8]],
* [[5, 5, 5, 5], [6, 6, 6, 6],
@@ -5286,10 +5325,10 @@ public ScatterMul scatterMul(Operand ref,
*
* The resulting tensor would look like this:
*
- * [[[5, 5, 5, 5], [6, 6, 6, 6], [7, 7, 7, 7], [8, 8, 8, 8]],
- * [[0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]],
+ * [[[0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]],
* [[5, 5, 5, 5], [6, 6, 6, 6], [7, 7, 7, 7], [8, 8, 8, 8]],
- * [[0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]]]
+ * [[0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]],
+ * [[5, 5, 5, 5], [6, 6, 6, 6], [7, 7, 7, 7], [8, 8, 8, 8]]]
*
* Note that on CPU, if an out of bound index is found, an error is returned.
* On GPU, if an out of bound index is found, the index is ignored.
@@ -5638,7 +5677,8 @@ public SetDiff1d setDiff1d(Operand
* and {@code set_shape}. The last dimension contains values in a set, duplicates are
* allowed but ignored.
* If {@code validate_indices} is {@code True}, this op validates the order and range of {@code set}
- * indices.
+ * indices. Setting is to {@code False} while passing invalid arguments results in
+ * undefined behavior.
*
* @param setIndices 2D {@code Tensor}, indices of a {@code SparseTensor}.
* @param setValues 1D {@code Tensor}, values of a {@code SparseTensor}.
@@ -6141,7 +6181,8 @@ public StatefulIf statefulIf(Operand extends TType> cond, Iterable>
* @return a new instance of StatefulPartitionedCall
*/
public StatefulPartitionedCall statefulPartitionedCall(Iterable> args,
- List> Tout, ConcreteFunction f, PartitionedCall.Options... options) {
+ List> Tout, ConcreteFunction f,
+ StatefulPartitionedCall.Options... options) {
return StatefulPartitionedCall.create(scope, args, Tout, f, options);
}
@@ -6204,28 +6245,6 @@ public StatelessIf statelessIf(Operand extends TType> cond, Iterable
- * A function that takes 'args', a list of tensors, and returns 'output',
- * another list of tensors. Input and output types are specified by 'Tin'
- * and 'Tout'. The function body of f will be placed and partitioned across
- * devices, setting this op apart from the regular Call op.
- *
- * @param options carries optional attribute values
- * @return a new instance of StatelessPartitionedCall
- */
- public StatelessPartitionedCall statelessPartitionedCall(Iterable> args,
- List> Tout, ConcreteFunction f, PartitionedCall.Options... options) {
- return StatelessPartitionedCall.create(scope, args, Tout, f, options);
- }
-
/**
* output = input; While (Cond(output)) { output = Body(output) }
*
@@ -6665,7 +6684,9 @@ public TensorArrayClose tensorArrayClose(Operand extends TType> handle) {
* (n0 x d0 x d1 x ...), (n1 x d0 x d1 x ...), ..., (n(T-1) x d0 x d1 x ...)
*
* and concatenates them into a Tensor of shape:
- *
{@code (n0 + n1 + ... + n(T-1) x d0 x d1 x ...)}
+ *
+ * (n0 + n1 + ... + n(T-1) x d0 x d1 x ...)
+ *
* All elements must have the same shape (excepting the first dimension).
*
* @param data type for {@code value} output
@@ -6825,14 +6846,22 @@ public TensorArraySize tensorArraySize(Operand extends TType> handle,
/**
* Split the data from the input value into TensorArray elements.
* Assuming that {@code lengths} takes on values
- * {@code (n0, n1, ..., n(T-1))}
+ *
+ * (n0, n1, ..., n(T-1))
+ *
* and that {@code value} has shape
- *
{@code (n0 + n1 + ... + n(T-1) x d0 x d1 x ...)},
+ *
+ * (n0 + n1 + ... + n(T-1) x d0 x d1 x ...),
+ *
* this splits values into a TensorArray with T tensors.
*
TensorArray index t will be the subtensor of values with starting position
- *
{@code (n0 + n1 + ... + n(t-1), 0, 0, ...)}
+ *
+ * (n0 + n1 + ... + n(t-1), 0, 0, ...)
+ *
* and having size
- *
{@code nt x d0 x d1 x ...}
+ *
+ * nt x d0 x d1 x ...
+ *
*
* @param handle The handle to a TensorArray.
* @param value The concatenated tensor to write to the TensorArray.
@@ -6968,7 +6997,10 @@ public TensorListGather tensorListGather(
}
/**
- * The TensorListGetItem operation
+ * Returns the item in the list with the given index.
+ * input_handle: the list
+ * index: the position in the list from which an element will be retrieved
+ * item: the element at that position
*
* @param data type for {@code item} output
* @param inputHandle The inputHandle value
@@ -7123,16 +7155,21 @@ public TensorListScatterIntoExistingList tensorListScatterIntoExistingList(
}
/**
- * The TensorListSetItem operation
+ * Sets the index-th position of the list to contain the given tensor.
+ * input_handle: the list
+ * index: the position in the list to which the tensor will be assigned
+ * item: the element to be assigned to that position
+ * output_handle: the new list, with the element in the proper position
*
* @param inputHandle The inputHandle value
* @param index The index value
* @param item The item value
+ * @param options carries optional attribute values
* @return a new instance of TensorListSetItem
*/
public TensorListSetItem tensorListSetItem(Operand extends TType> inputHandle,
- Operand index, Operand extends TType> item) {
- return TensorListSetItem.create(scope, inputHandle, index, item);
+ Operand index, Operand extends TType> item, TensorListSetItem.Options... options) {
+ return TensorListSetItem.create(scope, inputHandle, index, item, options);
}
/**
@@ -7574,8 +7611,16 @@ public Tile tile(Operand input, Operand extends TNumbe
/**
* Provides the time since epoch in seconds.
* Returns the timestamp as a {@code float64} for seconds since the Unix epoch.
- * Note: the timestamp is computed when the op is executed, not when it is added
- * to the graph.
+ *
Common usages include:
+ *
+ * - Logging
+ * - Providing a random number seed
+ * - Debugging graph execution
+ * - Generating timing information, mainly through comparison of timestamps
+ *
+ * Note: In graph mode, the timestamp is computed when the op is executed,
+ * not when it is added to the graph. In eager mode, the timestamp is computed
+ * when the op is eagerly executed.
*
* @return a new instance of Timestamp
*/
@@ -7806,7 +7851,7 @@ public Unique unique(Operand x,
* For example:
*
* x = tf.constant([1, 1, 2, 4, 4, 4, 7, 8, 8])
- * y, idx, count = UniqueWithCountsV2(x, axis = [0])
+ * y, idx, count = tf.raw_ops.UniqueWithCountsV2(x=x, axis = [0])
* y ==> [1, 2, 4, 7, 8]
* idx ==> [0, 0, 1, 2, 2, 2, 3, 4, 4]
* count ==> [2, 1, 3, 1, 2]
@@ -7816,7 +7861,7 @@ public Unique unique(Operand x,
* x = tf.constant([[1, 0, 0],
* [1, 0, 0],
* [2, 0, 0]])
- * y, idx, count = UniqueWithCountsV2(x, axis=[0])
+ * y, idx, count = tf.raw_ops.UniqueWithCountsV2(x=x, axis=[0])
* y ==> [[1, 0, 0],
* [2, 0, 0]]
* idx ==> [0, 0, 1]
@@ -7827,7 +7872,7 @@ public Unique unique(Operand x,
* x = tf.constant([[1, 0, 0],
* [1, 0, 0],
* [2, 0, 0]])
- * y, idx, count = UniqueWithCountsV2(x, axis=[1])
+ * y, idx, count = tf.raw_ops.UniqueWithCountsV2(x=x, axis=[1])
* y ==> [[1, 0],
* [1, 0],
* [2, 0]]
@@ -7862,7 +7907,7 @@ public UniqueWithCounts uniqueWithCounts(Operand
* For example:
*
* x = tf.constant([1, 1, 2, 4, 4, 4, 7, 8, 8])
- * y, idx, count = UniqueWithCountsV2(x, axis = [0])
+ * y, idx, count = tf.raw_ops.UniqueWithCountsV2(x=x, axis = [0])
* y ==> [1, 2, 4, 7, 8]
* idx ==> [0, 0, 1, 2, 2, 2, 3, 4, 4]
* count ==> [2, 1, 3, 1, 2]
@@ -7872,7 +7917,7 @@ public UniqueWithCounts uniqueWithCounts(Operand
* x = tf.constant([[1, 0, 0],
* [1, 0, 0],
* [2, 0, 0]])
- * y, idx, count = UniqueWithCountsV2(x, axis=[0])
+ * y, idx, count = tf.raw_ops.UniqueWithCountsV2(x=x, axis=[0])
* y ==> [[1, 0, 0],
* [2, 0, 0]]
* idx ==> [0, 0, 1]
@@ -7883,7 +7928,7 @@ public UniqueWithCounts uniqueWithCounts(Operand
* x = tf.constant([[1, 0, 0],
* [1, 0, 0],
* [2, 0, 0]])
- * y, idx, count = UniqueWithCountsV2(x, axis=[1])
+ * y, idx, count = tf.raw_ops.UniqueWithCountsV2(x=x, axis=[1])
* y ==> [[1, 0],
* [1, 0],
* [2, 0]]
diff --git a/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/QuantizationOps.java b/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/QuantizationOps.java
index ea6c39d28c4..e0302dd0252 100644
--- a/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/QuantizationOps.java
+++ b/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/QuantizationOps.java
@@ -175,8 +175,11 @@ public Dequantize dequantize(Operand extends TNumber> i
}
/**
- * Fake-quantize the 'inputs' tensor, type float to 'outputs' tensor of same type.
- * Attributes
+ * Fake-quantize the 'inputs' tensor, type float to 'outputs' tensor of same shape and type.
+ * Quantization is called fake since the output is still in floating point.
+ * The API converts inputs into values within the range [min and max] and returns
+ * as output.
+ * Attributes
*
* - {@code [min; max]} define the clamping range for the {@code inputs} data.
* - {@code inputs} values are quantized into the quantization range (
@@ -195,7 +198,27 @@ public Dequantize dequantize(Operand extends TNumber> i
*
- If {@code min <= 0 <= max}: {@code scale = (max - min) / (2^num_bits - 1) },
* {@code min_adj = scale * round(min / scale)} and {@code max_adj = max + min_adj - min}.
*
- * Quantization is called fake since the output is still in floating point.
+ *
Examples
+ *
+ *
+ * inp = tf.constant ([10.03, -10.23, 3])
+ * out = tf.quantization.fake_quant_with_min_max_args(inp, min=-5, max=5,
+ * num_bits=16)
+ * print(out)
+ *
+ * # Output:
+ * # tf.Tensor([ 4.9999237 -5.0000763 3.0000763], shape=(3,), dtype=float32)
+ *
+ * Raises:
+ *
+ * - InvalidArgumentError:
+ *
+ * - If num_bits are outside of range [2, 16].
+ * - If min >= max.
+ *
+ *
+ * - ValueError: If {@code inputs} are of any other type than float32.
+ *
*
* @param inputs The inputs value
* @param options carries optional attribute values
diff --git a/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/RaggedOps.java b/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/RaggedOps.java
index 04aed43f2a2..a1cd7bbdc4b 100644
--- a/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/RaggedOps.java
+++ b/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/RaggedOps.java
@@ -19,8 +19,11 @@
import org.tensorflow.Operand;
import org.tensorflow.op.ragged.RaggedBincount;
+import org.tensorflow.op.ragged.RaggedFillEmptyRows;
+import org.tensorflow.op.ragged.RaggedFillEmptyRowsGrad;
import org.tensorflow.types.TInt64;
import org.tensorflow.types.family.TNumber;
+import org.tensorflow.types.family.TType;
/**
* An API for building {@code ragged} operations as {@link Op Op}s
@@ -64,6 +67,36 @@ public RaggedBincount raggedBincount(
return RaggedBincount.create(scope, splits, values, sizeOutput, weights, options);
}
+ /**
+ * The RaggedFillEmptyRows operation
+ *
+ * @param data type for {@code output_values} output
+ * @param valueRowids The valueRowids value
+ * @param values The values value
+ * @param nrows The nrows value
+ * @param defaultValue The defaultValue value
+ * @param data type for {@code RaggedFillEmptyRows} output and operands
+ * @return a new instance of RaggedFillEmptyRows
+ */
+ public RaggedFillEmptyRows raggedFillEmptyRows(Operand valueRowids,
+ Operand values, Operand nrows, Operand defaultValue) {
+ return RaggedFillEmptyRows.create(scope, valueRowids, values, nrows, defaultValue);
+ }
+
+ /**
+ * The RaggedFillEmptyRowsGrad operation
+ *
+ * @param data type for {@code d_values} output
+ * @param reverseIndexMap The reverseIndexMap value
+ * @param gradValues The gradValues value
+ * @param data type for {@code RaggedFillEmptyRowsGrad} output and operands
+ * @return a new instance of RaggedFillEmptyRowsGrad
+ */
+ public RaggedFillEmptyRowsGrad raggedFillEmptyRowsGrad(
+ Operand reverseIndexMap, Operand gradValues) {
+ return RaggedFillEmptyRowsGrad.create(scope, reverseIndexMap, gradValues);
+ }
+
/**
* Get the parent {@link Ops} object.
*/
diff --git a/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/SignalOps.java b/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/SignalOps.java
index 43866adaa9b..0fb3e8edde0 100644
--- a/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/SignalOps.java
+++ b/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/SignalOps.java
@@ -27,15 +27,19 @@
import org.tensorflow.op.signal.Fft;
import org.tensorflow.op.signal.Fft2d;
import org.tensorflow.op.signal.Fft3d;
+import org.tensorflow.op.signal.FftNd;
import org.tensorflow.op.signal.Ifft;
import org.tensorflow.op.signal.Ifft2d;
import org.tensorflow.op.signal.Ifft3d;
+import org.tensorflow.op.signal.IfftNd;
import org.tensorflow.op.signal.Irfft;
import org.tensorflow.op.signal.Irfft2d;
import org.tensorflow.op.signal.Irfft3d;
+import org.tensorflow.op.signal.IrfftNd;
import org.tensorflow.op.signal.Rfft;
import org.tensorflow.op.signal.Rfft2d;
import org.tensorflow.op.signal.Rfft3d;
+import org.tensorflow.op.signal.RfftNd;
import org.tensorflow.types.TFloat32;
import org.tensorflow.types.TInt32;
import org.tensorflow.types.family.TNumber;
@@ -158,6 +162,29 @@ public Fft3d fft3d(Operand input) {
return Fft3d.create(scope, input);
}
+ /**
+ * ND fast Fourier transform.
+ * Computes the n-dimensional discrete Fourier transform over
+ * designated dimensions of {@code input}. The designated dimensions of
+ * {@code input} are assumed to be the result of {@code signal.FftNd}.
+ * If fft_length[i]<shape(input)[i], the input is cropped. If
+ * fft_length[i]>shape(input)[i], the input is padded with zeros. If fft_length
+ * is not given, the default shape(input) is used.
+ *
Axes mean the dimensions to perform the transform on. Default is to perform on
+ * all axes.
+ *
+ * @param data type for {@code output} output
+ * @param input A complex tensor.
+ * @param fftLength An int32 tensor. The FFT length for each dimension.
+ * @param axes An int32 tensor with a same shape as fft_length. Axes to perform the transform.
+ * @param data type for {@code FFTND} output and operands
+ * @return a new instance of FftNd
+ */
+ public FftNd fftNd(Operand input, Operand fftLength,
+ Operand axes) {
+ return FftNd.create(scope, input, fftLength, axes);
+ }
+
/**
* Inverse fast Fourier transform.
* Computes the inverse 1-dimensional discrete Fourier transform over the
@@ -200,6 +227,29 @@ public Ifft3d ifft3d(Operand input) {
return Ifft3d.create(scope, input);
}
+ /**
+ * ND inverse fast Fourier transform.
+ * Computes the n-dimensional inverse discrete Fourier transform over designated
+ * dimensions of {@code input}. The designated dimensions of {@code input} are assumed to be
+ * the result of {@code signal.IfftNd}.
+ * If fft_length[i]<shape(input)[i], the input is cropped. If
+ * fft_length[i]>shape(input)[i], the input is padded with zeros. If fft_length
+ * is not given, the default shape(input) is used.
+ *
Axes mean the dimensions to perform the transform on. Default is to perform on
+ * all axes.
+ *
+ * @param data type for {@code output} output
+ * @param input A complex tensor.
+ * @param fftLength An int32 tensor. The FFT length for each dimension.
+ * @param axes An int32 tensor with a same shape as fft_length. Axes to perform the transform.
+ * @param data type for {@code IFFTND} output and operands
+ * @return a new instance of IfftNd
+ */
+ public IfftNd ifftNd(Operand input, Operand fftLength,
+ Operand axes) {
+ return IfftNd.create(scope, input, fftLength, axes);
+ }
+
/**
* Inverse real-valued fast Fourier transform.
* Computes the inverse 1-dimensional discrete Fourier transform of a real-valued
@@ -351,6 +401,54 @@ public Irfft3d irfft3d(Operand extends TType> input,
return Irfft3d.create(scope, input, fftLength, Treal);
}
+ /**
+ * ND inverse real fast Fourier transform.
+ * Computes the n-dimensional inverse real discrete Fourier transform over
+ * designated dimensions of {@code input}. The designated dimensions of {@code input} are
+ * assumed to be the result of {@code signal.IrfftNd}. The inner-most dimension contains the
+ * {@code fft_length / 2 + 1} unique components of the DFT of a real-valued signal.
+ * If fft_length[i]<shape(input)[i], the input is cropped. If
+ * fft_length[i]>shape(input)[i], the input is padded with zeros. If fft_length
+ * is not given, the default shape(input) is used.
+ *
Axes mean the dimensions to perform the transform on. Default is to perform on
+ * all axes.
+ *
+ * @param data type for {@code output} output
+ * @param input A complex tensor.
+ * @param fftLength An int32 tensor. The FFT length for each dimension.
+ * @param axes An int32 tensor with a same shape as fft_length. Axes to perform the transform.
+ * @return a new instance of IrfftNd, with default output types
+ */
+ public IrfftNd irfftNd(Operand extends TType> input, Operand fftLength,
+ Operand axes) {
+ return IrfftNd.create(scope, input, fftLength, axes);
+ }
+
+ /**
+ * ND inverse real fast Fourier transform.
+ * Computes the n-dimensional inverse real discrete Fourier transform over
+ * designated dimensions of {@code input}. The designated dimensions of {@code input} are
+ * assumed to be the result of {@code signal.IrfftNd}. The inner-most dimension contains the
+ * {@code fft_length / 2 + 1} unique components of the DFT of a real-valued signal.
+ * If fft_length[i]<shape(input)[i], the input is cropped. If
+ * fft_length[i]>shape(input)[i], the input is padded with zeros. If fft_length
+ * is not given, the default shape(input) is used.
+ *
Axes mean the dimensions to perform the transform on. Default is to perform on
+ * all axes.
+ *
+ * @param data type for {@code output} output
+ * @param input A complex tensor.
+ * @param fftLength An int32 tensor. The FFT length for each dimension.
+ * @param axes An int32 tensor with a same shape as fft_length. Axes to perform the transform.
+ * @param Treal The value of the Treal attribute
+ * @param data type for {@code IRFFTND} output and operands
+ * @return a new instance of IrfftNd
+ */
+ public IrfftNd irfftNd(Operand extends TType> input,
+ Operand fftLength, Operand axes, Class Treal) {
+ return IrfftNd.create(scope, input, fftLength, axes, Treal);
+ }
+
/**
* Real-valued fast Fourier transform.
* Computes the 1-dimensional discrete Fourier transform of a real-valued signal
@@ -422,6 +520,31 @@ public Rfft3d rfft3d(Operand extends TNumber> input,
return Rfft3d.create(scope, input, fftLength, Tcomplex);
}
+ /**
+ * ND fast real Fourier transform.
+ * Computes the n-dimensional real discrete Fourier transform over designated
+ * dimensions of {@code input}. The designated dimensions of {@code input} are assumed to be
+ * the result of {@code signal.RfftNd}. The length of the last axis transformed will be
+ * fft_length[-1]//2+1.
+ * If fft_length[i]<shape(input)[i], the input is cropped. If
+ * fft_length[i]>shape(input)[i], the input is padded with zeros. If fft_length
+ * is not given, the default shape(input) is used.
+ *
Axes mean the dimensions to perform the transform on. Default is to perform on
+ * all axes.
+ *
+ * @param data type for {@code output} output
+ * @param input A complex tensor.
+ * @param fftLength An int32 tensor. The FFT length for each dimension.
+ * @param axes An int32 tensor with a same shape as fft_length. Axes to perform the transform.
+ * @param Tcomplex The value of the Tcomplex attribute
+ * @param data type for {@code RFFTND} output and operands
+ * @return a new instance of RfftNd
+ */
+ public RfftNd rfftNd(Operand extends TNumber> input,
+ Operand fftLength, Operand axes, Class Tcomplex) {
+ return RfftNd.create(scope, input, fftLength, axes, Tcomplex);
+ }
+
/**
* Get the parent {@link Ops} object.
*/
diff --git a/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/SparseOps.java b/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/SparseOps.java
index ee27d62406b..c2b253fe29e 100644
--- a/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/SparseOps.java
+++ b/tensorflow-core/tensorflow-core-api/src/gen/annotations/org/tensorflow/op/SparseOps.java
@@ -905,31 +905,36 @@ public SparseReshape sparseReshape(Operand inputIndices, Operand
* @param data The data value
* @param indices A 1-D tensor. Has same rank as {@code segment_ids}.
* @param segmentIds A 1-D tensor. Values should be sorted and can be repeated.
+ * @param options carries optional attribute values
* @param data type for {@code SparseSegmentMean} output and operands
* @return a new instance of SparseSegmentMean
*/
public SparseSegmentMean sparseSegmentMean(Operand