|
| 1 | +# Welcome to Sprig! |
| 2 | + |
| 3 | +## READ ME FIRST |
| 4 | + |
| 5 | +**After each edit, hit the "Run" button in the top right of the editor to see your changes.** |
| 6 | +*P.S.: you can also use the `shift + enter` shortcut.* |
| 7 | + |
| 8 | +THIS CODE HAS INTENTIONAL ERRORS. |
| 9 | + |
| 10 | +To complete each step you'll have to edit the code. |
| 11 | + |
| 12 | +The code for this game starts below this comment. |
| 13 | + |
| 14 | +Made based on this workshop https://workshops.hackclub.com/sprig_dodge/ |
| 15 | + |
| 16 | +The goal is to dodge the fireballs. |
| 17 | + |
| 18 | +## Step 1: Add the player to the map |
| 19 | + |
| 20 | +The basic thing is to have a player on the screen, right? So let's go! Click on the map and add the player sprite to a tile. |
| 21 | + |
| 22 | +<details> |
| 23 | +<summary>Stuck? Show Hint.</summary> |
| 24 | + |
| 25 | +Scroll through the code to find `setMap`. |
| 26 | +</details> |
| 27 | +<details> |
| 28 | +<summary>I've tried my best. Show Solution.</summary> |
| 29 | + |
| 30 | +Sprig creates [tile-based games](https://en.wikipedia.org/wiki/Tile-based_game), uses structures like `bitmap` and `map` to make it. |
| 31 | + |
| 32 | +`bitmap` is 1 tile and `map` is many tiles that `bitmap` can move. |
| 33 | + |
| 34 | +`bitmap` don't appear out of nowhere, need to be added to the `map`, to do click on `map`, select player `bitmap` and place on a tile: |
| 35 | + |
| 36 | +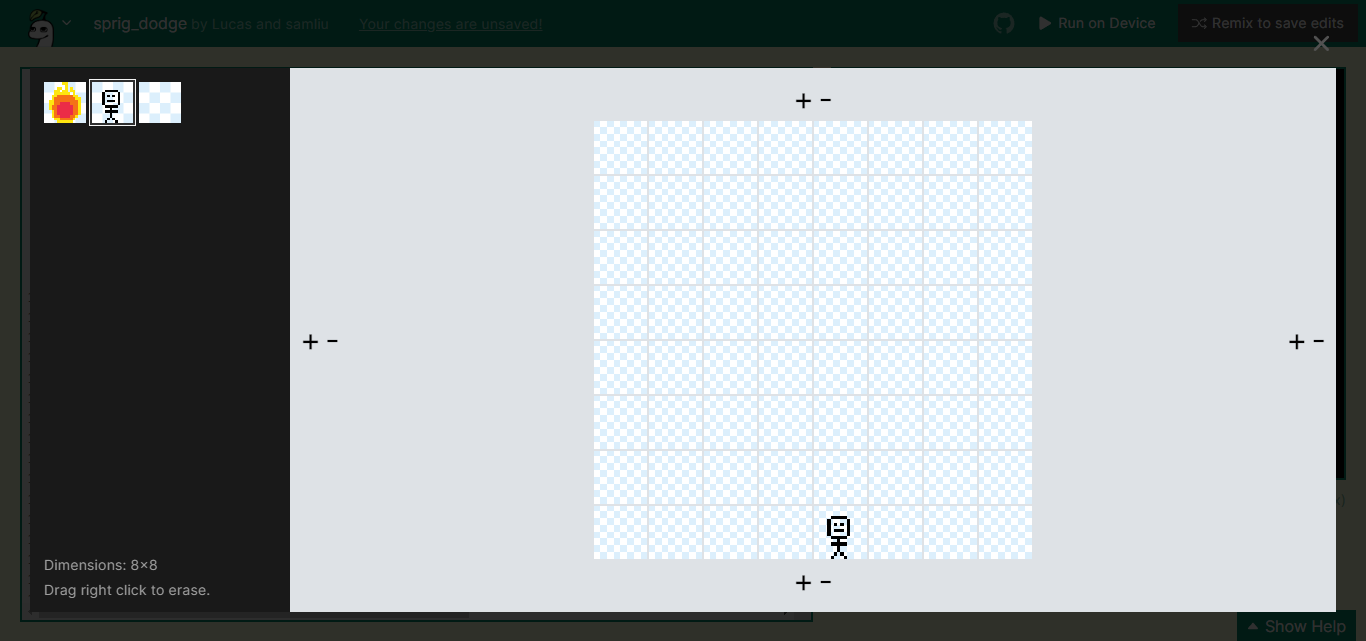 |
| 37 | + |
| 38 | +</details> |
| 39 | + |
| 40 | +## Step 2: Add controls for moving the player to right |
| 41 | + |
| 42 | +Try moving the character around with the `a` and `d` keys on your keyboard. You'll notice that the player can only move left (when you press `a`). |
| 43 | + |
| 44 | +Your challenge is to add control for the player to move right, use `d` as input. |
| 45 | + |
| 46 | +<details> |
| 47 | +<summary>Stuck? Show Hint.</summary> |
| 48 | + |
| 49 | +Scroll through the code to find `onInput`. |
| 50 | +</details> |
| 51 | + |
| 52 | +<details> |
| 53 | +<summary>I've tried my best. Show Solution.</summary> |
| 54 | + |
| 55 | +In JavaScript, a [function](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Functions) is a block of code designed to do a specific task. In Sprig, an `onInput` function is used to detect when a keyboard input is given. In our code, we can see that there are two `onInput` functions for the keys `a` and `d`. |
| 56 | + |
| 57 | +We'll need to add one more for the key `d`. Type this out below your `onInput` functions for `d`. |
| 58 | + |
| 59 | +```js |
| 60 | +onInput("d", function() { |
| 61 | + getFirst(player).x += 1; |
| 62 | +}); |
| 63 | +``` |
| 64 | +</details> |
| 65 | + |
| 66 | +## Step 3: Fix the code! |
| 67 | + |
| 68 | +If you have already clicked on `Run` button, you have already noticed that this error appears: |
| 69 | + |
| 70 | +``` |
| 71 | +Uncaught TypeError: Cannot read properties of undefined (reading 'length') on line ... in column 33. Open the browser console for more information. |
| 72 | +``` |
| 73 | + |
| 74 | +<details> |
| 75 | +<summary>Stuck? Show Hint.</summary> |
| 76 | + |
| 77 | +the `getAll()` and `getFirst()` functions are a bit strange, aren't they? |
| 78 | + |
| 79 | +</details> |
| 80 | + |
| 81 | +<details> |
| 82 | +<summary>I've tried my best. Show Solution.</summary> |
| 83 | + |
| 84 | +This is a great example of when the error itself isn't in the line that says error. |
| 85 | + |
| 86 | +If you look at `getFirst()` and `getAll()` and knowing what each one is used for: |
| 87 | + |
| 88 | +``` |
| 89 | +getAll(type) |
| 90 | +Returns all sprites of the given type. If no bitmap key is specified, it returns all the sprites in the game. |
| 91 | +
|
| 92 | +getFirst(type) |
| 93 | +Returns the first sprite of a given type. Useful if you know there’s only one of a sprite, such as with a player character. |
| 94 | +``` |
| 95 | + |
| 96 | +you will notice that they are swapped, after all, it wouldn't make sense to use `getFirst()` for some sprite that has several copies on the map. |
| 97 | +</details> |
| 98 | + |
| 99 | +## Step 4: Add all functions in the gameLoop interval |
| 100 | + |
| 101 | +For everything to work, add the functions created in gameLoop. |
| 102 | + |
| 103 | +<details> |
| 104 | +<summary>Stuck? Show Hint.</summary> |
| 105 | + |
| 106 | +Use spawnObstacle(), moveObstacles(), despawnObstacles() and checkHit() |
| 107 | + |
| 108 | +</details> |
| 109 | + |
| 110 | +<details> |
| 111 | +<summary>I've tried my best. Show Solution.</summary> |
| 112 | + |
| 113 | +Just copy the functions in gameLoop:) |
| 114 | + |
| 115 | +```js |
| 116 | +var gameLoop = setInterval(() => { |
| 117 | + despawnObstacles(); |
| 118 | + moveObstacles(); |
| 119 | + spawnObstacle(); |
| 120 | +``` |
| 121 | +
|
| 122 | +</details> |
| 123 | +
|
| 124 | +## Done! |
| 125 | +
|
| 126 | +You’re now a Sprig game developer! You have a few options for how to continue on your Sprig journey: |
| 127 | +
|
| 128 | +If you feel confident with the Sprig editor and ready to create your own games from scratch, |
| 129 | +- Take a look at the [Sprig Gallery](/gallery) and collect ideas from games you look through |
| 130 | +- [Click here](/~/new) to create a new Sprig game |
| 131 | +- Once you're done, [submit](/get) your game to the gallery and get a free Sprig console |
| 132 | +
|
| 133 | +If you're enjoying hacking on this game, here are some ideas for how to expand on it: |
| 134 | + - adding two players |
| 135 | + - adding powerups |
| 136 | + - come up with your own mechanic! |
| 137 | +
|
| 138 | +If you need help, remember that the toolkit (at the top of this panel) is there for you. You can also ask in the `#sprig` channel in the [Hack Club Slack](https://hackclub.com/slack/). |
0 commit comments