|
| 1 | +<!-- This file is compiled from bolt-dev/bolt/dev/README.md. Do not edit this file directly. --> |
| 2 | + |
| 3 | +# bolt-dev |
| 4 | + |
| 5 | +A single command to run everything you need for local Bolt development. |
| 6 | + |
| 7 | +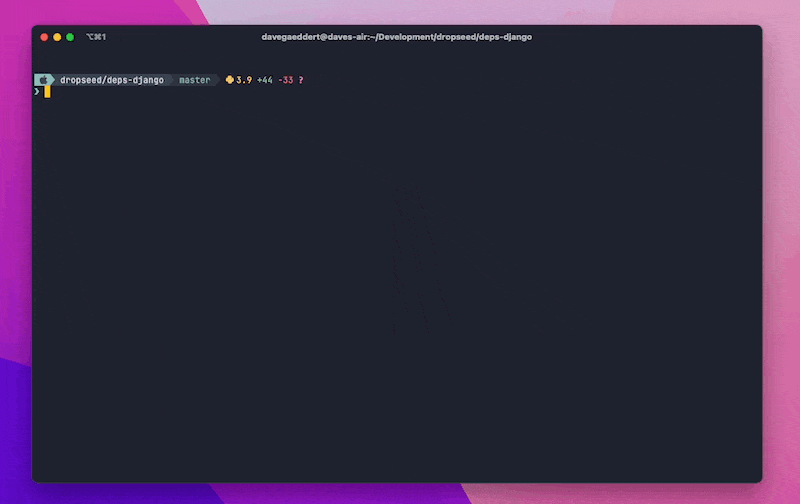 |
| 8 | + |
| 9 | +The `bolt dev` command runs a combination of local commands + a Docker container for your database. |
| 10 | + |
| 11 | +The following processes will run simultaneously (some will only run if they are detected as available): |
| 12 | + |
| 13 | +<!-- - [`manage.py runserver` (and migrations)](#runserver) |
| 14 | +- [`bolt-db start --logs`](#bolt-db) |
| 15 | +- [`bolt-tailwind compile --watch`](#bolt-tailwind) |
| 16 | +- [`npm run watch`](#package-json) |
| 17 | +- [`stripe listen --forward-to`](#stripe) |
| 18 | +- [`ngrok http --subdomain`](#ngrok) |
| 19 | +
|
| 20 | +It also comes with [debugging](#debugging) tools to make local debugging easier with VS Code. --> |
| 21 | + |
| 22 | +## Installation |
| 23 | + |
| 24 | +```sh |
| 25 | +pip install bolt-dev |
| 26 | +``` |
| 27 | + |
| 28 | +If you have `bolt-db` installed (i.e. you're using a database), |
| 29 | +then add `DATABASE_URL` to your `.env` file. |
| 30 | + |
| 31 | +```sh |
| 32 | +DATABASE_URL=postgres://postgres:postgres@localhost:54321/postgres |
| 33 | +``` |
| 34 | + |
| 35 | +```toml |
| 36 | +# pyproject.toml |
| 37 | +[tool.bolt.dev.services] |
| 38 | +postgres = {cmd = "docker run --name app-postgres --rm -p 54321:5432 -v $(pwd)/.bolt/dev/pgdata:/var/lib/postgresql/data -e POSTGRES_PASSWORD=postgres postgres:15 postgres"} |
| 39 | +``` |
| 40 | + |
| 41 | +```sh |
| 42 | +bolt dev |
| 43 | +``` |
| 44 | + |
| 45 | +## `bolt dev` |
| 46 | + |
| 47 | +### Default processes |
| 48 | + |
| 49 | +- bolt preflight |
| 50 | +- gunicorn |
| 51 | +- migrations |
| 52 | +- tailwind |
| 53 | + |
| 54 | +### Custom processes |
| 55 | + |
| 56 | +- package.json "dev" script |
| 57 | +- pyproject.toml `tool.bolt.dev.run = {command = "..."}` |
| 58 | + |
| 59 | +### GitHub Codespaces |
| 60 | + |
| 61 | +The `BASE_URL` setting is automatically set to the Codespace URL. |
| 62 | + |
| 63 | +TODO |
| 64 | + |
| 65 | +## `bolt dev db` |
| 66 | + |
| 67 | +Only supports Postgres currently. |
| 68 | + |
| 69 | +- snapshot |
| 70 | +- import |
| 71 | +- export |
| 72 | + |
| 73 | + |
| 74 | +## Development processes |
| 75 | + |
| 76 | +### Gunicorn |
| 77 | + |
| 78 | +The key process here is still `manage.py runserver`. |
| 79 | +But, before that runs, it will also wait for the database to be available and run `manage.py migrate`. |
| 80 | + |
| 81 | +### bolt-db |
| 82 | + |
| 83 | +If [`bolt-db`](https://github.com/boltpackages/bolt-db) is installed, it will automatically start and show the logs of the running database container. |
| 84 | + |
| 85 | +### bolt-tailwind |
| 86 | + |
| 87 | +If [`bolt-tailwind`](https://github.com/boltpackages/bolt-tailwind) is installed, it will automatically run the Tailwind `compile --watch` process. |
| 88 | + |
| 89 | +## Debugging |
| 90 | + |
| 91 | +[View on YouTube →](https://www.youtube.com/watch?v=pG0KaJSVyBw) |
| 92 | + |
| 93 | +Since `bolt work` runs multiple processes at once, the regular [pdb](https://docs.python.org/3/library/pdb.html) debuggers can be hard to use. |
| 94 | +Instead, we include [microsoft/debugpy](https://github.com/microsoft/debugpy) and an `attach` function to make it even easier to use VS Code's debugger. |
| 95 | + |
| 96 | +First, import and run the `debug.attach()` function: |
| 97 | + |
| 98 | +```python |
| 99 | +class HomeView(TemplateView): |
| 100 | + template_name = "home.html" |
| 101 | + |
| 102 | + def get_context(self, **kwargs): |
| 103 | + context = super().get_context(**kwargs) |
| 104 | + |
| 105 | + # Make sure the debugger is attached (will need to be if runserver reloads) |
| 106 | + from bolt.work import debug; debug.attach() |
| 107 | + |
| 108 | + # Add a breakpoint (or use the gutter in VSCode to add one) |
| 109 | + breakpoint() |
| 110 | + |
| 111 | + return context |
| 112 | +``` |
| 113 | + |
| 114 | +When you load the page, you'll see "Waiting for debugger to attach...". |
| 115 | + |
| 116 | +Add a new VS Code debug configuration (using localhost and port 5768) by saving this to `.vscode/launch.json` or using the GUI: |
| 117 | + |
| 118 | +```json |
| 119 | +// .vscode/launch.json |
| 120 | +{ |
| 121 | + // Use IntelliSense to learn about possible attributes. |
| 122 | + // Hover to view descriptions of existing attributes. |
| 123 | + // For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387 |
| 124 | + "version": "0.2.0", |
| 125 | + "configurations": [ |
| 126 | + { |
| 127 | + "name": "Bolt: Attach to Django", |
| 128 | + "type": "python", |
| 129 | + "request": "attach", |
| 130 | + "connect": { |
| 131 | + "host": "localhost", |
| 132 | + "port": 5678 |
| 133 | + }, |
| 134 | + "pathMappings": [ |
| 135 | + { |
| 136 | + "localRoot": "${workspaceFolder}", |
| 137 | + "remoteRoot": "." |
| 138 | + } |
| 139 | + ], |
| 140 | + "justMyCode": true, |
| 141 | + "django": true |
| 142 | + } |
| 143 | + ] |
| 144 | +} |
| 145 | +``` |
| 146 | + |
| 147 | +Then in the "Run and Debug" tab, you can click the green arrow next to "Bolt: Attach to Django" to start the debugger. |
| 148 | + |
| 149 | +In your terminal is should tell you it was attached, and when you hit a breakpoint you'll see the debugger information in VS Code. |
| 150 | +If Django's runserver reloads, you'll be prompted to reattach by clicking the green arrow again. |
0 commit comments