We read every piece of feedback, and take your input very seriously.
To see all available qualifiers, see our documentation.
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
PHP version: 8.0 Package version: v1.0.10
How to reproduce:
<?php declare(strict_types=1); namespace App\Database; use App\Database\Typecast\Uuid; use App\Exception; use App\Repository; use App\Database\Column; use Cycle\Annotated\Annotation as Cycle; use Doctrine\Common\Collections\ArrayCollection; use Doctrine\Common\Collections\Collection; #[Cycle\Entity( repository: Repository\User::class )] class User { use Column\Id; #[Cycle\Column(type: 'string(64)')] public string $username; #[Cycle\Relation\HasMany(target: Comment::class)] private Collection $comments; public function __construct(Uuid $id, string $username, string $password) { $this->id = $id; $this->username = $username; $this->comments = new ArrayCollection(); } public function getComments(): Collection { return $this->comments; } }
<?php declare(strict_types=1); namespace App\Database; use App\Repository; use App\Database\Column; use Cycle\Annotated\Annotation as Cycle; #[Cycle\Entity( repository: Repository\Comment::class )] class AuthCode { use Column\Id; #[Cycle\Relation\BelongsTo(target: User::class, nullable: true)] private ?User $user = null; #[Cycle\Column(type: 'string')] private string $text; public function setUser(?User $user): void { $this->user = $user; } public function setText(string $value): void { $this->text = $value; } public function getText(): string { return $this->text; } public function getUser(): ?User { return $this->user; } }
php app.php cycle:migrate -r
Results:
P.S. Column Id Trait:
<?php declare(strict_types=1); namespace App\Database\Column; use Cycle\Annotated\Annotation as Cycle; use App\Database\Typecast\Uuid; trait Id { #[Cycle\Column(type: 'uuid', typecast: Uuid::class, primary: true)] private ?Uuid $id = null; public function getId(): ?Uuid { return $this->id; } }
Results expected as nullable: true.
nullable: true
The text was updated successfully, but these errors were encountered:
probably this is the same problem as I faced in this issue cycle/orm#120
Sorry, something went wrong.
Try to add nullable to comments relation
#[Cycle\Relation\HasMany(target: Comment::class, nullable: true)] private Collection $comments;
No branches or pull requests
PHP version: 8.0
Package version: v1.0.10
How to reproduce:
php app.php cycle:migrate -r
Results:
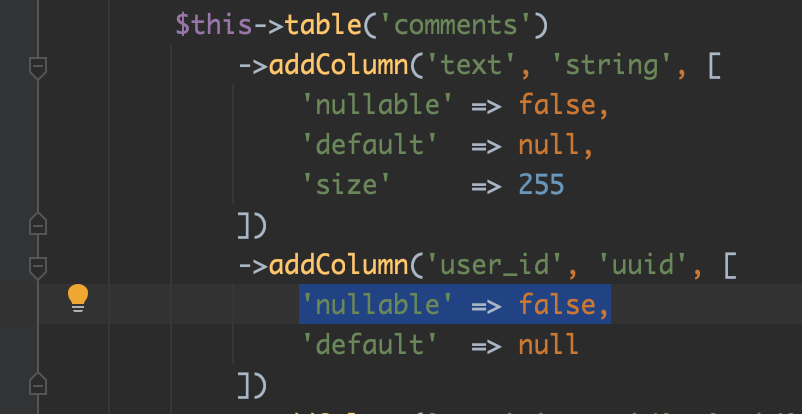
P.S. Column Id Trait:
Results expected as
nullable: true
.The text was updated successfully, but these errors were encountered: