|
1 |
| -**Springboot重要知识点&高频面试题**是我的[知识星球](https://topjavaer.cn/zsxq/introduce.html)**内部专属资料**,已经整理到Java面试手册**完整版**。 |
| 1 | +--- |
| 2 | +sidebar: heading |
| 3 | +title: Springboot常见面试题总结 |
| 4 | +category: 框架 |
| 5 | +tag: |
| 6 | + - SpringBoot |
| 7 | +head: |
| 8 | + - - meta |
| 9 | + - name: keywords |
| 10 | + content: Spring Boot面试题,Spring Boot,自动配置,Spring Boot注解,Spring Boot多数据源 |
| 11 | + - - meta |
| 12 | + - name: description |
| 13 | + content: 高质量的Springboot常见知识点和面试题总结,让天下没有难背的八股文! |
| 14 | +--- |
2 | 15 |
|
3 |
| -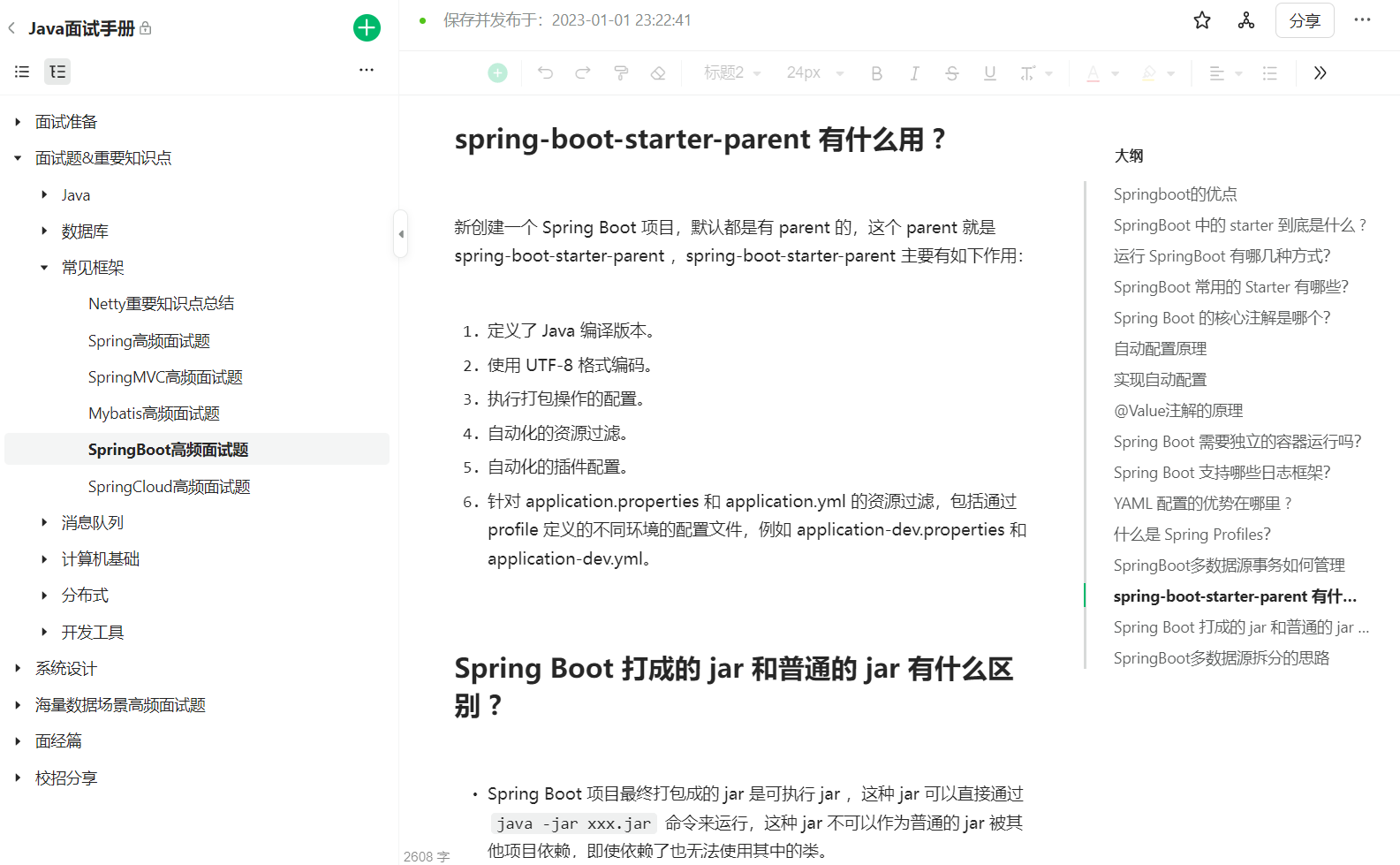 |
4 | 16 |
|
5 |
| -除了Java面试手册完整版之外,星球还有很多其他**优质资料**,比如包括Java项目、进阶知识、实战经验总结、优质书籍、笔试面试资源等等。 |
| 17 | +## Springboot的优点 |
6 | 18 |
|
7 |
| -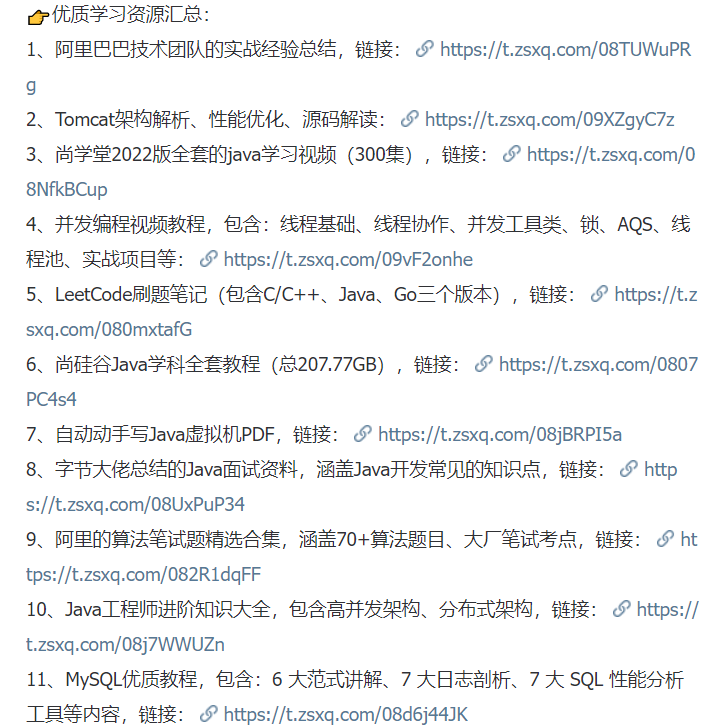 |
| 19 | +- 内置servlet容器,不需要在服务器部署 tomcat。只需要将项目打成 jar 包,使用 java -jar xxx.jar一键式启动项目 |
| 20 | +- SpringBoot提供了starter,把常用库聚合在一起,简化复杂的环境配置,快速搭建spring应用环境 |
| 21 | +- 可以快速创建独立运行的spring项目,集成主流框架 |
| 22 | +- 准生产环境的运行应用监控 |
8 | 23 |
|
9 |
| -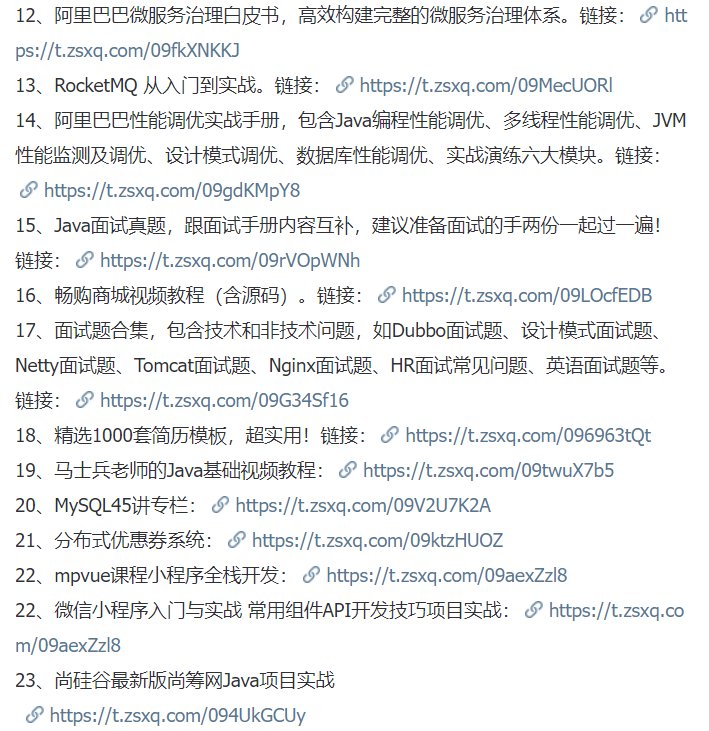 |
| 24 | +## SpringBoot 中的 starter 到底是什么 ? |
10 | 25 |
|
11 |
| -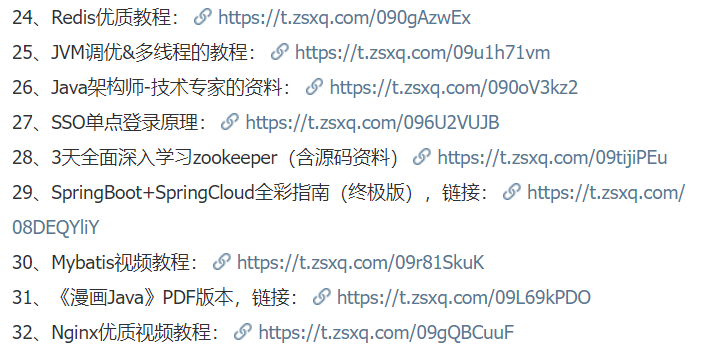 |
| 26 | +starter提供了一个自动化配置类,一般命名为 XXXAutoConfiguration ,在这个配置类中通过条件注解来决定一个配置是否生效(条件注解就是 Spring 中原本就有的),然后它还会提供一系列的默认配置,也允许开发者根据实际情况自定义相关配置,然后通过类型安全的属性注入将这些配置属性注入进来,新注入的属性会代替掉默认属性。正因为如此,很多第三方框架,我们只需要引入依赖就可以直接使用了。 |
12 | 27 |
|
13 |
| -**专属一对一的提问答疑**,帮你解答各种疑难问题,包括自学Java路线、职业规划、面试问题等等。大彬会**优先解答**球友的问题。 |
| 28 | +## 运行 SpringBoot 有哪几种方式? |
14 | 29 |
|
15 |
| -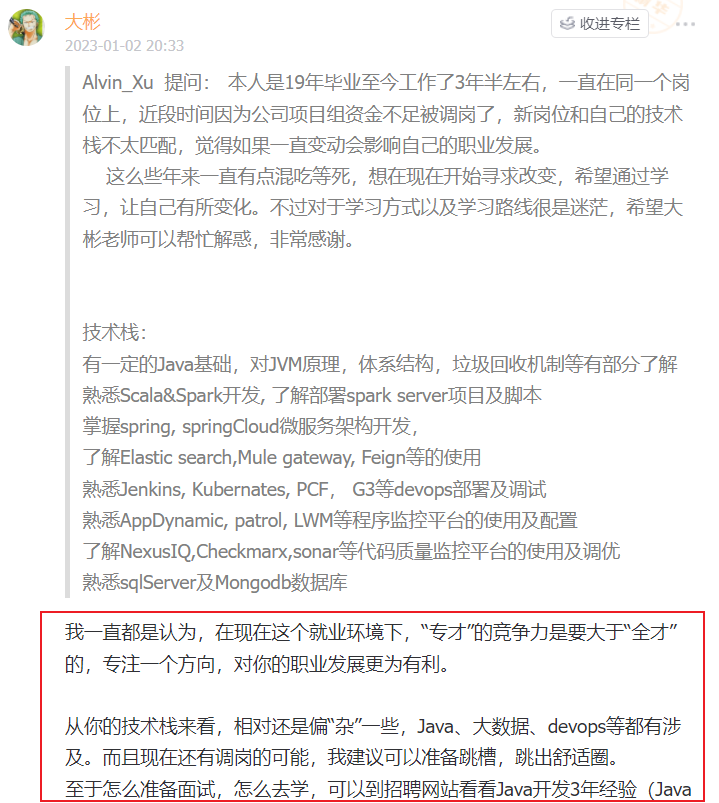 |
| 30 | +1. 打包用命令或者者放到容器中运行 |
| 31 | +2. 用 Maven/Gradle 插件运行 |
| 32 | +3. 直接执行 main 方法运行 |
16 | 33 |
|
17 |
| -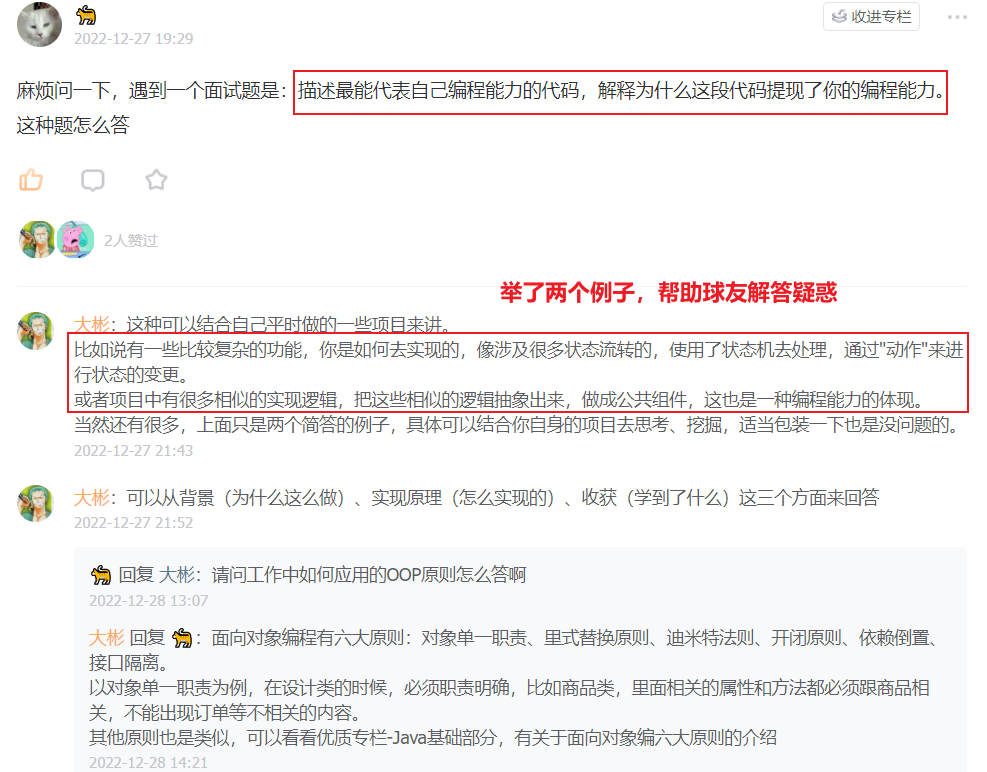 |
| 34 | +## SpringBoot 常用的 Starter 有哪些? |
18 | 35 |
|
19 |
| -另外星球还提供**简历指导、修改服务**,大彬已经帮**90**+个小伙伴修改了简历,相对还是比较有经验的。 |
| 36 | +1. spring-boot-starter-web :提供 Spring MVC + 内嵌的 Tomcat 。 |
| 37 | +2. spring-boot-starter-data-jpa :提供 Spring JPA + Hibernate 。 |
| 38 | +3. spring-boot-starter-data-Redis :提供 Redis 。 |
| 39 | +4. mybatis-spring-boot-starter :提供 MyBatis 。 |
20 | 40 |
|
21 |
| -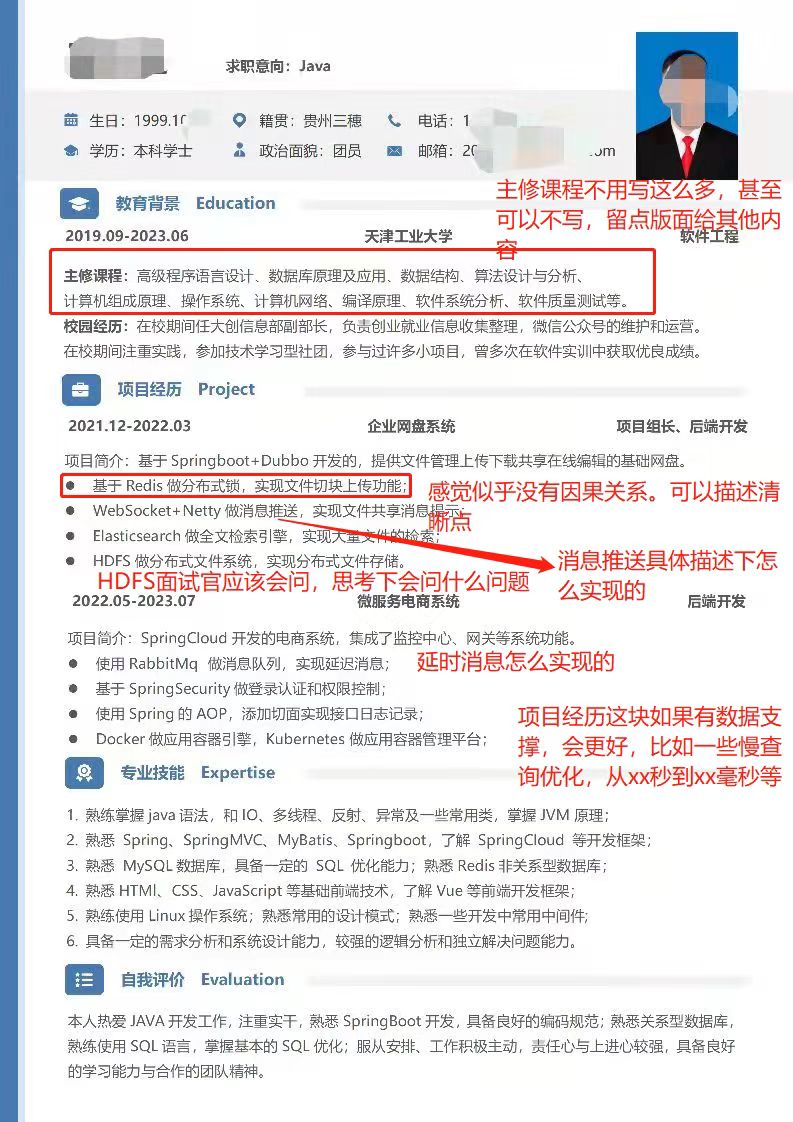 |
| 41 | +## Spring Boot 的核心注解是哪个? |
22 | 42 |
|
23 |
| -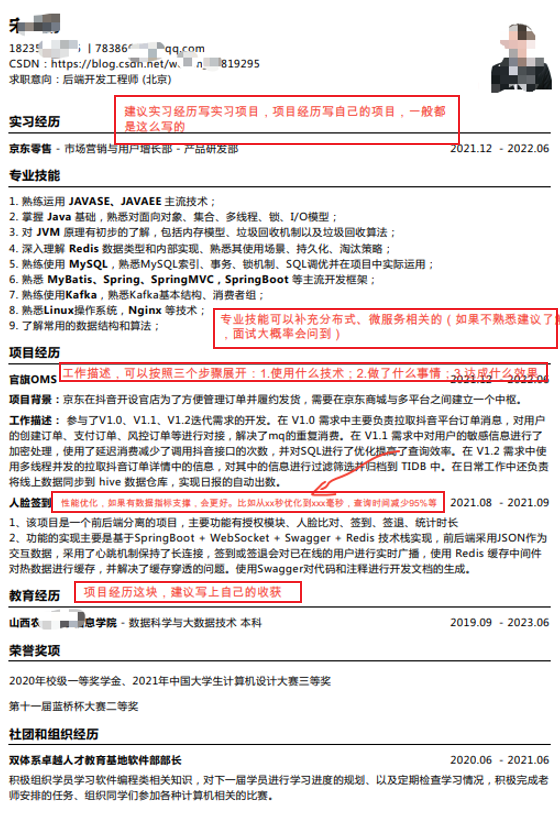 |
| 43 | +启动类上面的注解是@SpringBootApplication,它也是 Spring Boot 的核心注解,主要组合包含了以下 3 个注解: |
24 | 44 |
|
25 |
| -[知识星球](https://topjavaer.cn/zsxq/introduce.html)**加入方式**: |
| 45 | +- @SpringBootConfiguration:组合了 @Configuration 注解,实现配置文件的功能。 |
26 | 46 |
|
27 |
| -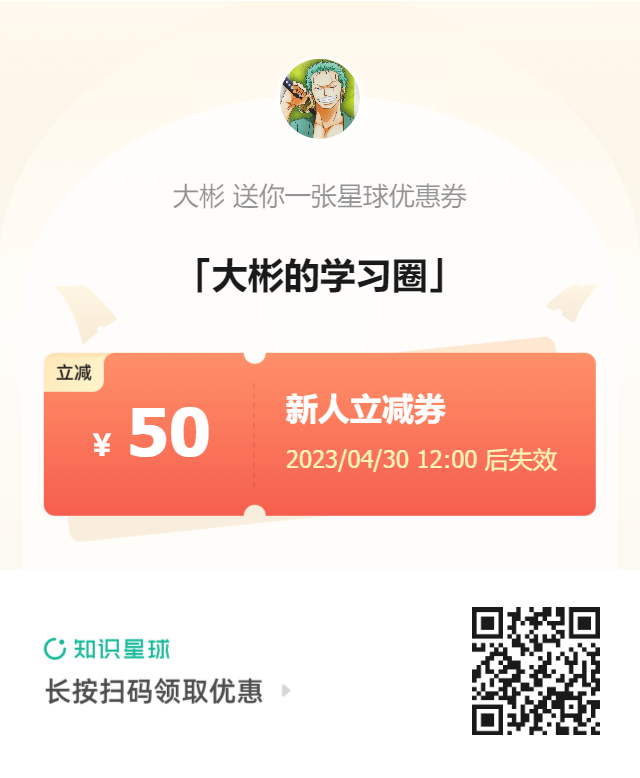 |
| 47 | +- @EnableAutoConfiguration:打开自动配置的功能,也可以关闭某个自动配置的选项,如关闭数据源自动配置功能: @SpringBootApplication(exclude = { DataSourceAutoConfiguration.class })。 |
| 48 | + |
| 49 | +- @ComponentScan:Spring组件扫描。 |
| 50 | + |
| 51 | +## 自动配置原理 |
| 52 | + |
| 53 | +SpringBoot实现自动配置原理图解: |
| 54 | + |
| 55 | +> 公众号【程序员大彬】,回复【自动配置】下载高清图片 |
| 56 | +
|
| 57 | +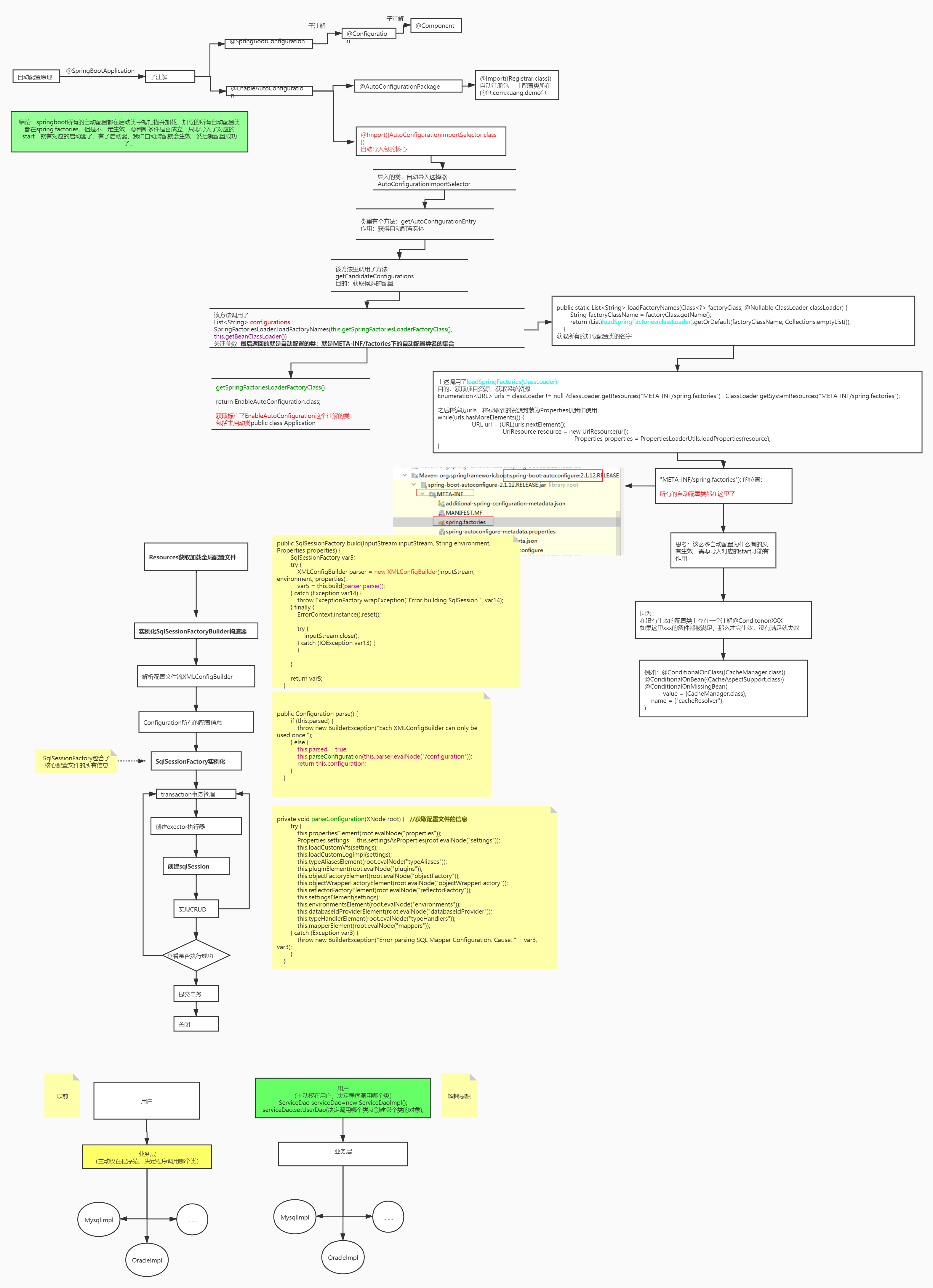 |
| 58 | + |
| 59 | +在 application.properties 中设置属性 debug=true,可以在控制台查看已启用和未启用的自动配置。 |
| 60 | + |
| 61 | +@SpringBootApplication是@Configuration、@EnableAutoConfiguration和@ComponentScan的组合。 |
| 62 | + |
| 63 | +@Configuration表示该类是Java配置类。 |
| 64 | + |
| 65 | +@ComponentScan开启自动扫描符合条件的bean(添加了@Controller、@Service等注解)。 |
| 66 | + |
| 67 | +@EnableAutoConfiguration会根据类路径中的jar依赖为项目进行自动配置,比如添加了`spring-boot-starter-web`依赖,会自动添加Tomcat和Spring MVC的依赖,然后Spring Boot会对Tomcat和Spring MVC进行自动配置(spring.factories EnableAutoConfiguration配置了`WebMvcAutoConfiguration`)。 |
| 68 | + |
| 69 | +```java |
| 70 | +@Target(ElementType.TYPE) |
| 71 | +@Retention(RetentionPolicy.RUNTIME) |
| 72 | +@Documented |
| 73 | +@Inherited |
| 74 | +@AutoConfigurationPackage |
| 75 | +@Import(EnableAutoConfigurationImportSelector.class) |
| 76 | +public @interface EnableAutoConfiguration { |
| 77 | +} |
| 78 | +``` |
| 79 | + |
| 80 | +EnableAutoConfiguration主要由 @AutoConfigurationPackage,@Import(EnableAutoConfigurationImportSelector.class)这两个注解组成的。 |
| 81 | + |
| 82 | +@AutoConfigurationPackage用于将启动类所在的包里面的所有组件注册到spring容器。 |
| 83 | + |
| 84 | +@Import 将EnableAutoConfigurationImportSelector注入到spring容器中,EnableAutoConfigurationImportSelector通过SpringFactoriesLoader从类路径下去读取META-INF/spring.factories文件信息,此文件中有一个key为org.springframework.boot.autoconfigure.EnableAutoConfiguration,定义了一组需要自动配置的bean。 |
| 85 | + |
| 86 | +```properties |
| 87 | +# Auto Configure |
| 88 | +org.springframework.boot.autoconfigure.EnableAutoConfiguration=\ |
| 89 | +org.springframework.boot.autoconfigure.admin.SpringApplicationAdminJmxAutoConfiguration,\ |
| 90 | +org.springframework.boot.autoconfigure.aop.AopAutoConfiguration,\ |
| 91 | +org.springframework.boot.autoconfigure.amqp.RabbitAutoConfiguration,\ |
| 92 | +org.springframework.boot.autoconfigure.batch.BatchAutoConfiguration,\ |
| 93 | +org.springframework.boot.autoconfigure.cache.CacheAutoConfiguration,\ |
| 94 | +``` |
| 95 | + |
| 96 | +这些配置类不是都会被加载,会根据xxxAutoConfiguration上的@ConditionalOnClass等条件判断是否加载,符合条件才会将相应的组件被加载到spring容器。(比如mybatis-spring-boot-starter,会自动配置sqlSessionFactory、sqlSessionTemplate、dataSource等mybatis所需的组件) |
| 97 | + |
| 98 | +```java |
| 99 | +@Configuration |
| 100 | +@ConditionalOnClass({ EnableAspectJAutoProxy.class, Aspect.class, Advice.class, |
| 101 | + AnnotatedElement.class }) //类路径存在EnableAspectJAutoProxy等类文件,才会加载此配置类 |
| 102 | +@ConditionalOnProperty(prefix = "spring.aop", name = "auto", havingValue = "true", matchIfMissing = true) |
| 103 | +public class AopAutoConfiguration { |
| 104 | + |
| 105 | + @Configuration |
| 106 | + @EnableAspectJAutoProxy(proxyTargetClass = false) |
| 107 | + @ConditionalOnProperty(prefix = "spring.aop", name = "proxy-target-class", havingValue = "false", matchIfMissing = false) |
| 108 | + public static class JdkDynamicAutoProxyConfiguration { |
| 109 | + |
| 110 | + } |
| 111 | + |
| 112 | + @Configuration |
| 113 | + @EnableAspectJAutoProxy(proxyTargetClass = true) |
| 114 | + @ConditionalOnProperty(prefix = "spring.aop", name = "proxy-target-class", havingValue = "true", matchIfMissing = true) |
| 115 | + public static class CglibAutoProxyConfiguration { |
| 116 | + |
| 117 | + } |
| 118 | + |
| 119 | +} |
| 120 | +``` |
| 121 | + |
| 122 | +全局配置文件中的属性如何生效,比如:server.port=8081,是如何生效的? |
| 123 | + |
| 124 | +@ConfigurationProperties的作用就是将配置文件的属性绑定到对应的bean上。全局配置的属性如:server.port等,通过@ConfigurationProperties注解,绑定到对应的XxxxProperties bean,通过这个 bean 获取相应的属性(serverProperties.getPort())。 |
| 125 | + |
| 126 | +```java |
| 127 | +//server.port = 8080 |
| 128 | +@ConfigurationProperties(prefix = "server", ignoreUnknownFields = true) |
| 129 | +public class ServerProperties { |
| 130 | + private Integer port; |
| 131 | + private InetAddress address; |
| 132 | + |
| 133 | + @NestedConfigurationProperty |
| 134 | + private final ErrorProperties error = new ErrorProperties(); |
| 135 | + private Boolean useForwardHeaders; |
| 136 | + private String serverHeader; |
| 137 | + //... |
| 138 | +} |
| 139 | +``` |
| 140 | + |
| 141 | +## 实现自动配置 |
| 142 | + |
| 143 | +实现当某个类存在时,自动配置这个类的bean,并且可以在application.properties中配置bean的属性。 |
| 144 | + |
| 145 | +(1)新建Maven项目spring-boot-starter-hello,修改pom.xml如下: |
| 146 | + |
| 147 | +```xml |
| 148 | +<?xml version="1.0" encoding="UTF-8"?> |
| 149 | +<project xmlns="http://maven.apache.org/POM/4.0.0" |
| 150 | + xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" |
| 151 | + xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> |
| 152 | + <modelVersion>4.0.0</modelVersion> |
| 153 | + |
| 154 | + <groupId>com.tyson</groupId> |
| 155 | + <artifactId>spring-boot-starter-hello</artifactId> |
| 156 | + <version>1.0-SNAPSHOT</version> |
| 157 | + |
| 158 | + <dependencies> |
| 159 | + <dependency> |
| 160 | + <groupId>org.springframework.boot</groupId> |
| 161 | + <artifactId>spring-boot-autoconfigure</artifactId> |
| 162 | + <version>1.3.0.M1</version> |
| 163 | + </dependency> |
| 164 | + <dependency> |
| 165 | + <groupId>junit</groupId> |
| 166 | + <artifactId>junit</artifactId> |
| 167 | + <version>3.8.1</version> |
| 168 | + </dependency> |
| 169 | + </dependencies> |
| 170 | + |
| 171 | +</project> |
| 172 | +``` |
| 173 | + |
| 174 | +(2)属性配置 |
| 175 | + |
| 176 | +```java |
| 177 | +public class HelloService { |
| 178 | + private String msg; |
| 179 | + |
| 180 | + public String getMsg() { |
| 181 | + return msg; |
| 182 | + } |
| 183 | + |
| 184 | + public void setMsg(String msg) { |
| 185 | + this.msg = msg; |
| 186 | + } |
| 187 | + |
| 188 | + public String sayHello() { |
| 189 | + return "hello" + msg; |
| 190 | + |
| 191 | + } |
| 192 | +} |
| 193 | + |
| 194 | + |
| 195 | +import org.springframework.boot.context.properties.ConfigurationProperties; |
| 196 | + |
| 197 | +@ConfigurationProperties(prefix="hello") |
| 198 | +public class HelloServiceProperties { |
| 199 | + private static final String MSG = "world"; |
| 200 | + private String msg = MSG; |
| 201 | + |
| 202 | + public String getMsg() { |
| 203 | + return msg; |
| 204 | + } |
| 205 | + |
| 206 | + public void setMsg(String msg) { |
| 207 | + this.msg = msg; |
| 208 | + } |
| 209 | +} |
| 210 | +``` |
| 211 | + |
| 212 | +(3)自动配置类 |
| 213 | + |
| 214 | +```java |
| 215 | +import com.tyson.service.HelloService; |
| 216 | +import org.springframework.beans.factory.annotation.Autowired; |
| 217 | +import org.springframework.boot.autoconfigure.condition.ConditionalOnClass; |
| 218 | +import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean; |
| 219 | +import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty; |
| 220 | +import org.springframework.boot.context.properties.EnableConfigurationProperties; |
| 221 | +import org.springframework.context.annotation.Bean; |
| 222 | +import org.springframework.context.annotation.Configuration; |
| 223 | + |
| 224 | +@Configuration |
| 225 | +@EnableConfigurationProperties(HelloServiceProperties.class) //1 |
| 226 | +@ConditionalOnClass(HelloService.class) //2 |
| 227 | +@ConditionalOnProperty(prefix="hello", value = "enabled", matchIfMissing = true) //3 |
| 228 | +public class HelloServiceAutoConfiguration { |
| 229 | + |
| 230 | + @Autowired |
| 231 | + private HelloServiceProperties helloServiceProperties; |
| 232 | + |
| 233 | + @Bean |
| 234 | + @ConditionalOnMissingBean(HelloService.class) //4 |
| 235 | + public HelloService helloService() { |
| 236 | + HelloService helloService = new HelloService(); |
| 237 | + helloService.setMsg(helloServiceProperties.getMsg()); |
| 238 | + return helloService; |
| 239 | + } |
| 240 | +} |
| 241 | +``` |
| 242 | + |
| 243 | +1. @EnableConfigurationProperties 注解开启属性注入,将带有@ConfigurationProperties 注解的类注入为Spring 容器的 Bean。 |
| 244 | + |
| 245 | +2. 当 HelloService 在类路径的条件下。 |
| 246 | +3. 当设置 hello=enabled 的情况下,如果没有设置则默认为 true,即条件符合。 |
| 247 | +4. 当容器没有这个 Bean 的时候。 |
| 248 | + |
| 249 | +(4)注册配置 |
| 250 | + |
| 251 | +想要自动配置生效,需要注册自动配置类。在 src/main/resources 下新建 META-INF/spring.factories。添加以下内容: |
| 252 | + |
| 253 | +```factories |
| 254 | +org.springframework.boot.autoconfigure.EnableAutoConfiguration=\ |
| 255 | +com.tyson.config.HelloServiceAutoConfiguration |
| 256 | +``` |
| 257 | + |
| 258 | +"\\"是为了换行后仍然能读到属性。若有多个自动配置,则用逗号隔开。 |
| 259 | + |
| 260 | +(5)使用starter |
| 261 | + |
| 262 | +在 Spring Boot 项目的 pom.xml 中添加: |
| 263 | + |
| 264 | +```xml |
| 265 | +<dependency> |
| 266 | + <groupId>com.tyson</groupId> |
| 267 | + <artifactId>spring-boot-starter-hello</artifactId> |
| 268 | + <version>1.0-SNAPSHOT</version> |
| 269 | +</dependency> |
| 270 | +``` |
| 271 | + |
| 272 | +运行类如下: |
| 273 | + |
| 274 | +```java |
| 275 | +import com.tyson.service.HelloService; |
| 276 | +import org.springframework.beans.factory.annotation.Autowired; |
| 277 | +import org.springframework.boot.SpringApplication; |
| 278 | +import org.springframework.boot.autoconfigure.SpringBootApplication; |
| 279 | +import org.springframework.web.bind.annotation.RequestMapping; |
| 280 | +import org.springframework.web.bind.annotation.RestController; |
| 281 | + |
| 282 | +@RestController |
| 283 | +@SpringBootApplication |
| 284 | +public class SpringbootDemoApplication { |
| 285 | + |
| 286 | + @Autowired |
| 287 | + public HelloService helloService; |
| 288 | + |
| 289 | + @RequestMapping("/") |
| 290 | + public String index() { |
| 291 | + return helloService.getMsg(); |
| 292 | + } |
| 293 | + |
| 294 | + public static void main(String[] args) { |
| 295 | + SpringApplication.run(SpringbootDemoApplication.class, args); |
| 296 | + } |
| 297 | + |
| 298 | +} |
| 299 | +``` |
| 300 | + |
| 301 | +在项目中没有配置 HelloService bean,但是我们可以注入这个bean,这是通过自动配置实现的。 |
| 302 | + |
| 303 | +在 application.properties 中添加 debug 属性,运行配置类,在控制台可以看到: |
| 304 | + |
| 305 | +```java |
| 306 | + HelloServiceAutoConfiguration matched: |
| 307 | + - @ConditionalOnClass found required class 'com.tyson.service.HelloService' (OnClassCondition) |
| 308 | + - @ConditionalOnProperty (hello.enabled) matched (OnPropertyCondition) |
| 309 | + |
| 310 | + HelloServiceAutoConfiguration#helloService matched: |
| 311 | + - @ConditionalOnMissingBean (types: com.tyson.service.HelloService; SearchStrategy: all) did not find any beans (OnBeanCondition) |
| 312 | +``` |
| 313 | + |
| 314 | +可以在 application.properties 中配置 msg 的内容: |
| 315 | + |
| 316 | +```properties |
| 317 | +hello.msg=大彬 |
| 318 | +``` |
| 319 | + |
| 320 | +## @Value注解的原理 |
| 321 | + |
| 322 | +@Value的解析就是在bean初始化阶段。BeanPostProcessor定义了bean初始化前后用户可以对bean进行操作的接口方法,它的一个重要实现类`AutowiredAnnotationBeanPostProcessor`为bean中的@Autowired和@Value注解的注入功能提供支持。 |
| 323 | + |
| 324 | +## Spring Boot 需要独立的容器运行吗? |
| 325 | + |
| 326 | +不需要,内置了 Tomcat/ Jetty 等容器。 |
| 327 | + |
| 328 | +## Spring Boot 支持哪些日志框架? |
| 329 | + |
| 330 | +Spring Boot 支持 Java Util Logging, Log4j2, Lockback 作为日志框架,如果你使用 Starters 启动器,Spring Boot 将使用 Logback 作为默认日志框架,但是不管是那种日志框架他都支持将配置文件输出到控制台或者文件中。 |
| 331 | + |
| 332 | +## YAML 配置的优势在哪里 ? |
| 333 | + |
| 334 | +YAML 配置和传统的 properties 配置相比之下,有这些优势: |
| 335 | + |
| 336 | +- 配置有序 |
| 337 | +- 简洁明了,支持数组,数组中的元素可以是基本数据类型也可以是对象 |
| 338 | + |
| 339 | +缺点就是不支持 @PropertySource 注解导入自定义的 YAML 配置。 |
| 340 | + |
| 341 | +## 什么是 Spring Profiles? |
| 342 | + |
| 343 | +在项目的开发中,有些配置文件在开发、测试或者生产等不同环境中可能是不同的,例如数据库连接、redis的配置等等。那我们如何在不同环境中自动实现配置的切换呢?Spring给我们提供了profiles机制给我们提供的就是来回切换配置文件的功能 |
| 344 | + |
| 345 | +Spring Profiles 允许用户根据配置文件(dev,test,prod 等)来注册 bean。因此,当应用程序在开发中运行时,只有某些 bean 可以加载,而在 PRODUCTION中,某些其他 bean 可以加载。假设我们的要求是 Swagger 文档仅适用于 QA 环境,并且禁用所有其他文档。这可以使用配置文件来完成。Spring Boot 使得使用配置文件非常简单。 |
| 346 | + |
| 347 | +## SpringBoot多数据源事务如何管理 |
| 348 | + |
| 349 | +第一种方式是在service层的@TransactionManager中使用transactionManager指定DataSourceConfig中配置的事务。 |
| 350 | + |
| 351 | +第二种是使用jta-atomikos实现分布式事务管理。 |
| 352 | + |
| 353 | +## spring-boot-starter-parent 有什么用 ? |
| 354 | + |
| 355 | +新创建一个 Spring Boot 项目,默认都是有 parent 的,这个 parent 就是 spring-boot-starter-parent ,spring-boot-starter-parent 主要有如下作用: |
| 356 | + |
| 357 | +1. 定义了 Java 编译版本。 |
| 358 | +2. 使用 UTF-8 格式编码。 |
| 359 | +3. 执行打包操作的配置。 |
| 360 | +4. 自动化的资源过滤。 |
| 361 | +5. 自动化的插件配置。 |
| 362 | +6. 针对 application.properties 和 application.yml 的资源过滤,包括通过 profile 定义的不同环境的配置文件,例如 application-dev.properties 和 application-dev.yml。 |
| 363 | + |
| 364 | +## Spring Boot 打成的 jar 和普通的 jar 有什么区别 ? |
| 365 | + |
| 366 | +- Spring Boot 项目最终打包成的 jar 是可执行 jar ,这种 jar 可以直接通过 `java -jar xxx.jar` 命令来运行,这种 jar 不可以作为普通的 jar 被其他项目依赖,即使依赖了也无法使用其中的类。 |
| 367 | +- Spring Boot 的 jar 无法被其他项目依赖,主要还是他和普通 jar 的结构不同。普通的 jar 包,解压后直接就是包名,包里就是我们的代码,而 Spring Boot 打包成的可执行 jar 解压后,在 `\BOOT-INF\classes` 目录下才是我们的代码,因此无法被直接引用。如果非要引用,可以在 pom.xml 文件中增加配置,将 Spring Boot 项目打包成两个 jar ,一个可执行,一个可引用。 |
| 368 | + |
| 369 | +## SpringBoot多数据源拆分的思路 |
| 370 | + |
| 371 | +先在properties配置文件中配置两个数据源,创建分包mapper,使用@ConfigurationProperties读取properties中的配置,使用@MapperScan注册到对应的mapper包中 。 |
| 372 | + |
| 373 | + |
| 374 | + |
| 375 | +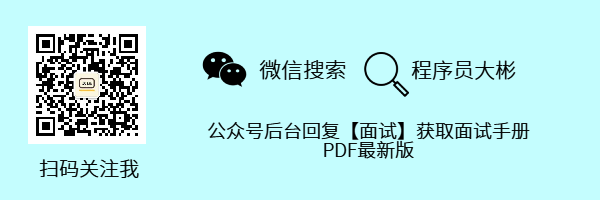 |
0 commit comments