This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Commit 56903ba
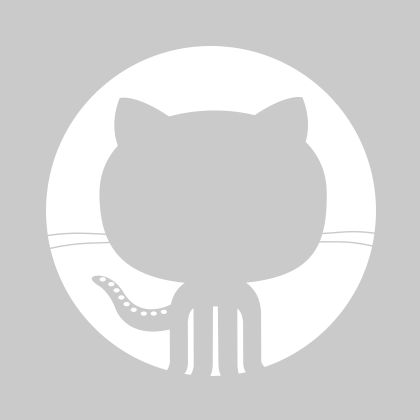
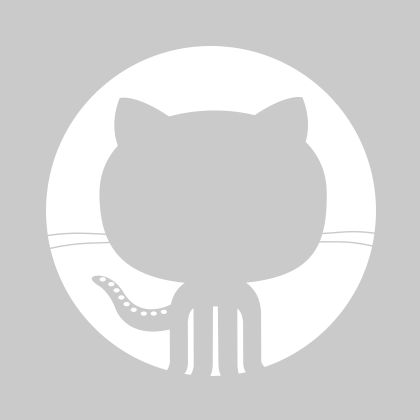
Rachit Tiwari
Rachit Tiwari
1 parent a8e61ff commit 56903ba
File tree
7 files changed
+64
-17
lines changed- src/main/java/io/github/lambdatest/gradle
7 files changed
+64
-17
lines changed+1-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
4 | 4 |
| |
5 | 5 |
| |
6 | 6 |
| |
7 |
| - | |
| 7 | + | |
8 | 8 |
| |
9 | 9 |
| |
10 | 10 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
9 | 9 |
| |
10 | 10 |
| |
11 | 11 |
| |
12 |
| - | |
13 |
| - | |
| 12 | + | |
| 13 | + | |
14 | 14 |
| |
15 | 15 |
| |
16 | 16 |
| |
| |||
19 | 19 |
| |
20 | 20 |
| |
21 | 21 |
| |
| 22 | + | |
22 | 23 |
| |
23 | 24 |
| |
24 | 25 |
| |
25 | 26 |
| |
26 | 27 |
| |
27 | 28 |
| |
28 | 29 |
| |
| 30 | + | |
29 | 31 |
| |
30 |
| - | |
| 32 | + | |
| 33 | + | |
31 | 34 |
| |
32 | 35 |
| |
33 | 36 |
| |
34 | 37 |
| |
35 | 38 |
| |
36 | 39 |
| |
37 | 40 |
| |
| 41 | + | |
38 | 42 |
| |
39 | 43 |
| |
40 | 44 |
| |
| |||
49 | 53 |
| |
50 | 54 |
| |
51 | 55 |
| |
52 |
| - | |
| 56 | + | |
| 57 | + | |
53 | 58 |
| |
54 | 59 |
| |
55 | 60 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
45 | 45 |
| |
46 | 46 |
| |
47 | 47 |
| |
| 48 | + | |
48 | 49 |
| |
49 | 50 |
| |
50 | 51 |
| |
| |||
66 | 67 |
| |
67 | 68 |
| |
68 | 69 |
| |
69 |
| - | |
| 70 | + | |
| 71 | + | |
70 | 72 |
| |
71 | 73 |
| |
72 | 74 |
| |
73 | 75 |
| |
74 | 76 |
| |
75 | 77 |
| |
76 |
| - | |
| 78 | + | |
77 | 79 |
| |
78 | 80 |
| |
79 | 81 |
| |
| |||
96 | 98 |
| |
97 | 99 |
| |
98 | 100 |
| |
99 |
| - | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
100 | 109 |
| |
101 | 110 |
| |
102 | 111 |
| |
| |||
217 | 226 |
| |
218 | 227 |
| |
219 | 228 |
| |
| 229 | + | |
| 230 | + | |
| 231 | + | |
| 232 | + | |
| 233 | + | |
| 234 | + | |
| 235 | + | |
| 236 | + | |
| 237 | + | |
220 | 238 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
21 | 21 |
| |
22 | 22 |
| |
23 | 23 |
| |
| 24 | + | |
24 | 25 |
| |
25 | 26 |
| |
26 | 27 |
| |
| |||
33 | 34 |
| |
34 | 35 |
| |
35 | 36 |
| |
36 |
| - | |
| 37 | + | |
| 38 | + | |
37 | 39 |
| |
38 | 40 |
| |
39 | 41 |
| |
40 | 42 |
| |
41 | 43 |
| |
42 | 44 |
| |
43 |
| - | |
| 45 | + | |
44 | 46 |
| |
45 | 47 |
| |
46 | 48 |
| |
| |||
80 | 82 |
| |
81 | 83 |
| |
82 | 84 |
| |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
83 | 89 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
24 | 24 |
| |
25 | 25 |
| |
26 | 26 |
| |
| 27 | + | |
27 | 28 |
| |
28 | 29 |
| |
29 | 30 |
| |
| |||
41 | 42 |
| |
42 | 43 |
| |
43 | 44 |
| |
44 |
| - | |
| 45 | + | |
| 46 | + | |
45 | 47 |
| |
46 | 48 |
| |
47 | 49 |
| |
48 | 50 |
| |
49 | 51 |
| |
50 | 52 |
| |
| 53 | + | |
51 | 54 |
| |
52 | 55 |
| |
53 | 56 |
| |
| |||
70 | 73 |
| |
71 | 74 |
| |
72 | 75 |
| |
| 76 | + | |
| 77 | + | |
| 78 | + | |
73 | 79 |
| |
74 | 80 |
| |
75 | 81 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
16 | 16 |
| |
17 | 17 |
| |
18 | 18 |
| |
| 19 | + | |
19 | 20 |
| |
20 | 21 |
| |
21 | 22 |
| |
22 | 23 |
| |
23 | 24 |
| |
24 | 25 |
| |
25 | 26 |
| |
| 27 | + | |
26 | 28 |
| |
27 |
| - | |
| 29 | + | |
| 30 | + | |
28 | 31 |
| |
29 | 32 |
| |
30 | 33 |
| |
| 34 | + | |
31 | 35 |
| |
32 | 36 |
| |
33 | 37 |
| |
| |||
42 | 46 |
| |
43 | 47 |
| |
44 | 48 |
| |
45 |
| - | |
| 49 | + | |
| 50 | + | |
46 | 51 |
| |
47 | 52 |
| |
48 | 53 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
35 | 35 |
| |
36 | 36 |
| |
37 | 37 |
| |
| 38 | + | |
38 | 39 |
| |
39 | 40 |
| |
40 | 41 |
| |
41 |
| - | |
| 42 | + | |
| 43 | + | |
42 | 44 |
| |
43 | 45 |
| |
44 | 46 |
| |
| |||
48 | 50 |
| |
49 | 51 |
| |
50 | 52 |
| |
51 |
| - | |
| 53 | + | |
52 | 54 |
| |
53 | 55 |
| |
54 | 56 |
| |
55 | 57 |
| |
56 | 58 |
| |
57 | 59 |
| |
58 |
| - | |
59 |
| - | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
60 | 67 |
| |
61 | 68 |
| |
62 | 69 |
| |
|
0 commit comments