|
| 1 | +--- |
| 2 | +Title: '.Contour()' |
| 3 | +Description: 'Creates a contour plot, which represents 3D surface data in 2D using contour lines or filled color regions to show variations in value.' |
| 4 | +Subjects: |
| 5 | + - 'Data Science' |
| 6 | + - 'Data Visualization' |
| 7 | +Tags: |
| 8 | + - 'Data' |
| 9 | + - 'Data Structures' |
| 10 | + - 'Functions' |
| 11 | + - 'Plotly' |
| 12 | +CatalogContent: |
| 13 | + - 'learn-python-3' |
| 14 | + - 'paths/data-science' |
| 15 | +--- |
| 16 | + |
| 17 | +In Plotly, the **`.Contour()`** function creates a contour plot, which represents 3D surface data in a 2D projection using contour lines or filled color regions. This function is useful for visualizing gradual variations in a dataset over a 2D plane, such as temperature distributions, elevation maps, and probability density functions. |
| 18 | + |
| 19 | +## Syntax |
| 20 | + |
| 21 | +```pseudo |
| 22 | +plotly.graph_objects.Contour(z=None, x=None, y=None, colorscale=None, contours=None, ...) |
| 23 | +``` |
| 24 | + |
| 25 | +- `z`: A 2D array (list or [NumPy array](https://www.codecademy.com/resources/docs/numpy/ndarray)) representing the values to be contoured. |
| 26 | +- `x`: A 1D or 2D array defining the x-coordinates corresponding to `z` values. |
| 27 | +- `y`: A 1D or 2D array defining the y-coordinates corresponding to `z` values. |
| 28 | +- `colorscale`: Defines the color scheme of the contour plot (e.g., `"Viridis"`, `"Jet"`, etc.). |
| 29 | +- `contours`: Controls the contour levels with a dictionary containing: |
| 30 | + - `start`: The starting value of the contours. |
| 31 | + - `end`: The ending value of the contours. |
| 32 | + - `size`: The step size between contour levels. |
| 33 | + |
| 34 | +> **Note:** The ellipsis (...) in the syntax indicates that there can be additional optional parameters beyond those listed here. |
| 35 | +
|
| 36 | +## Example |
| 37 | + |
| 38 | +The following example demonstrates the usage of the `.Contour()` function: |
| 39 | + |
| 40 | +```py |
| 41 | +import plotly.graph_objects as go |
| 42 | + |
| 43 | +# Define x and y coordinates |
| 44 | +x = [-2, -1, 0, 1, 2] |
| 45 | +y = [-2, -1, 0, 1, 2] |
| 46 | + |
| 47 | +# Define a 2D list for z values |
| 48 | +z = [[0.1, 0.2, 0.3, 0.2, 0.1], |
| 49 | + [0.2, 0.4, 0.6, 0.4, 0.2], |
| 50 | + [0.3, 0.6, 1.0, 0.6, 0.3], |
| 51 | + [0.2, 0.4, 0.6, 0.4, 0.2], |
| 52 | + [0.1, 0.2, 0.3, 0.2, 0.1]] |
| 53 | + |
| 54 | +# Create a contour plot |
| 55 | +fig = go.Figure(data=[go.Contour(z=z, # Data values for contour levels |
| 56 | + x=x, # X-coordinates |
| 57 | + y=y, # Y-coordinates |
| 58 | + colorscale="Viridis", # Color scheme |
| 59 | + contours=dict(start=0, end=1, size=0.1))]) # Define contour levels |
| 60 | + |
| 61 | +# Display the plot |
| 62 | +fig.show() |
| 63 | +``` |
| 64 | + |
| 65 | +The above code generates the following output: |
| 66 | + |
| 67 | +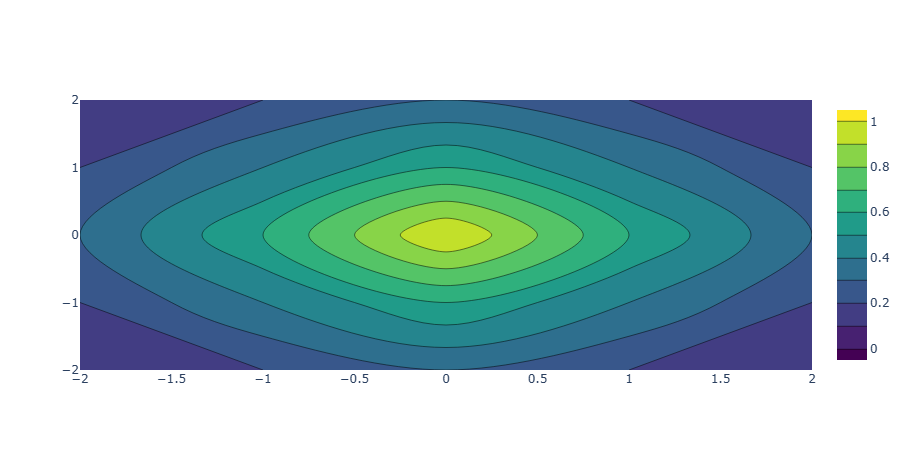 |
0 commit comments