|
1 | 1 | ---
|
2 | 2 | Title: 'Loops'
|
3 |
| -Description: 'C++ has two types of loops: For and While. Loops execute the same group of statements multiple times until the condition is no longer true.' |
| 3 | +Description: 'Loops provide a way to execute a block of code multiple times, making programs more efficient and concise.' |
4 | 4 | Subjects:
|
| 5 | + - 'Code Foundations' |
5 | 6 | - 'Computer Science'
|
6 |
| - - 'Game Development' |
7 | 7 | Tags:
|
| 8 | + - 'Algorithms' |
| 9 | + - 'Control Flow' |
| 10 | + - 'Iterators' |
8 | 11 | - 'Loops'
|
9 |
| - - 'While' |
10 |
| - - 'For' |
11 | 12 | CatalogContent:
|
12 | 13 | - 'learn-c-plus-plus'
|
13 | 14 | - 'paths/computer-science'
|
14 | 15 | ---
|
15 | 16 |
|
16 |
| -A **loop** can execute a statement or group of statements multiple times and the following is the general form of a loop statement in most programming languages. |
| 17 | +In C++ programming, executing a task multiple times without **loops** requires duplicating code, which is inefficient and hard to maintain. For example, displaying a message 10 times would involve writing the same `cout` statement repeatedly: |
17 | 18 |
|
18 |
| -## While Loop |
19 |
| - |
20 |
| -A `while` loop statement repeatedly executes the code block within as long as the condition is true. The moment the condition becomes false, the program will exit the loop. |
| 19 | +```cpp |
| 20 | +#include <iostream> |
| 21 | +using namespace std; |
21 | 22 |
|
22 |
| -> **Note:** The `while` loop might not ever run. If the condition is initially `false`, the code block will be skipped. |
| 23 | +int main() { |
| 24 | + cout << "This is a text" << endl; |
| 25 | + cout << "This is a text" << endl; |
| 26 | + cout << "This is a text" << endl; |
| 27 | + cout << "This is a text" << endl; |
| 28 | + cout << "This is a text" << endl; |
| 29 | + cout << "This is a text" << endl; |
| 30 | + cout << "This is a text" << endl; |
| 31 | + cout << "This is a text" << endl; |
| 32 | + cout << "This is a text" << endl; |
| 33 | + cout << "This is a text" << endl; |
23 | 34 |
|
24 |
| -```cpp |
25 |
| -while (password != 1234) { |
26 |
| - std::cout << "Try again: "; |
27 |
| - std::cin >> password; |
| 35 | + return 0; |
28 | 36 | }
|
29 | 37 | ```
|
30 | 38 |
|
31 |
| -## For Loop |
| 39 | +This method is time-consuming, error-prone, and difficult to maintain. To address this, C++ provides **loops**, control structures that execute a block of code repeatedly based on a condition. By using loops, code becomes more concise, efficient, and easier to manage. They are one of the fundamental building blocks in programming that help solve problems involving repetition. |
| 40 | + |
| 41 | +## How Loops Work |
| 42 | + |
| 43 | +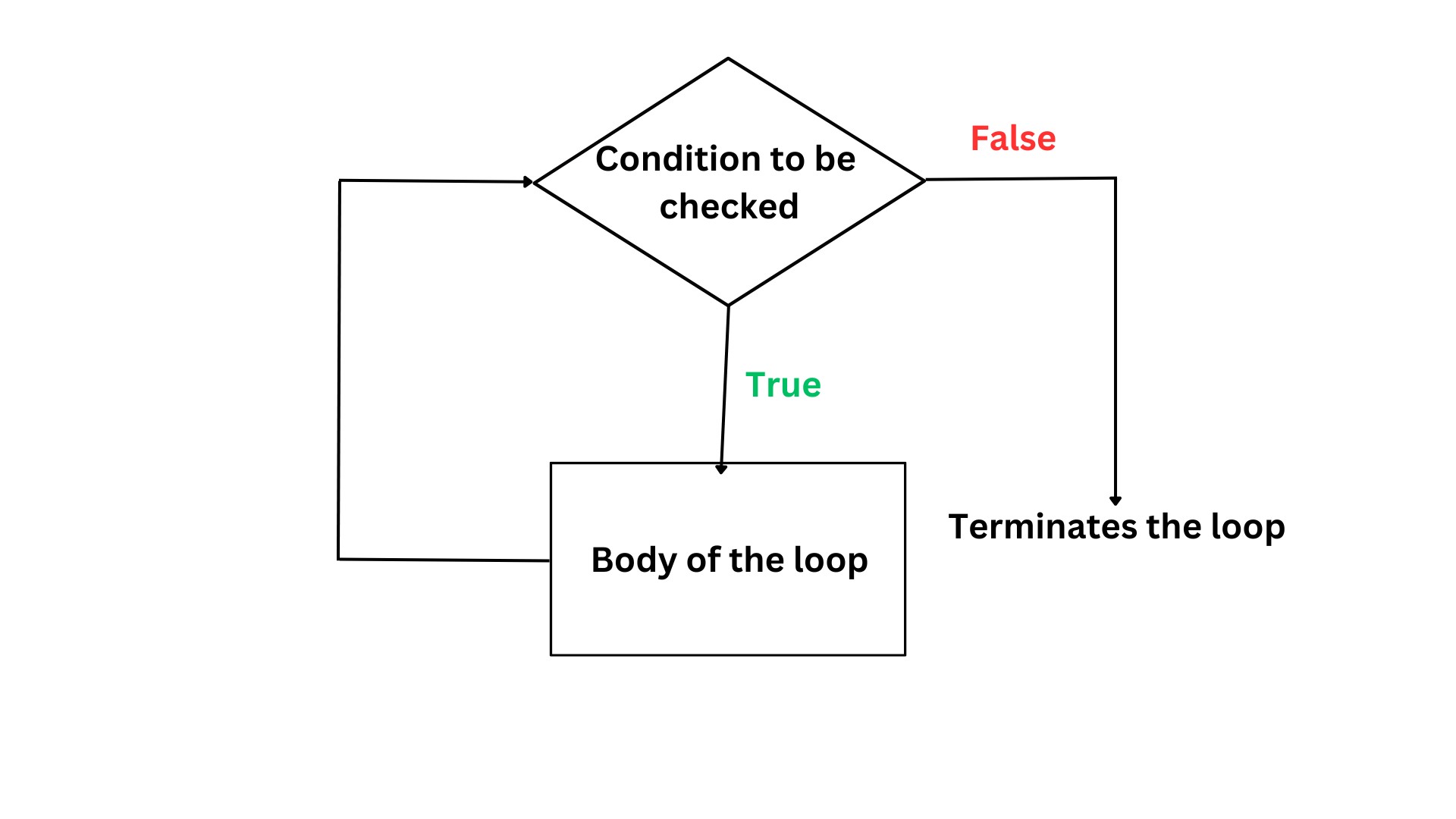 |
32 | 44 |
|
33 |
| -A `for` loop executes a code block a specific number of times. It has three parts: |
| 45 | +The general flow of a loop involves: |
34 | 46 |
|
35 |
| -- The initialization of a counter |
36 |
| -- The continue condition |
37 |
| -- The increment/decrement of the counter |
| 47 | +1. An initialization (setting up a starting point) |
| 48 | +2. A condition check (determining whether to run the loop) |
| 49 | +3. Code execution (running the statements inside the loop) |
| 50 | +4. An update (modifying values before checking the condition again) |
38 | 51 |
|
39 |
| -This example prints 0 to 9 on the screen: |
| 52 | +Using the earlier example, we can use a loop to display "This is a text" 10 times with just a few lines of code: |
40 | 53 |
|
41 | 54 | ```cpp
|
42 |
| -for (int i = 0; i < 10; i++) { |
43 |
| - std::cout << i << "\n"; |
| 55 | +#include <iostream> |
| 56 | +using namespace std; |
| 57 | + |
| 58 | +int main() { |
| 59 | + for (int i = 0; i < 10; i++) { |
| 60 | + cout << "This is a text" << endl; |
| 61 | + } |
| 62 | + |
| 63 | + return 0; |
44 | 64 | }
|
45 | 65 | ```
|
| 66 | + |
| 67 | +## Types of Loops in C++ |
| 68 | + |
| 69 | +C++ provides several types of loops, each with its own use cases: |
| 70 | + |
| 71 | +- **for loop**: Used when the number of iterations is known beforehand. |
| 72 | +- **while loop**: Executes as long as the specified condition remains true. |
| 73 | +- **do-while loop**: Similar to while loop, but guarantees at least one execution of the code block. |
| 74 | +- **range-based for loop**: Introduced in C++11, it simplifies iteration over elements in arrays, vectors, and other containers. |
0 commit comments