|
| 1 | +--- |
| 2 | +Title: '.odeint()' |
| 3 | +Description: 'Solves ordinary differential equations in SciPy using the LSODA method, automatically handling stiff and non-stiff problems.' |
| 4 | +Subjects: |
| 5 | + - 'Computer Science' |
| 6 | + - 'Data Science' |
| 7 | +Tags: |
| 8 | + - 'Data' |
| 9 | + - 'Math' |
| 10 | + - 'Python' |
| 11 | +CatalogContent: |
| 12 | + - 'learn-python-3' |
| 13 | + - 'paths/computer-science' |
| 14 | +--- |
| 15 | + |
| 16 | +The **`odeint()`** function from SciPy's [`integrate`](https://www.codecademy.com/resources/docs/scipy/scipy-integrate) module is a powerful tool for solving initial value problems for _Ordinary Differential Equations (ODEs)_. |
| 17 | + |
| 18 | +It integrates a system of ordinary differential equations using the LSODA method from the FORTRAN library `odepack`, which automatically switches between stiff and non-stiff methods based on the problem's characteristics. |
| 19 | + |
| 20 | +## Syntax |
| 21 | + |
| 22 | +The general syntax for using `odeint()` is as follows: |
| 23 | + |
| 24 | +```pseudo |
| 25 | +from scipy.integrate import odeint |
| 26 | +
|
| 27 | +solution = odeint(func, y0, t, args=(), Dfun=None, col_deriv=0, full_output=0, ml=None, mu=None, rtol=None, atol=None, tcrit=None, h0=0.0, hmax=0.0, hmin=0.0, ixpr=0, mxstep=500, mxhnil=10, mxordn=12, mxords=5) |
| 28 | +``` |
| 29 | + |
| 30 | +- `func`: A callable that defines the derivative of the system, ({dy}/{dt} = f(y, t, ...)). |
| 31 | +- `y0`: Initial condition(s) (array-like). Represents the initial state of the system. |
| 32 | +- `t`: Array of time points at which the solution is to be computed (1D array-like). |
| 33 | +- `args` (Optional): Tuple of extra arguments to pass to `func`. |
| 34 | + |
| 35 | +Other parameters are optional and control solver behavior, precision, and output verbosity. |
| 36 | + |
| 37 | +It returns a 2D [NumPy](https://www.codecademy.com/resources/docs/numpy) array, where each row corresponds to the solution at a specific time point in `t`. |
| 38 | + |
| 39 | +## Example |
| 40 | + |
| 41 | +The code below numerically solves a first-order ordinary differential equation using `odeint`. The model function defines the ODE, and `odeint` integrates it over specified time points starting from the initial condition, and the results are plotted to visualize the solution: |
| 42 | + |
| 43 | +```py |
| 44 | +import numpy as np |
| 45 | +from scipy.integrate import odeint |
| 46 | +import matplotlib.pyplot as plt |
| 47 | + |
| 48 | +def model(y, t): |
| 49 | + dydt = -2 * y + 3 |
| 50 | + return dydt |
| 51 | + |
| 52 | +# Initial condition |
| 53 | +y0 = 5 |
| 54 | + |
| 55 | +# Time points |
| 56 | +t = np.linspace(0, 5, 100) |
| 57 | + |
| 58 | +# Solve ODE |
| 59 | +solution = odeint(model, y0, t) |
| 60 | + |
| 61 | +# Plot results (indexing the solution to get the actual y values) |
| 62 | +plt.plot(t, solution[:, 0]) Since solution is a 2D array, we index its first column to extract the solution values. |
| 63 | +plt.title('Solution of dy/dt = -2y + 3') |
| 64 | +plt.xlabel('Time (t)') |
| 65 | +plt.ylabel('y(t)') |
| 66 | +plt.grid() |
| 67 | +plt.show() |
| 68 | +``` |
| 69 | + |
| 70 | +The output plot shows the numerical solution of the ODE, illustrating how `y(t)` evolves over time: |
| 71 | + |
| 72 | +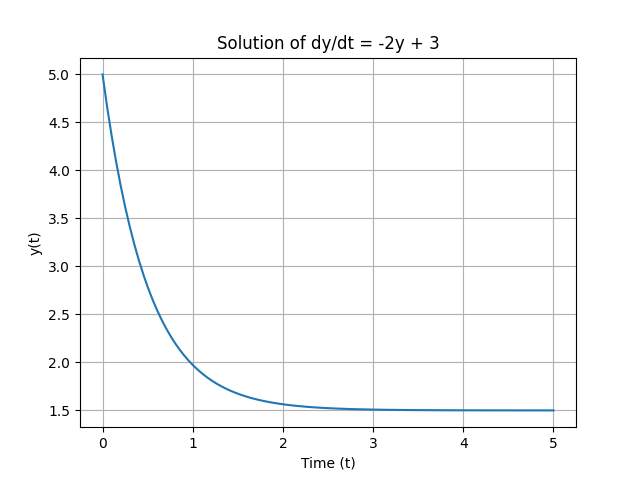 |
| 73 | + |
| 74 | +`odeint()` is ideal for many scientific and engineering applications due to its robustness and flexibility. |
| 75 | + |
| 76 | +> For more advanced control or alternative solvers, consider using `scipy.integrate.solve_ivp()`, which offers a more modern API. |
0 commit comments