|
| 1 | +# 2058. Find the Minimum and Maximum Number of Nodes Between Critical Points |
| 2 | + |
| 3 | +- Difficulty: Medium. |
| 4 | +- Related Topics: Linked List. |
| 5 | +- Similar Questions: . |
| 6 | + |
| 7 | +## Problem |
| 8 | + |
| 9 | +A **critical point** in a linked list is defined as **either** a **local maxima** or a **local minima**. |
| 10 | + |
| 11 | +A node is a **local maxima** if the current node has a value **strictly greater** than the previous node and the next node. |
| 12 | + |
| 13 | +A node is a **local minima** if the current node has a value **strictly smaller** than the previous node and the next node. |
| 14 | + |
| 15 | +Note that a node can only be a local maxima/minima if there exists **both** a previous node and a next node. |
| 16 | + |
| 17 | +Given a linked list `head`, return **an array of length 2 containing **`[minDistance, maxDistance]`** where **`minDistance`** is the **minimum distance** between **any two distinct** critical points and **`maxDistance`** is the **maximum distance** between **any two distinct** critical points. If there are **fewer** than two critical points, return **`[-1, -1]`. |
| 18 | + |
| 19 | + |
| 20 | +Example 1: |
| 21 | + |
| 22 | +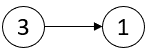 |
| 23 | + |
| 24 | +``` |
| 25 | +Input: head = [3,1] |
| 26 | +Output: [-1,-1] |
| 27 | +Explanation: There are no critical points in [3,1]. |
| 28 | +``` |
| 29 | + |
| 30 | +Example 2: |
| 31 | + |
| 32 | + |
| 33 | + |
| 34 | +``` |
| 35 | +Input: head = [5,3,1,2,5,1,2] |
| 36 | +Output: [1,3] |
| 37 | +Explanation: There are three critical points: |
| 38 | +- [5,3,1,2,5,1,2]: The third node is a local minima because 1 is less than 3 and 2. |
| 39 | +- [5,3,1,2,5,1,2]: The fifth node is a local maxima because 5 is greater than 2 and 1. |
| 40 | +- [5,3,1,2,5,1,2]: The sixth node is a local minima because 1 is less than 5 and 2. |
| 41 | +The minimum distance is between the fifth and the sixth node. minDistance = 6 - 5 = 1. |
| 42 | +The maximum distance is between the third and the sixth node. maxDistance = 6 - 3 = 3. |
| 43 | +``` |
| 44 | + |
| 45 | +Example 3: |
| 46 | + |
| 47 | + |
| 48 | + |
| 49 | +``` |
| 50 | +Input: head = [1,3,2,2,3,2,2,2,7] |
| 51 | +Output: [3,3] |
| 52 | +Explanation: There are two critical points: |
| 53 | +- [1,3,2,2,3,2,2,2,7]: The second node is a local maxima because 3 is greater than 1 and 2. |
| 54 | +- [1,3,2,2,3,2,2,2,7]: The fifth node is a local maxima because 3 is greater than 2 and 2. |
| 55 | +Both the minimum and maximum distances are between the second and the fifth node. |
| 56 | +Thus, minDistance and maxDistance is 5 - 2 = 3. |
| 57 | +Note that the last node is not considered a local maxima because it does not have a next node. |
| 58 | +``` |
| 59 | + |
| 60 | + |
| 61 | +**Constraints:** |
| 62 | + |
| 63 | + |
| 64 | + |
| 65 | +- The number of nodes in the list is in the range `[2, 105]`. |
| 66 | + |
| 67 | +- `1 <= Node.val <= 105` |
| 68 | + |
| 69 | + |
| 70 | + |
| 71 | +## Solution |
| 72 | + |
| 73 | +```javascript |
| 74 | +/** |
| 75 | + * Definition for singly-linked list. |
| 76 | + * function ListNode(val, next) { |
| 77 | + * this.val = (val===undefined ? 0 : val) |
| 78 | + * this.next = (next===undefined ? null : next) |
| 79 | + * } |
| 80 | + */ |
| 81 | +/** |
| 82 | + * @param {ListNode} head |
| 83 | + * @return {number[]} |
| 84 | + */ |
| 85 | +var nodesBetweenCriticalPoints = function(head) { |
| 86 | + var firstPoint = -1; |
| 87 | + var lastPoint = -1; |
| 88 | + var last = null; |
| 89 | + var now = head; |
| 90 | + var i = 0; |
| 91 | + var minDistance = Number.MAX_SAFE_INTEGER; |
| 92 | + while (now) { |
| 93 | + if (last && now.next && ((now.val > last.val && now.val > now.next.val) || (now.val < last.val && now.val < now.next.val))) { |
| 94 | + if (firstPoint === -1) firstPoint = i; |
| 95 | + if (lastPoint !== -1) minDistance = Math.min(minDistance, i - lastPoint); |
| 96 | + lastPoint = i; |
| 97 | + } |
| 98 | + last = now; |
| 99 | + now = now.next; |
| 100 | + i += 1; |
| 101 | + } |
| 102 | + if (firstPoint !== -1 && lastPoint !== -1 && lastPoint !== firstPoint) { |
| 103 | + return [minDistance, lastPoint - firstPoint]; |
| 104 | + } |
| 105 | + return [-1, -1]; |
| 106 | +}; |
| 107 | +``` |
| 108 | + |
| 109 | +**Explain:** |
| 110 | + |
| 111 | +nope. |
| 112 | + |
| 113 | +**Complexity:** |
| 114 | + |
| 115 | +* Time complexity : O(n). |
| 116 | +* Space complexity : O(n). |
0 commit comments