|
| 1 | +# 514. Freedom Trail |
| 2 | + |
| 3 | +- Difficulty: Hard. |
| 4 | +- Related Topics: String, Dynamic Programming, Depth-First Search, Breadth-First Search. |
| 5 | +- Similar Questions: . |
| 6 | + |
| 7 | +## Problem |
| 8 | + |
| 9 | +In the video game Fallout 4, the quest **"Road to Freedom"** requires players to reach a metal dial called the **"Freedom Trail Ring"** and use the dial to spell a specific keyword to open the door. |
| 10 | + |
| 11 | +Given a string `ring` that represents the code engraved on the outer ring and another string `key` that represents the keyword that needs to be spelled, return **the minimum number of steps to spell all the characters in the keyword**. |
| 12 | + |
| 13 | +Initially, the first character of the ring is aligned at the `"12:00"` direction. You should spell all the characters in `key` one by one by rotating `ring` clockwise or anticlockwise to make each character of the string key aligned at the `"12:00"` direction and then by pressing the center button. |
| 14 | + |
| 15 | +At the stage of rotating the ring to spell the key character `key[i]`: |
| 16 | + |
| 17 | + |
| 18 | + |
| 19 | +- You can rotate the ring clockwise or anticlockwise by one place, which counts as **one step**. The final purpose of the rotation is to align one of `ring`'s characters at the `"12:00"` direction, where this character must equal `key[i]`. |
| 20 | + |
| 21 | +- If the character `key[i]` has been aligned at the `"12:00"` direction, press the center button to spell, which also counts as **one step**. After the pressing, you could begin to spell the next character in the key (next stage). Otherwise, you have finished all the spelling. |
| 22 | + |
| 23 | + |
| 24 | + |
| 25 | +Example 1: |
| 26 | + |
| 27 | +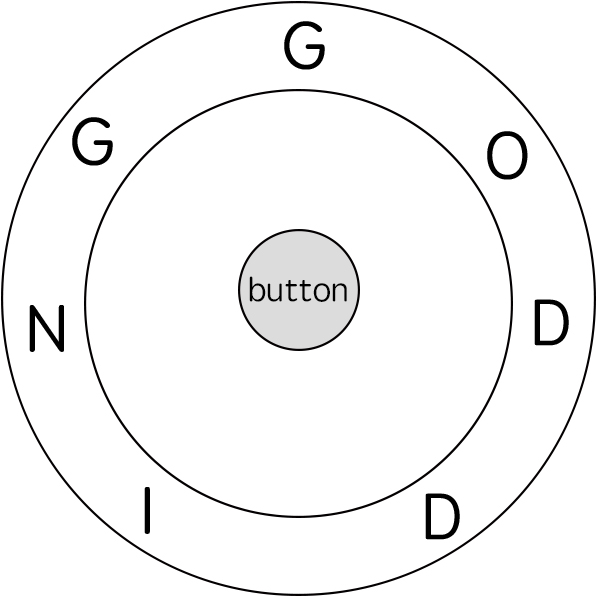 |
| 28 | + |
| 29 | +``` |
| 30 | +Input: ring = "godding", key = "gd" |
| 31 | +Output: 4 |
| 32 | +Explanation: |
| 33 | +For the first key character 'g', since it is already in place, we just need 1 step to spell this character. |
| 34 | +For the second key character 'd', we need to rotate the ring "godding" anticlockwise by two steps to make it become "ddinggo". |
| 35 | +Also, we need 1 more step for spelling. |
| 36 | +So the final output is 4. |
| 37 | +``` |
| 38 | + |
| 39 | +Example 2: |
| 40 | + |
| 41 | +``` |
| 42 | +Input: ring = "godding", key = "godding" |
| 43 | +Output: 13 |
| 44 | +``` |
| 45 | + |
| 46 | + |
| 47 | +**Constraints:** |
| 48 | + |
| 49 | + |
| 50 | + |
| 51 | +- `1 <= ring.length, key.length <= 100` |
| 52 | + |
| 53 | +- `ring` and `key` consist of only lower case English letters. |
| 54 | + |
| 55 | +- It is guaranteed that `key` could always be spelled by rotating `ring`. |
| 56 | + |
| 57 | + |
| 58 | + |
| 59 | +## Solution |
| 60 | + |
| 61 | +```javascript |
| 62 | +/** |
| 63 | + * @param {string} ring |
| 64 | + * @param {string} key |
| 65 | + * @return {number} |
| 66 | + */ |
| 67 | +var findRotateSteps = function(ring, key) { |
| 68 | + var next = function(i) { |
| 69 | + return i === ring.length - 1 ? 0 : i + 1; |
| 70 | + }; |
| 71 | + var prev = function(i) { |
| 72 | + return i === 0 ? ring.length - 1 : i - 1; |
| 73 | + }; |
| 74 | + var dp = Array(ring.length).fill(0).map(() => Array(key.length)); |
| 75 | + var dfs = function(i, j) { |
| 76 | + if (j === key.length) return 0; |
| 77 | + if (dp[i][j] !== undefined) return dp[i][j]; |
| 78 | + if (ring[i] === key[j]) { |
| 79 | + dp[i][j] = 1 + dfs(i, j + 1); |
| 80 | + return dp[i][j]; |
| 81 | + } |
| 82 | + var nextI = next(i); |
| 83 | + while (ring[nextI] !== key[j]) nextI = next(nextI); |
| 84 | + var prevI = prev(i); |
| 85 | + while (ring[prevI] !== key[j]) prevI = prev(prevI); |
| 86 | + dp[i][j] = Math.min( |
| 87 | + ((nextI - i + ring.length) % ring.length) + dfs(nextI, j), |
| 88 | + ((i - prevI + ring.length) % ring.length) + dfs(prevI, j), |
| 89 | + ); |
| 90 | + return dp[i][j]; |
| 91 | + }; |
| 92 | + return dfs(0, 0); |
| 93 | +}; |
| 94 | +``` |
| 95 | + |
| 96 | +**Explain:** |
| 97 | + |
| 98 | +nope. |
| 99 | + |
| 100 | +**Complexity:** |
| 101 | + |
| 102 | +* Time complexity : O(n * n * m). |
| 103 | +* Space complexity : O(n * m). |
0 commit comments