|
| 1 | +# 543. Diameter of Binary Tree |
| 2 | + |
| 3 | +- Difficulty: Easy. |
| 4 | +- Related Topics: Tree, Depth-First Search, Binary Tree. |
| 5 | +- Similar Questions: Diameter of N-Ary Tree, Longest Path With Different Adjacent Characters. |
| 6 | + |
| 7 | +## Problem |
| 8 | + |
| 9 | +Given the `root` of a binary tree, return **the length of the **diameter** of the tree**. |
| 10 | + |
| 11 | +The **diameter** of a binary tree is the **length** of the longest path between any two nodes in a tree. This path may or may not pass through the `root`. |
| 12 | + |
| 13 | +The **length** of a path between two nodes is represented by the number of edges between them. |
| 14 | + |
| 15 | + |
| 16 | +Example 1: |
| 17 | + |
| 18 | +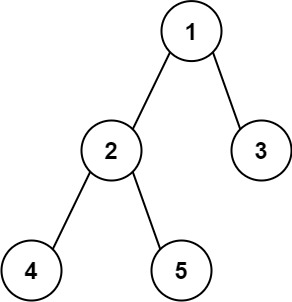 |
| 19 | + |
| 20 | +``` |
| 21 | +Input: root = [1,2,3,4,5] |
| 22 | +Output: 3 |
| 23 | +Explanation: 3 is the length of the path [4,2,1,3] or [5,2,1,3]. |
| 24 | +``` |
| 25 | + |
| 26 | +Example 2: |
| 27 | + |
| 28 | +``` |
| 29 | +Input: root = [1,2] |
| 30 | +Output: 1 |
| 31 | +``` |
| 32 | + |
| 33 | + |
| 34 | +**Constraints:** |
| 35 | + |
| 36 | + |
| 37 | + |
| 38 | +- The number of nodes in the tree is in the range `[1, 104]`. |
| 39 | + |
| 40 | +- `-100 <= Node.val <= 100` |
| 41 | + |
| 42 | + |
| 43 | + |
| 44 | +## Solution |
| 45 | + |
| 46 | +```javascript |
| 47 | +/** |
| 48 | + * Definition for a binary tree node. |
| 49 | + * function TreeNode(val, left, right) { |
| 50 | + * this.val = (val===undefined ? 0 : val) |
| 51 | + * this.left = (left===undefined ? null : left) |
| 52 | + * this.right = (right===undefined ? null : right) |
| 53 | + * } |
| 54 | + */ |
| 55 | +/** |
| 56 | + * @param {TreeNode} root |
| 57 | + * @return {number} |
| 58 | + */ |
| 59 | +var diameterOfBinaryTree = function(root) { |
| 60 | + return dfs(root)[0]; |
| 61 | +}; |
| 62 | + |
| 63 | +var dfs = function(node) { |
| 64 | + if (!node) return [0, 0]; |
| 65 | + var left = dfs(node.left); |
| 66 | + var right = dfs(node.right); |
| 67 | + var child = Math.max( |
| 68 | + node.left ? left[1] + 1 : 0, |
| 69 | + node.right ? right[1] + 1 : 0, |
| 70 | + ); |
| 71 | + var branch = (node.left ? left[1] + 1 : 0) + |
| 72 | + (node.right ? right[1] + 1 : 0); |
| 73 | + return [ |
| 74 | + Math.max( |
| 75 | + left[0], |
| 76 | + right[0], |
| 77 | + branch, |
| 78 | + ), |
| 79 | + child, |
| 80 | + ]; |
| 81 | +}; |
| 82 | +``` |
| 83 | + |
| 84 | +**Explain:** |
| 85 | + |
| 86 | +nope. |
| 87 | + |
| 88 | +**Complexity:** |
| 89 | + |
| 90 | +* Time complexity : O(n). |
| 91 | +* Space complexity : O(1). |
0 commit comments